Image processing is very common in modern Internet applications, ranging from website design to graphical data visualization and computer vision applications that require image processing. PHP provides an image processing library called GD, which supports most common image format processing and provides conventional operations and conversion methods, such as rotation, scaling, cropping, filters, etc. Today we will introduce how to use the GD image processing library for image processing in PHP applications.
1. Install the GD extension
Before you start using the GD library, you need to ensure that the extension has been enabled in the php.ini configuration file. If you are using a Linux server, you can check whether the GD extension has been installed by running the following command:
sudo apt-get install php-gd
For other operating systems and PHP versions, please Read the installation documentation carefully.
2. Loading images
In PHP, it is very simple to load images using the GD library:
$filename = "image.jpg";
$image = imagecreatefromjpeg ($filename);
In this example, we first specify the path to an image file, and then load the image using the imagecreatefromjpeg() function. You can also load images in PNG and GIF formats using the imagecreatefrompng() or imagecreatefromgif() functions.
3. Resizing
For most image processing applications, one of the common tasks is to resize the image. Using the GD library, images can be scaled by calling the imagecopyresampled() function, for example:
$desiredwidth = 800;
$desiredheight = 600;
$origwidth = imagesx($image);
$origheight = imagesy($image);
$ratio = $origwidth / $origheight;
if ($desiredwidth / $desiredheight > $ratio) {
$newheight = $desiredwidth / $ratio; $newwidth = $desiredwidth;
} else {
$newwidth = $desiredheight * $ratio; $newheight = $desiredheight;
}
$newimage = imagecreatetruecolor($desiredwidth, $desiredheight);
imagecopyresampled($newimage, $image, 0, 0, 0, 0, $ newwidth, $newheight, $origwidth, $origheight);
$image = $newimage;
In this code, we first define the width and height we want to adjust. Then, we get the width and height of the original image through the imagesx() and imagesy() functions. We calculate the ratio of the original height and width to ensure that the scaled image maintains the same aspect ratio. Next, create a new canvas and use the imagecopyresampled() function to copy the image from the old canvas to the new canvas.
4. Rotate and flip
The GD library also supports rotation and flipping of images, for example:
$image = imagecreatefromjpeg($filename);
$image = imagerotate($image, 45, 0);
In this example, we use the imagerotate() function to rotate the loaded image 45 degrees. If you want to flip horizontally or vertically, you can use the imageflip() function, as follows:
$image = imagecreatefromjpeg($filename);
imageflip($image, IMG_FLIP_VERTICAL);
5. Crop the image
If you need to crop the image, you can use the imagecrop() function. For example:
$image = imagecreatefromjpeg($filename);
$x = 20;
$y = 20;
$width = 200;
$height = 200;
$crop = imagecrop($image, ['x' => $x, 'y' => $y, 'width' => $width, 'height' => $height]);
$image = $crop;
In this example, we crop by defining the horizontal and vertical coordinates, width and height of the crop. The crop() function then returns a new canvas and assigns it to the $image variable.
6. Add filters
The GD library makes images more interesting and vivid by providing some built-in filter effects. For example, the following code can turn an image into grayscale:
$image = imagecreatefromjpeg($filename);
imagefilter($image, IMG_FILTER_GRAYSCALE);
$crop = imagecrop( $image, ['x' => $x, 'y' => $y, 'width' => $width, 'height' => $height]);
$image = $crop ;
Use the following constants to use other built-in filters:
- IMG_FILTER_NEGATE - Negative effect
- IMG_FILTER_EMBOSS - Embossing effect
- IMG_FILTER_EDGEDETECT - Edge detection Effect
- IMG_FILTER_GAUSSIAN_BLUR - Gaussian blur effect
- IMG_FILTER_SELECTIVE_BLUR - Non-linear blur effect
7. Save the image
After completing the image processing, the last step That is to save the processed image to a file or output it to the browser. Use the imagejpeg() function to save the image in JPEG format:
$destination = "new-image.jpg";
imagejpeg($image, $destination);
can be used The imagepng() or imagegif() function saves the image in PNG or GIF format respectively.
Summary
The GD library is a powerful image processing library that can make PHP applications richer and more vivid. In this article, we covered how to load, scale, crop, filter, and save images using the GD library. Using these techniques, you can take the graphical effects of your PHP applications to the next level!
The above is the detailed content of How to use the image processing library GD in PHP. For more information, please follow other related articles on the PHP Chinese website!
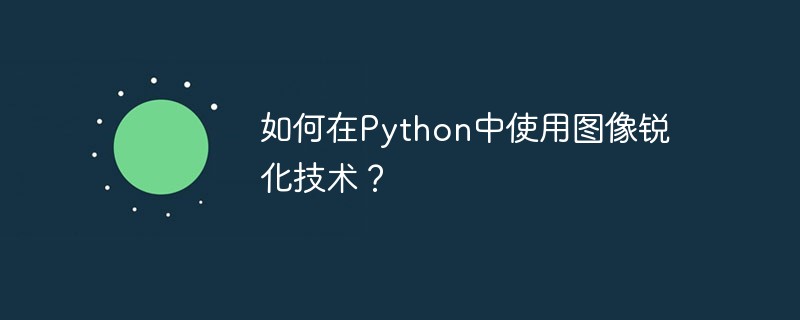
图像锐化是一种常用的图像处理技术,它能够使图片变得更加清晰和细节明显。在Python中,我们可以使用一些常见的图像处理库来实现图像锐化功能。本文将介绍如何使用Python中的Pillow库、OpenCV库和Scikit-Image库进行图像锐化。使用Pillow库进行图像锐化Pillow库是Python中常用的图像处理库,其提供了PIL(PythonIma
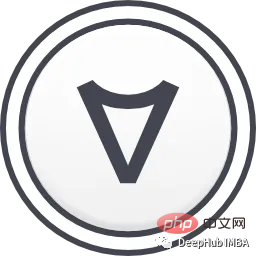
图像处理已经成为我们日常生活中不可或缺的一部分,涉及到社交媒体和医学成像等各个领域。通过数码相机或卫星照片和医学扫描等其他来源获得的图像可能需要预处理以消除或增强噪声。频域滤波是一种可行的解决方案,它可以在增强图像锐化的同时消除噪声。快速傅里叶变换(FFT)是一种将图像从空间域变换到频率域的数学技术,是图像处理中进行频率变换的关键工具。通过利用图像的频域表示,我们可以根据图像的频率内容有效地分析图像,从而简化滤波程序的应用以消除噪声。本文将讨论图像从FFT到逆FFT的频率变换所涉及的各个阶段,并
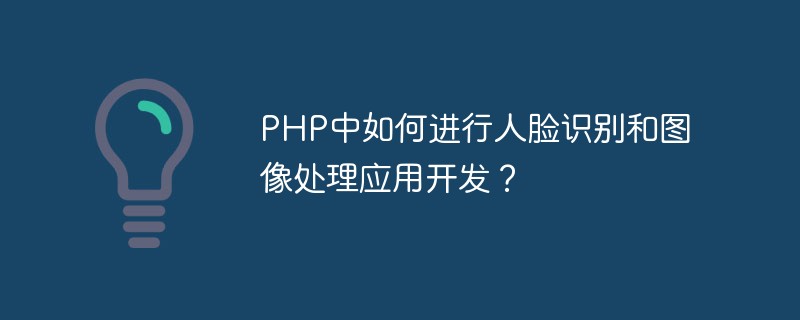
在当今数字化时代,图像处理技术已成为了一种必备的技能,而人脸识别技术则被广泛应用于各行各业。其中,PHP作为一门广泛应用于web开发的脚本语言,其在人脸识别和图像处理应用开发方面的技术初步成熟,而其开发工具和框架也在不断发展。本文将给大家介绍PHP中如何进行图像处理和人脸识别技术的应用开发。I.图像处理应用开发GD库GD库是PHP中非常重要的一个图像处理工
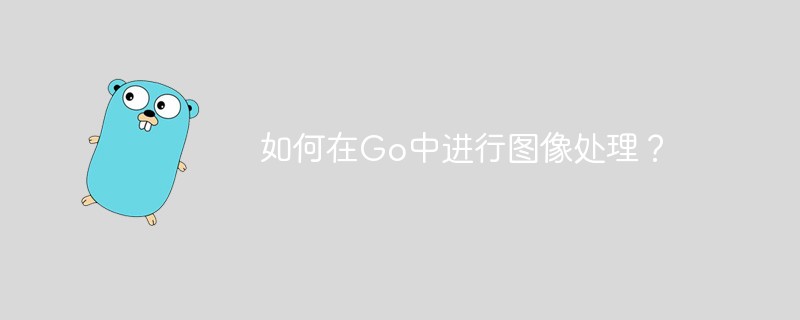
作为一门高效的编程语言,Go在图像处理领域也有着不错的表现。虽然Go本身的标准库中没有提供专门的图像处理相关的API,但是有一些优秀的第三方库可以供我们使用,比如GoCV、ImageMagick和GraphicsMagick等。本文将重点介绍使用GoCV进行图像处理的方法。GoCV是一个高度依赖于OpenCV的Go语言绑定库,其
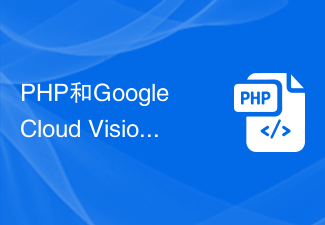
PHP是一种广泛使用的开放源代码的服务器端编程语言。它在网站开发二维图形处理和图片渲染技术方面广受欢迎。要实现有关图像和视觉数据的处理,我们可以使用GoogleCloudVisionAPI以及PHP。GoogleCloudVisionAPI是一个灵活的计算机视觉API,它可以帮助开发者更轻松地构建各种机器视觉应用程序。它支持图像标记、面部识别、文
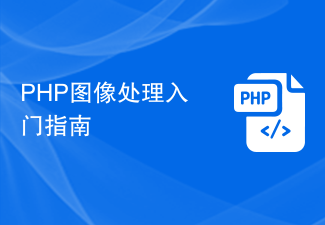
PHP是一种非常流行的服务器端编程语言,它被广泛用于Web开发。在Web开发中,图像处理是一个非常常见的需求,而在PHP中实现图像处理也是很简单的。本文将简要介绍PHP图像处理的入门指南。一、环境要求要使用PHP图像处理,首先需要PHPGD库的支持。该库提供了将图像写入文件或输出到浏览器的功能、裁剪和缩放图像、添加文字、以及使图像变为灰度或反转等功能。因此
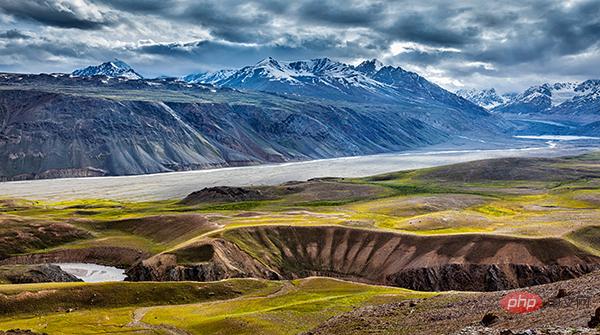
当今世界充满了各种数据,而图像是其中高的重要组成部分。然而,若想其有所应用,我们需要对这些图像进行处理。图像处理是分析和操纵数字图像的过程,旨在提高其质量或从中提取一些信息,然后将其用于某些方面。图像处理中的常见任务包括显示图像,基本操作(如裁剪、翻转、旋转等),图像分割,分类和特征提取,图像恢复和图像识别等。Python之成为图像处理任务的最佳选择,是因为这一科学编程语言日益普及,并且其自身免费提供许多最先进的图像处理工具。让我们看一下用于图像处理任务的一些常用Python库。1、scikit
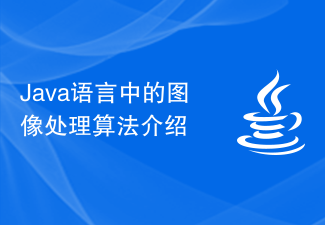
Java语言中的图像处理算法介绍随着数字化时代的到来,图像处理已经成为了计算机科学中的一个重要分支。在计算机中,图像是以数字形式存储的,而图像处理则是通过对这些数字进行一系列的算法运算,改变图像的质量和外观。Java语言作为一种跨平台的编程语言,其丰富的图像处理库和强大的算法支持,使得它成为了很多开发者的首选。本文将介绍Java语言中常用的图像处理算法,以及


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
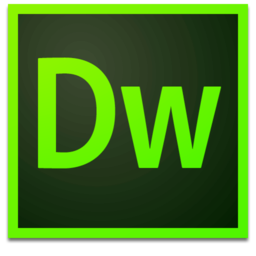
Dreamweaver Mac version
Visual web development tools
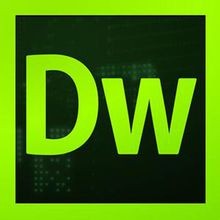
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
