


How to write a recommendation system-based social network application using Java
In modern social network applications, recommendation systems have become an essential function. Whether it is recommending friends to users, recommending topics of interest, recommending related products, or recommending more valuable content, recommendation systems can effectively improve user experience and stickiness.
In this article, we will introduce how to use Java to write a social network application based on a recommendation system. We will combine actual code and detailed steps to help readers quickly understand and implement a basic recommendation system.
1. Data collection and processing
Before implementing any recommendation system, we need to collect and process a large amount of data. In social network applications, user information, posts, comments, likes and other data are all valuable sources of data.
To facilitate demonstration, we can use an open source virtual data generator to generate these data. The specific steps are as follows:
- Download and install a virtual data generator, such as Mockaroo (https://www.mockaroo.com/).
- Define the data sets that need to be generated, including user information, posts, comments, etc.
- Generate data and export to CSV file.
- Use Java code to read the data in the CSV file and store it in the database. We can use popular relational databases such as MySQL and Oracle to store data. Here, we use MySQL 8.0 as the database for data storage.
2. Representation of users and items
In the recommendation system, we need to convert users and items into vector or matrix form in order to calculate their similarity or Make recommendations. In social network applications, we can use the following methods to represent users and items:
- User vector: We can use data such as the topics that users follow, posts they publish, and friends they interact with to represent them. A vector of users. For example, if a user A follows the topics Java, Python, JavaScript, etc., posts "How to learn Java well" and "Getting started with Java", and interacts with users B and C, then we can use the following vector to represent user A:
User A = [1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 0 , 0, 1, 0, 0, 1]
The vector length is 24, and each position represents a topic or post. 1 means that user A has followed the topic or published the post, and 0 means not.
- Item vector: We can use the tags, content, comments and other data of each post to represent the vector of a post. For example, if a post is tagged "Java, programming" and the content is "Four suggestions for learning Java programming" and has 10 comments, then we can use the following vector to represent the post:
Post A = [1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 10 , 0]
The vector length is 24, and each position represents a label or statistical data. 1 means the post contains the tag or content, 0 means it does not.
3. User-based collaborative filtering recommendation
User-based collaborative filtering is a common method in recommendation systems. It recommends items based on the similarity of user interests. Here, we use user-based collaborative filtering to recommend suitable posts for users. The specific steps are as follows:
- Calculate the similarity between users. Here, we use the Pearson correlation coefficient as the similarity metric.
- Select K users with the highest interest similarity to the target user.
- For each user, select N posts that they like but have not been seen by the target user.
- For the selected N posts, calculate the recommendation score of each post and sort them from high to low.
- Select the top M posts with the highest scores as recommended results.
The following is the Java code implementation of the algorithm:
public class CollaborativeFiltering { /** * 计算用户间的皮尔逊相关系数 * @param user1 用户1 * @param user2 用户2 * @param data 数据集 * @return 皮尔逊相关系数 */ public double pearsonCorrelation(Map<Integer, Double> user1, Map<Integer, Double> user2, Map<Integer, Map<Integer, Double>> data) { double sum1 = 0, sum2 = 0, sum1Sq = 0, sum2Sq = 0, pSum = 0; int n = 0; for (int item : user1.keySet()) { if (user2.containsKey(item)) { sum1 += user1.get(item); sum2 += user2.get(item); sum1Sq += Math.pow(user1.get(item), 2); sum2Sq += Math.pow(user2.get(item), 2); pSum += user1.get(item) * user2.get(item); n++; } } if (n == 0) return 0; double num = pSum - (sum1 * sum2 / n); double den = Math.sqrt((sum1Sq - Math.pow(sum1, 2) / n) * (sum2Sq - Math.pow(sum2, 2) / n)); if (den == 0) return 0; return num / den; } /** * 基于用户的协同过滤推荐算法 * @param data 数据集 * @param userId 目标用户 ID * @param K 最相似的 K 个用户 * @param N 推荐的 N 个帖子 * @return 推荐的帖子 ID 列表 */ public List<Integer> userBasedCollaborativeFiltering(Map<Integer, Map<Integer, Double>> data, int userId, int K, int N) { Map<Integer, Double> targetUser = data.get(userId); // 目标用户 List<Map.Entry<Integer, Double>> similarUsers = new ArrayList<>(); // 与目标用户兴趣相似的用户 for (Map.Entry<Integer, Map<Integer, Double>> entry: data.entrySet()) { int id = entry.getKey(); if (id == userId) continue; double sim = pearsonCorrelation(targetUser, entry.getValue(), data); // 计算皮尔逊相关系数 if (sim > 0) similarUsers.add(new AbstractMap.SimpleEntry<>(id, sim)); } Collections.sort(similarUsers, (a, b) -> b.getValue().compareTo(a.getValue())); // 按相似度从高到低排序 List<Integer> itemIds = new ArrayList<>(); for (int i = 0; i < K && i < similarUsers.size(); i++) { Map.Entry<Integer, Double> entry = similarUsers.get(i); int userId2 = entry.getKey(); Map<Integer, Double> user2 = data.get(userId2); for (int itemId: user2.keySet()) { if (!targetUser.containsKey(itemId)) { // 如果目标用户没看过该帖子 itemIds.add(itemId); } } } Map<Integer, Double> scores = new HashMap<>(); for (int itemId: itemIds) { double score = 0; int count = 0; for (Map.Entry<Integer, Double> entry: similarUsers) { int userId2 = entry.getKey(); Map<Integer, Double> user2 = data.get(userId2); if (user2.containsKey(itemId)) { // 如果用户 2 看过该帖子 score += entry.getValue() * user2.get(itemId); count++; if (count == N) break; } } scores.put(itemId, score); } List<Integer> pickedItemIds = new ArrayList<>(); scores.entrySet().stream().sorted((a, b) -> b.getValue().compareTo(a.getValue())) .limit(N).forEach(entry -> pickedItemIds.add(entry.getKey())); // 按得分从高到低排序并选出前N个 return pickedItemIds; } }
4. Content-based recommendation algorithm
The content-based recommendation algorithm is another type of recommendation system. A common method that recommends items based on the similarity of their attributes. Here, we use a content-based recommendation algorithm to recommend suitable posts to users. The specific steps are as follows:
- For the target users, select the topics they follow, the posts they publish, etc.
- Based on these contents, calculate the similarity of each post to the target user's interests.
- Select the top N posts that are most similar to the target user’s interests.
- Sort according to the scores from high to low, and select the top M posts with the highest scores as the recommended results.
The following is the Java code implementation of the content-based recommendation algorithm:
public class ContentBasedRecommendation { /** * 计算两个向量的余弦相似度 * @param v1 向量1 * @param v2 向量2 * @return 余弦相似度 */ public double cosineSimilarity(double[] v1, double[] v2) { double dotProduct = 0; double norma = 0; double normb = 0; for (int i = 0; i < v1.length; i++) { dotProduct += v1[i] * v2[i]; norma += Math.pow(v1[i], 2); normb += Math.pow(v2[i], 2); } if (norma == 0 || normb == 0) return 0; return dotProduct / (Math.sqrt(norma) * Math.sqrt(normb)); } /** * 基于内容的推荐算法 * @param data 数据集 * @param userId 目标用户 ID * @param N 推荐的 N 个帖子 * @return 推荐的帖子 ID 列表 */ public List<Integer> contentBasedRecommendation(Map<Integer, Map<Integer, Double>> data, int userId, int N) { Map<Integer, Double> targetUser = data.get(userId); // 目标用户 int[] pickedItems = new int[data.size()]; double[][] itemFeatures = new double[pickedItems.length][24]; // 物品特征矩阵 for (Map.Entry<Integer, Map<Integer, Double>> entry: data.entrySet()) { int itemId = entry.getKey(); Map<Integer, Double> item = entry.getValue(); double[] feature = new double[24]; for (int i = 0; i < feature.length; i++) { if (item.containsKey(i+1)) { feature[i] = item.get(i+1); } else { feature[i] = 0; } } itemFeatures[itemId-1] = feature; // 物品 ID 从 1 开始,需要减一 } for (int itemId: targetUser.keySet()) { pickedItems[itemId-1] = 1; // 物品 ID 从 1 开始,需要减一 } double[] similarities = new double[pickedItems.length]; for (int i = 0; i < similarities.length; i++) { if (pickedItems[i] == 0) { similarities[i] = cosineSimilarity(targetUser.values().stream().mapToDouble(Double::doubleValue).toArray(), itemFeatures[i]); } } List<Integer> itemIds = new ArrayList<>(); while (itemIds.size() < N) { int maxIndex = -1; for (int i = 0; i < similarities.length; i++) { if (pickedItems[i] == 0 && (maxIndex == -1 || similarities[i] > similarities[maxIndex])) { maxIndex = i; } } if (maxIndex == -1 || similarities[maxIndex] < 0) { break; // 找不到更多相似的物品了 } itemIds.add(maxIndex + 1); // 物品 ID 从 1 开始,需要加一 pickedItems[maxIndex] = 1; } Map<Integer, Double> scores = new HashMap<>(); for (int itemId: itemIds) { double[] features = itemFeatures[itemId-1]; // 物品 ID 从 1 开始,需要减一 double score = cosineSimilarity(targetUser.values().stream().mapToDouble(Double::doubleValue).toArray(), features); scores.put(itemId, score); } List<Integer> pickedItemIds = new ArrayList<>(); scores.entrySet().stream().sorted((a, b) -> b.getValue().compareTo(a.getValue())) .limit(N).forEach(entry -> pickedItemIds.add(entry.getKey())); // 按得分从高到低排序并选出前N个 return pickedItemIds; } }
5. Integrate the recommendation algorithm into the application
After completing the above two recommendation algorithms Once implemented, we can integrate them into the application. The specific steps are as follows:
- Load data and store it in the database. We can use ORM frameworks such as Hibernate to simplify the operation of accessing the database.
- Define a RESTful API that accepts HTTP requests and returns responses in JSON format. We can use Spring Framework to build and deploy RESTful APIs.
- Implement user-based collaborative filtering recommendation and content-based recommendation algorithms and integrate them into RESTful API.
The following is the Java code implementation of the application:
@RestController @RequestMapping("/recommendation") public class RecommendationController { private CollaborativeFiltering collaborativeFiltering = new CollaborativeFiltering(); private ContentBasedRecommendation contentBasedRecommendation = new ContentBasedRecommendation(); @Autowired private UserService userService; @GetMapping("/userbased/{userId}") public List<Integer> userBasedRecommendation(@PathVariable Integer userId) { List<User> allUsers = userService.getAllUsers(); Map<Integer, Map<Integer, Double>> data = new HashMap<>(); for (User user: allUsers) { Map<Integer, Double> userVector = new HashMap<>(); List<Topic> followedTopics = user.getFollowedTopics(); for (Topic topic: followedTopics) { userVector.put(topic.getId(), 1.0); } List<Post> posts = user.getPosts(); for (Post post: posts) { userVector.put(post.getId() + 1000, 1.0); } List<Comment> comments = user.getComments(); for (Comment comment: comments) { userVector.put(comment.getId() + 2000, 1.0); } List<Like> likes = user.getLikes(); for (Like like: likes) { userVector.put(like.getId() + 3000, 1.0); } data.put(user.getId(), userVector); } List<Integer> itemIds = collaborativeFiltering.userBasedCollaborativeFiltering(data, userId, 5, 10); return itemIds; } @GetMapping("/contentbased/{userId}") public List<Integer> contentBasedRecommendation(@PathVariable Integer userId) { List<User> allUsers = userService.getAllUsers(); Map<Integer, Map<Integer, Double>> data = new HashMap<>(); for (User user: allUsers) { Map<Integer, Double> userVector = new HashMap<>(); List<Topic> followedTopics = user.getFollowedTopics(); for (Topic topic: followedTopics) { userVector.put(topic.getId(), 1.0); } List<Post> posts = user.getPosts(); for (Post post: posts) { userVector.put(post.getId() + 1000, 1.0); } List<Comment> comments = user.getComments(); for (Comment comment: comments) { userVector.put(comment.getId() + 2000, 1.0); } List<Like> likes = user.getLikes(); for (Like like: likes) { userVector.put(like.getId() + 3000, 1.0); }
The above is the detailed content of How to write a recommendation system-based social network application using Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
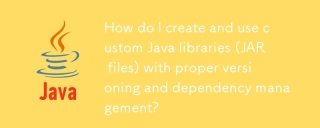
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
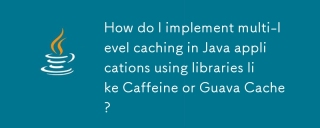
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
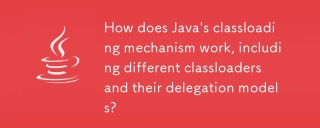
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
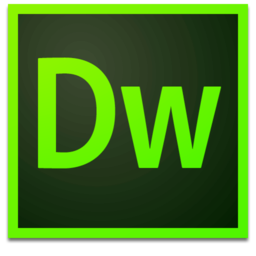
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.