With the continuous development of Internet technology, the performance issues of Web applications have attracted more and more attention from developers. Especially when the number of concurrent requests increases, the response speed and performance of applications tend to become slower. Slow and may even cause the system to crash. To solve this problem, developers began to take various optimization measures, among which the use of queues became a more effective solution. This article will introduce how to use queues for performance optimization in PHP development.
1. What is a queue
A queue is a data structure that can be used to arrange elements according to certain rules and maintain this order when elements are added and deleted. In computer science, queues are often used to handle large amounts of messages to avoid instantaneous spikes that overwhelm the system. The queue has the characteristics of first-in-first-out (FIFO), that is, the elements added to the queue first are processed first.
2. Why use queues
In web applications, there are many tasks that consume a lot of time and CPU resources, such as sending emails, generating PDFs, etc. If these tasks are performed directly in response to user requests, it is easy to cause the application to respond slowly, or even cause the system to crash. The use of queues can allocate these tasks to asynchronous processes for execution, thereby reducing the workload of the application and improving the response speed and performance of the application.
3. How to use queues
Queue can be implemented in many ways in PHP, such as Redis, Beanstalkd, Message Queue, etc. Before introducing the specific implementation method, we need to understand two important concepts of queues: producers and consumers.
Producer: A program or module that puts tasks that need to be executed into the queue. In PHP development, producers can be implemented by writing task data to the message queue.
Consumer: Get tasks from the queue and execute them, and finally return the execution results to the producer. In PHP development, consumers can obtain tasks by listening to the message queue, and then execute the task data after taking it out.
In actual applications, there are usually multiple consumers listening to the queue at the same time. If there are multiple tasks waiting to be executed in the queue, the consumer will process them in sequence according to rules, such as queuing, parallelism, load balancing, etc.
The following uses Redis and Beanstalkd as examples to introduce how to use queues for performance optimization in PHP.
Redis
Redis is a popular in-memory database that supports a variety of data structures, such as strings, hashes, lists, sets, ordered sets, etc. Simple queue functions can be implemented by writing task data to a Redis list.
Suppose there is a task to send emails, we need to add this task to the queue. You can use the following code:
$redis = new Redis(); $redis->connect('localhost', 6379); $taskData = [ 'to' => 'example@test.com', 'subject' => 'Test Email', 'content' => 'This is a test email.', ]; $redis->rpush('email_queue', json_encode($taskData));
The above code uses Redis's rpush command to add task data to a list named email_queue. Next, we need to write a consumer script to get tasks from the queue and execute them. The following is a simple email sending consumer example:
$redis = new Redis(); $redis->connect('localhost', 6379); while (true) { $taskData = $redis->blpop('email_queue', 0)[1]; $task = json_decode($taskData, true); // 发送邮件 $result = sendEmail($task['to'], $task['subject'], $task['content']); // 处理结果 if ($result) { // 发送成功,记录日志等 } else { // 发送失败,重试或记录日志等 } }
The above code uses the blpop command of Redis to obtain tasks from email_queue. This command blocks the consumer process until there are tasks in the queue to process. After obtaining the task, we parse the task data and use the sendEmail function to send the email. Finally, we perform logging and other subsequent processing based on the sending results.
Beanstalkd
Beanstalkd is a lightweight queue service that is very simple to use. By adding task data to the queue, it is made available to the consumer process for processing.
Suppose there is a task to generate PDF, we need to add this task to the queue. You can use the following code:
$pheanstalk = new Pheanstalk('localhost'); $taskData = [ 'template' => 'invoice', 'data' => [ 'invoice_id' => 1234, 'amount' => 100, // ... ], ]; $pheanstalk->putInTube('pdf_generation', json_encode($taskData));
The above code uses the Pheanstalk library to add task data to a tube named pdf_generation. A tube is similar to a list in Redis. In Beanstalkd, multiple tubes can be configured so that different tasks use different tubes to communicate.
Next, we need to write a consumer script to get tasks from the queue and execute them. The following is a simple PDF generation consumer example:
$pheanstalk = new Pheanstalk('localhost'); while (true) { $job = $pheanstalk->watchOnly('pdf_generation')->reserve(); $taskData = $job->getData(); $task = json_decode($taskData, true); // 生成PDF $result = generatePDF($task['template'], $task['data']); // 处理结果 if ($result) { // 生成成功,记录日志等 } else { // 生成失败,重试或记录日志等 } $pheanstalk->delete($job); }
The above code uses the reserve and delete methods of the Pheanstalk library to obtain tasks from pdf_generation tube. The reserve method blocks the consumer process until there are tasks in the queue to process. After obtaining the task, we parse the task data and use the generatePDF function to generate a PDF file. Finally, we perform subsequent processing such as logging based on the generated results. Finally, remember to use the delete method to mark the task as completed.
4. Notes
When using queues for performance optimization, you need to pay attention to the following points:
- Queue implementation methods are different, and performance and stability are also different. There will be differences. An appropriate queue implementation method needs to be selected based on the actual application scenario.
- When task execution fails, subsequent processing is required, such as retrying or logging.
- When there are too many tasks in the queue or the task processing time is too long, load balancing should be considered to avoid a certain consumer being over-occupied.
- Due to the asynchronous nature of the queue, the task execution results cannot be obtained immediately. If you need to obtain the task execution results immediately, you can use other methods to achieve it.
5. Conclusion
Using queues is an effective way to optimize web application performance. In PHP development, by writing task data to the message queue, resource-intensive or time-consuming tasks can be assigned to asynchronous processes for execution, thereby alleviating the Offload applications and improve system responsiveness and performance. In actual applications, it is necessary to choose an appropriate queue implementation method based on the actual situation, and pay attention to issues such as task execution failure and load balancing.
The above is the detailed content of How to use queues to optimize performance in PHP development. For more information, please follow other related articles on the PHP Chinese website!
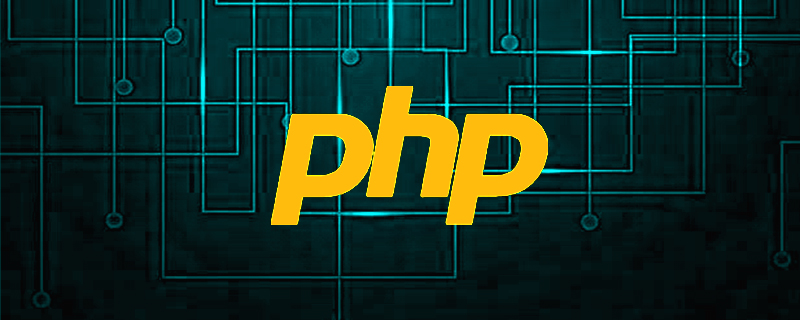
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
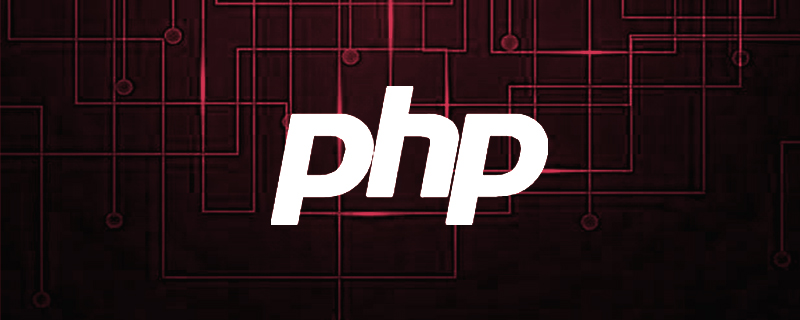
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
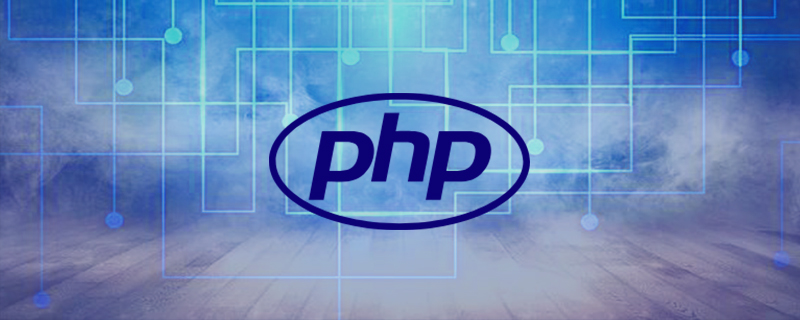
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
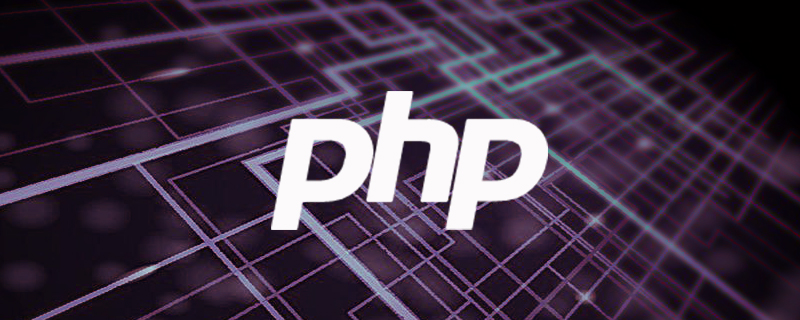
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
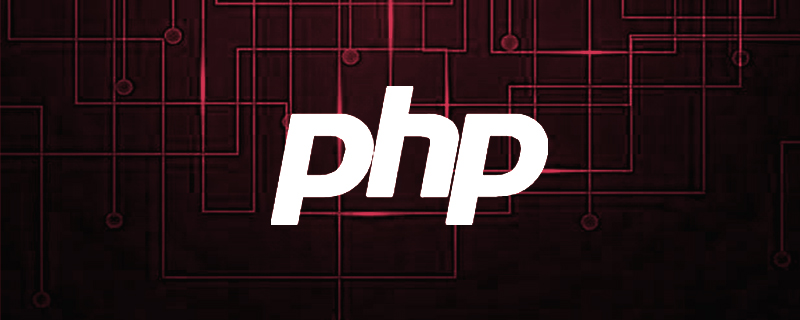
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
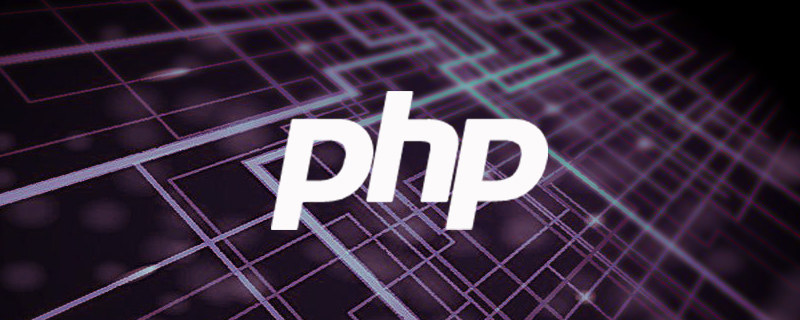
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
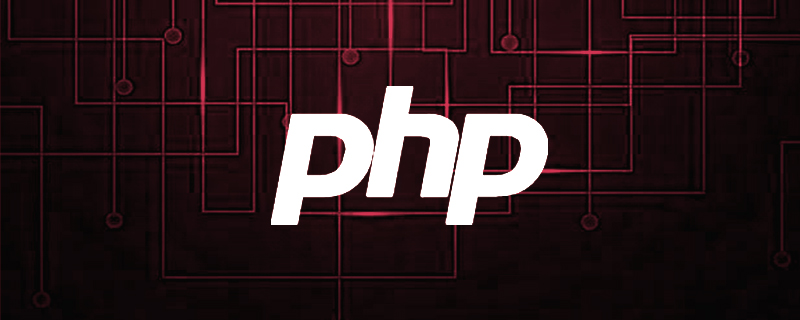
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
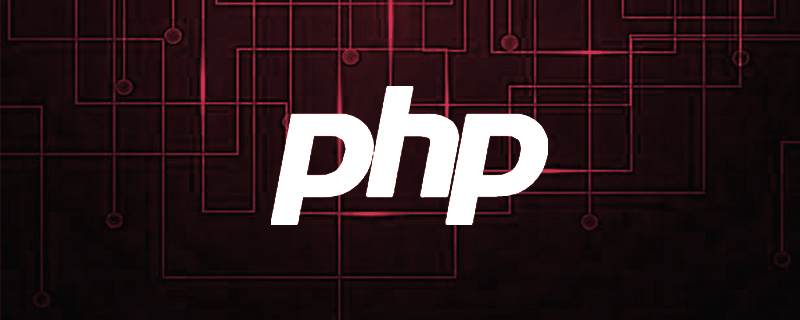
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
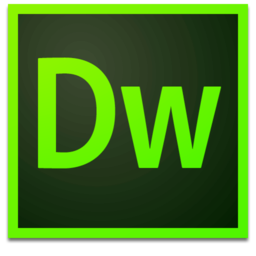
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
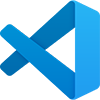
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
