Phar is an extension library for PHP. It can package the entire PHP project into an executable file, so that the project can be easily run in different environments without the need to install PHP and related dependent libraries. At the same time, this executable file can also be encrypted to protect the security of the project.
This article will introduce how to use Phar to package PHP projects.
- Install Phar extension library
To use the Phar extension library, you first need to install the Phar extension in PHP. In a Linux environment, you can use the following command to install:
sudo apt-get install php-phar
In a Windows environment, you can enable the Phar extension in the php.ini file and remove the semicolon before the following statement:
;extension=php_phar.dll
- Packaging project
Phar provides a packaging tool phar, which can package the entire project into a .phar file.
Enter the following command in the terminal:
php phar -c gz -f myapp.phar /path/to/myapp
Among them, -c gz means using the gzip compression algorithm, -f myapp.phar means the output file name is myapp.phar, /path/to /myapp represents the project path to be packaged.
After the packaging is completed, you can execute the following command in the terminal to run the .phar file:
php myapp.phar
- Phar file encryption
You can use Phar’s API Encrypt the .phar file to protect the project's code from being decompiled or tampered with.
For example, you can use the Phar::createDefaultStub() method to generate a default execution script, and use openssl to encrypt this script:
<?php $phar = new Phar('myapp.phar'); $phar->startBuffering(); $phar->buildFromDirectory('/path/to/myapp'); $phar->setStub($phar->createDefaultStub('index.php')); $key = 'mysecretkey'; $iv = substr(md5($key), 0, 16); $phar->setSignatureAlgorithm(Phar::SHA256); $phar->stopBuffering(); $phar->setMetadata(['encryption_key' => $key]); $content = file_get_contents('phar://myapp.phar/index.php'); $encrypted = openssl_encrypt($content, 'AES-256-CBC', $key, null, $iv); $phar->setStub("<?php __HALT_COMPILER(); ?>$encrypted");
In the above code, $key is encrypted Key, $iv is the initial vector. After encrypting the execution script, insert the encrypted result into the header of the .phar file. At this time, executing the .phar file requires entering the key to run successfully.
- Unpack Phar file
If you need to modify the Phar file, you can use the PharData class to unpack it.
For example, you can use the following code to unpack the .phar file to the specified directory:
<?php $phar = new Phar('myapp.phar'); $phar->extractTo('/path/to/extract');
After the unpacking is completed, you can modify and debug the project.
Summary
Phar is a very practical PHP extension library that can easily package the entire PHP project into an executable file and encrypt it. In actual project development, Phar can be used to simplify the deployment process and improve project operation efficiency and security.
The above is the detailed content of How to use Phar to package projects in PHP. For more information, please follow other related articles on the PHP Chinese website!
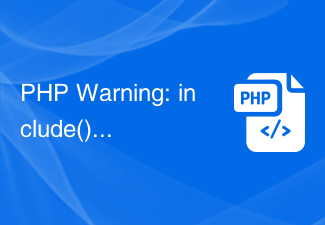
PHP是一种流行的开发语言,常用于构建动态网站和应用程序。虽然PHP在网站和应用程序的开发过程中具有很多优点,但也可能会遇到一些常见的错误。其中之一就是“PHPWarning:include():Failedopening”的错误提示。这个错误提示意味着PHP无法找到或读取被引用的文件。那么如何解决这个问题呢?本文将提供一些有效的解决方法。检查文件路径
![使用PHP$_SERVER['HTTP_REFERER']获取页面来源地址](https://img.php.cn/upload/article/000/887/227/169236391218703.jpg)
在网络上浏览网页时,我们经常会看到一些跳转链接,当我们点击这些链接时,会跳转到另一个网页或网站。那么,如何知道我们是从哪个网站或网页跳转过来的呢?这时候,我们就需要用到一个重要的PHP变量——$_SERVER['HTTP_REFERER']。$_SERVER['HTTP_REFERER']变量是一个用来获取HTTP请求来源地址的变量。也就是说,当一个网页跳转
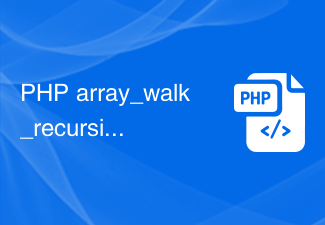
在PHP开发中,数组(array)是一个常见且必备的数据类型。而且,在PHP中,数组的数据结构非常灵活,可以包含不同类型的元素,如字符串、数字、布尔等,甚至可以嵌套其他数组。当需要在数组中对每个元素进行某些操作时,PHP提供的array_walk()函数是一个非常有效的方法。但是,如果数组嵌套了其他数组,则需要使用array_walk_recursive()
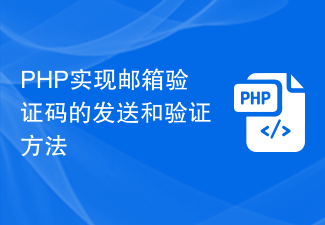
PHP实现邮箱验证码的发送和验证方法随着互联网的发展,邮箱验证码逐渐成为验证用户身份的一种重要方式。在开发网站或应用程序时,我们通常会使用邮箱验证码来实现用户注册、密码找回等功能。本文将介绍如何使用PHP来实现邮箱验证码的发送和验证,并提供具体的代码示例。发送邮箱验证码首先,我们需要使用PHP发送验证码邮件至用户的注册邮箱。下面是一个简单的示例代码,使用PH
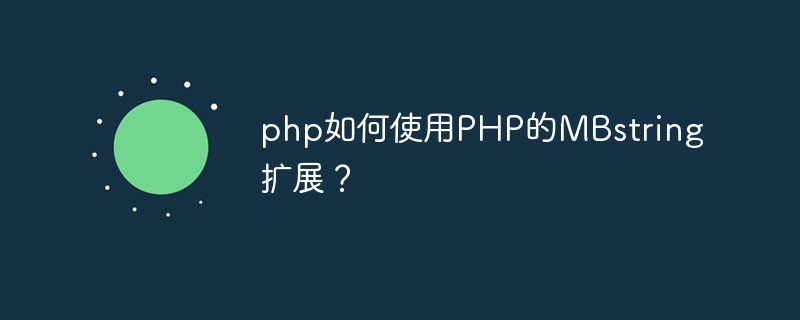
PHP是一种流行的编程语言,它被广泛应用于Web开发、服务器端脚本编程、命令行脚本编写等领域。其中,字符串操作是PHP编程中比较常用的一个功能。为了操作多字节字符,PHP提供了一个名为MBstring的扩展,本文将介绍如何使用PHP的MBstring扩展。一、MBstring扩展的介绍MBstring扩展是一个用于操作多字节字符的PHP扩展,其主要作用是提供
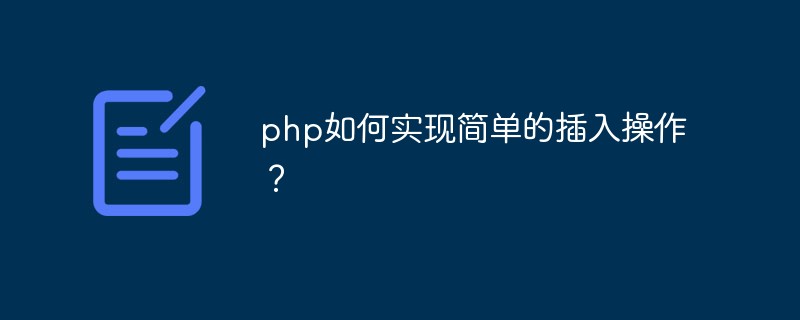
作为一门广受欢迎的编程语言,在Web开发中,PHP被广泛应用的其中一个应用就是实现数据库操作。而插入操作是数据库操作中最基本也是最常见的操作之一。在PHP中,要实现插入操作并不难,只需要按照以下几个步骤实现即可。一、准备数据库首先,我们需要在PHP中连接到数据库,并确保我们的PHP代码能够顺利地通过数据库进行读写操作。连接到数据库需要使用
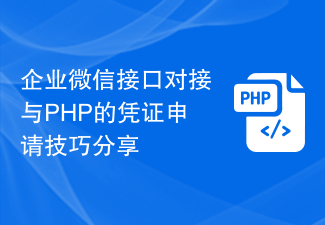
企业微信接口对接与PHP的凭证申请技巧分享随着移动互联网的快速发展,企业对于即时沟通和协作的需求越来越迫切。企业微信作为一款专为企业打造的通讯工具,成为越来越多企业选择的首选。为了满足企业的个性化需求,企业微信提供了丰富的应用接口供开发者进行定制开发。本文将分享企业微信接口对接的相关知识,并重点介绍如何使用PHP语言申请企业微信的凭证。企业微信接口对
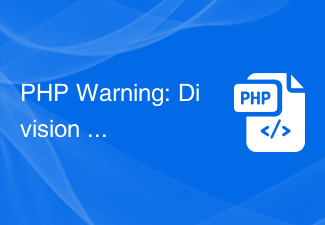
在进行PHP开发过程中,经常会遇到各种错误和异常。其中,PHPWarning:Divisionbyzeroin是一种经常出现的错误,它提示我们在某个地方进行了除零操作。这个错误消息看起来比较恐怖,但实际上它很好处理,下面就为大家介绍几种解决方法。检查代码首先,我们需要检查自己的代码。PHPWarning:Divisionbyzero


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
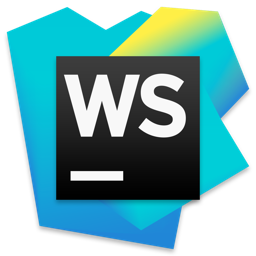
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
