


In Golang, the range statement is a convenient way to traverse data structures such as arrays, slices, strings, and maps. However, when we use the range statement, we sometimes encounter a common error: "cannot use x (type y) as type z in range...". This article aims to introduce the cause of this error and how to solve it.
- Cause of the error
The reason for this error is that when using the range statement, the type of the variable being traversed is inconsistent with the type expected by the range. Specifically, this error usually occurs in the following two situations:
Case 1: The variable being traversed implements an illegal range function
For example, we define a variable named myVar A variable of type string, and then when using range, because this string does not implement the range function, the above error will occur.
var myVar string = "Hello, World!" for index, value := range myVar { fmt.Println(index, string(value)) }
Case 2: The type of the variable being traversed is inconsistent with the type expected by range
For example, we define a variable named myVar with type []int, and use range , expects to access a value of type int in each loop iteration.
var myVar []int = []int{1, 2, 3, 4, 5} for _, value := range myVar { fmt.Println(string(value)) // 报错:cannot use value (type int) as type string in argument to string }
For another example, if we define a variable named myVar of type interface{}, and when using range, we expect to access a value of type string in each loop iteration, then it will also The above error occurs.
var myVar interface{} = []string{"Hello", "World"} for _, value := range myVar { fmt.Println(value.(int)) // 报错:cannot use value (type string) as type int in argument to .(int) }
- Solution
The method to solve this problem is mainly to distinguish based on the above two situations. Specifically, the following steps can be taken to solve this problem:
Step 1: Check whether the traversed variable implements the range function
If it is case 1, we need to check if it is traversed Whether the variable implements the range function. If it is not implemented, we need to transform it to comply with the requirements of the range statement.
For example, in the above example, we can change the type of myVar to []rune, so that we can use the range statement to traverse the string.
var myVar []rune = []rune("Hello, World!") for index, value := range myVar { fmt.Println(index, string(value)) }
Step 2: Convert the traversed variables to the correct type
If it is case 2, we need to convert the traversed variables to the correct type to comply with the range expected type Require.
For example, in the above example, we need to replace int with string in fmt.Println(value.(int)) so that each element can be accessed correctly.
var myVar interface{} = []string{"Hello", "World"} for _, value := range myVar.([]string) { fmt.Println(value) }
Step 3: Use assertions to ensure the correctness of variable types
If we do not know the specific type of the variable being traversed when using the range statement, we can use assertions to ensure the variable type correctness. For example, in the following example, we use the assertion operator on the variables being iterated over.
func printValues(values interface{}) { switch v := values.(type) { case []string: for _, value := range v { fmt.Println(value) } case []int: for _, value := range v { fmt.Println(value) } } }
In short, the reason for this error is that when we use the range statement, the type of the variable being traversed is inconsistent with the type expected by the range. We can solve this problem by checking whether the iterated variable implements the range function, converting the iterated variable to the correct type, and using assertions to ensure the correctness of the variable type.
The above is the detailed content of golang error: 'cannot use x (type y) as type z in range...' How to solve it?. For more information, please follow other related articles on the PHP Chinese website!
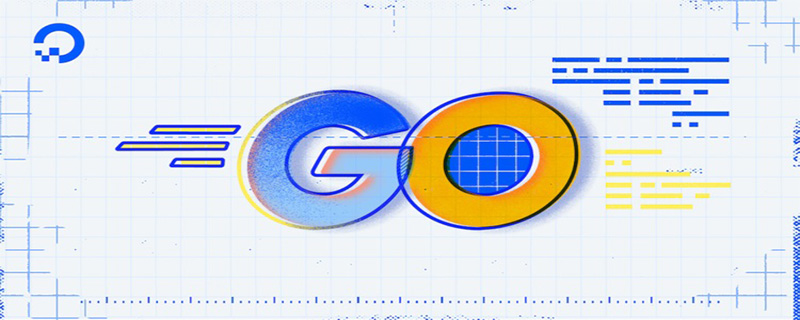
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
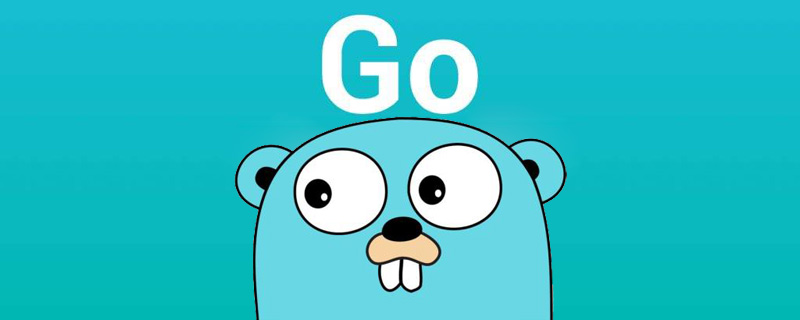
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
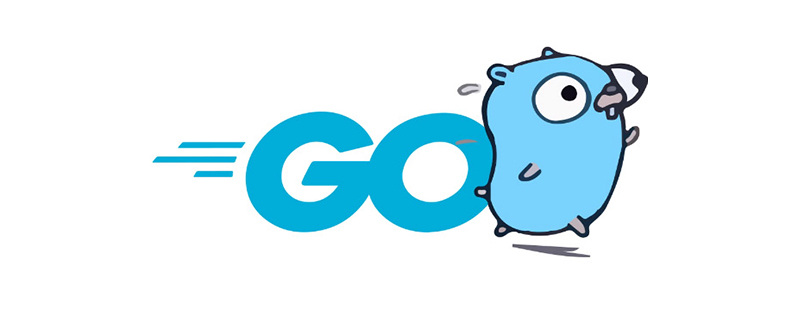
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
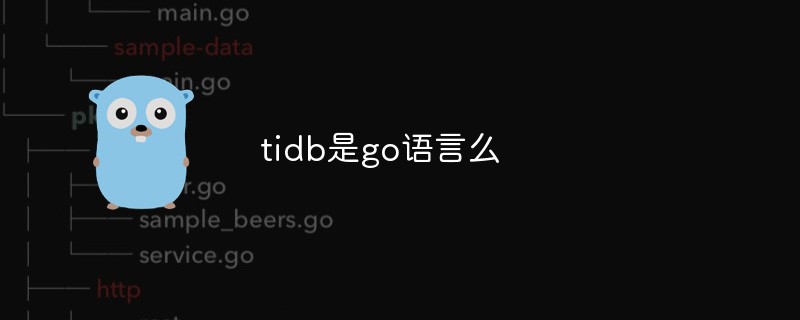
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
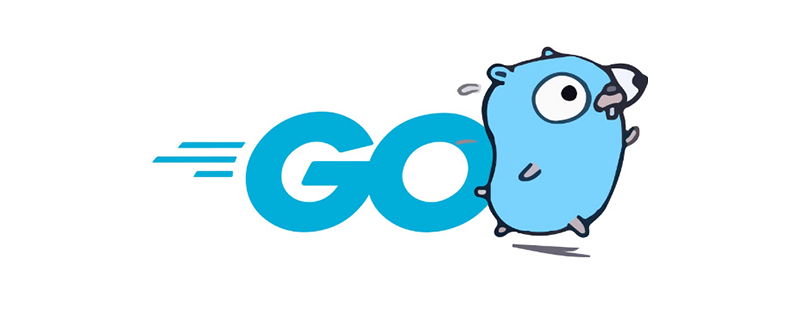
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
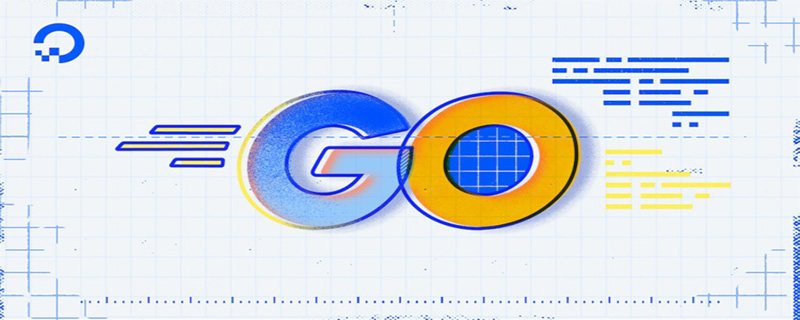
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
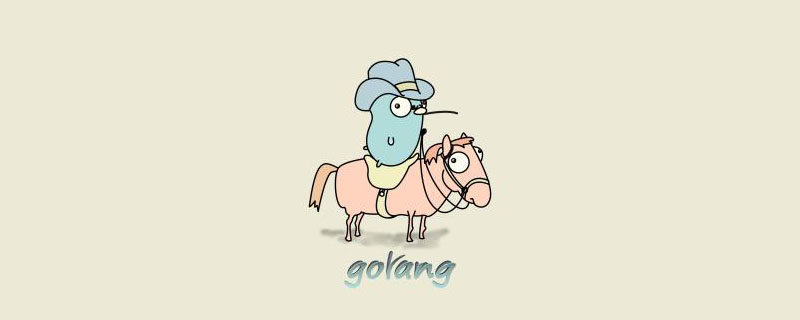
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
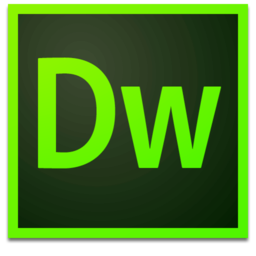
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),