Vue is a popular Javascript framework that has become one of the preferred frameworks for developing web applications. One of the reasons is that Vue developers can easily use CSS animations along with many other powerful animation libraries to help achieve amazing user experiences. Implementing rainbow chart CSS animation in Vue may seem difficult, but in this article, we will show you that it can be achieved easily. Here are some steps and methods.
Step 1: Install Vue CLI
To start using Vue to implement rainbow animation, you first need to install Vue CLI. Vue CLI is a command line interface tool that can be used to quickly create new Vue projects. You can install the Vue CLI by typing the following command in the terminal:
npm install -g vue-cli
Step 2: Create a new Vue project
After installing the Vue CLI, you can use the following command to create a new Vue project:
vue init webpack my-project
This command will generate a new Vue project using the Webpack template and generate a folder named "my-project".
Step 3: Install required packages
Continue using the terminal and enter the project folder (cd my-project
). Install the required npm packages using the following command:
npm install vue-lottie@0.2.6 animate.css@3.7.2
-
vue-lottie
: can help us create and manage Lottie animations -
animate.css
: We will use its animation library to add CSS animation effects
Step 4: Add Lottie JSON file
Lottie is a library for rendering Adobe After Effects animations . Using Lottie, developers can export After Effects animations to JSON files for use in web applications. In this example, we will use a Lottie JSON file to animate a rainbow chart. You can download various Lottie animations for free from [LottieFiles](https://lottiefiles.com/).
Save the downloaded Lottie JSON file in the src/assets directory.
Step 5: Create Vue component
Create a new Vue component named "Rainbow.vue" in the src/components directory. Add the following code to the Rainbow.vue file:
<template> <div class="rainbow-container"> <lottie-animation :animation-data="lottieAnimation" /> </div> </template> <script> import LottieAnimation from 'vue-lottie' import rainbowAnimation from '@/assets/rainbow.json' import 'animate.css/animate.css' export default { name: 'Rainbow', components: { LottieAnimation }, data() { return { lottieAnimation: null } }, mounted() { this.lottieAnimation = rainbowAnimation } } </script> <style lang="scss"> .rainbow-container { width: 100%; height: 50vh; display: flex; justify-content: center; align-items: center; .lottie-player { width: 50%; height: 50%; transform: scale(0.5); filter: drop-shadow(0px 0px 10px rgba(255, 255, 255, 0.8)); &.animated { animation-duration: 7s; animation-iteration-count: infinite; animation-name: rainbow-animation; } } @keyframes rainbow-animation { 0% { filter: drop-shadow(0px 0px 10px rgba(255, 0, 0, 0.8)); } 16% { filter: drop-shadow(0px 0px 10px rgba(255, 0, 0, 0.8)); } 33% { filter: drop-shadow(0px 0px 10px rgba(255, 165, 0, 0.8)); } 50% { filter: drop-shadow(0px 0px 10px rgba(255, 255, 0, 0.8)); } 66% { filter: drop-shadow(0px 0px 10px rgba(0, 128, 0, 0.8)); } 83% { filter: drop-shadow(0px 0px 10px rgba(0, 0, 255, 0.8)); } 100% { filter: drop-shadow(0px 0px 10px rgba(238, 130, 238, 0.8)); } } } </style>
In the above code, we use the Lottie Animation component to render the Lottie animation, and use the require function to introduce the downloaded Lottie JSON file into the Vue component. We also introduced the animate.css library into the component, so all animate.css animations can be used in CSS.
Specify the lottieAnimation declaration as an empty object so that the correct animation is assigned in the mounted lifecycle hook, and then specify the animation-data property in the Lottie Animation component. Finally, create a CSS animation named "rainbow-animation" and apply it to the Lottie Animation component.
Step 6: Add the component to App.vue
Now we can add the Rainbow component to our application. Edit the App.vue file and add the following code:
<template> <div id="app"> <Rainbow /> </div> </template> <script> import Rainbow from '@/components/Rainbow.vue' export default { name: 'App', components: { Rainbow } } </script>
Here, we import the Rainbow component and use it in #app
.
Step 7: Run the application
Now you can run the application on localhost:8080 using the npm run serve
command.
Open this URL in your browser and you will see a beautiful rainbow animation effect!
Summary
Implementing a rainbow chart CSS animation using Vue may seem tricky, but using the Vue CLI and a few tools and libraries, it can be a breeze. In this article, we installed the Vue CLI and some required npm packages, added the LottieJSON file and CSS animations to the Vue component, and used it in the App.vue file. Now you can see amazing rainbow animation effects in the app.
The above is the detailed content of How to use Vue to implement rainbow chart CSS animation?. For more information, please follow other related articles on the PHP Chinese website!
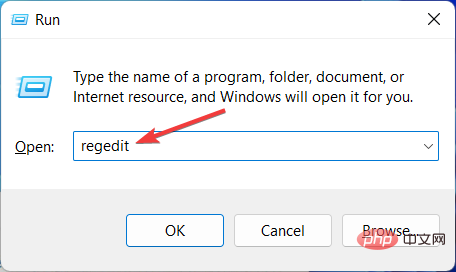
当微软推出Windows11时,它带来了许多变化。其中一项更改是增加了用户界面动画的数量。一些用户想要改变事物的出现方式,他们必须想办法去做。拥有动画让用户感觉更好、更友好。动画使用视觉效果使计算机看起来更具吸引力和响应能力。其中一些包括几秒钟或几分钟后的滑动菜单。计算机上有许多动画会影响PC性能、减慢速度并影响您的工作。在这种情况下,您必须关闭动画。本文将介绍用户可以提高其在PC上的动画速度的几种方法。您可以使用注册表编辑器或您运行的自定义文件来应用更改。如何提高Windows11动画的
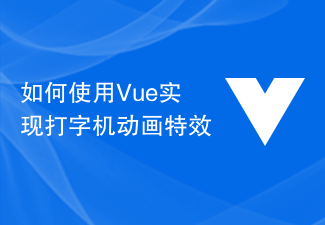
如何使用Vue实现打字机动画特效打字机动画是一种常见且引人注目的特效,常用于网站的标题、标语等文字展示上。在Vue中,我们可以通过使用Vue自定义指令来实现打字机动画效果。本文将详细介绍如何使用Vue来实现这一特效,并提供具体的代码示例。步骤1:创建Vue项目首先,我们需要创建一个Vue项目。可以使用VueCLI来快速创建一个新的Vue项目,或者手动在HT
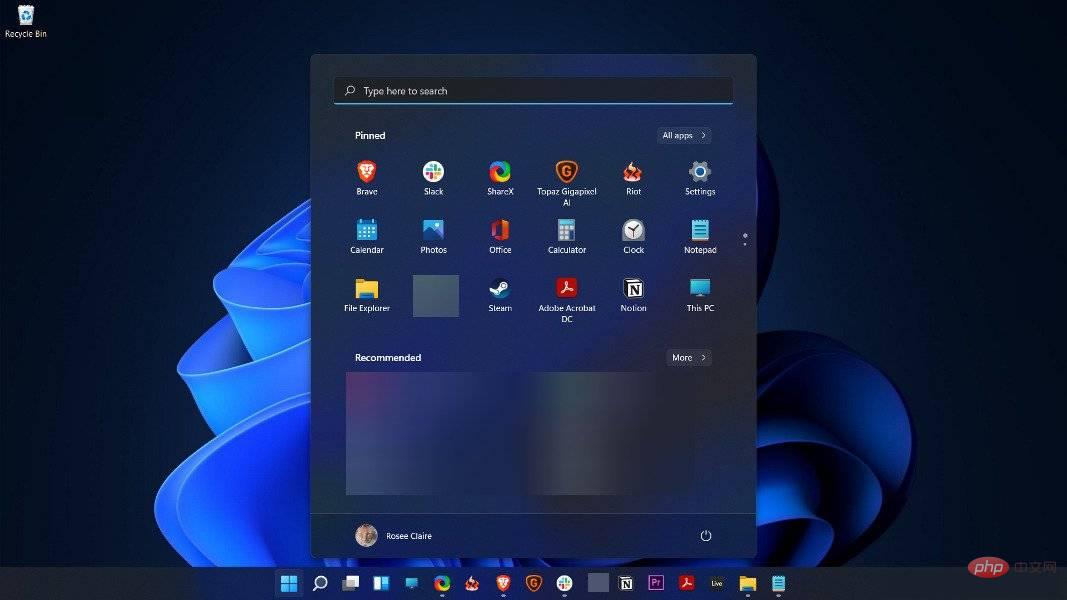
MicrosoftWindows11中包含多项新特性和功能。用户界面已更新,公司还引入了一些新效果。默认情况下,动画效果应用于控件和其他对象。我应该禁用这些动画吗?尽管Windows11具有视觉上吸引人的动画和淡入淡出效果,但它们可能会导致您的计算机对某些用户来说感觉迟钝,因为它们会为某些任务增加一点延迟。关闭动画以获得更灵敏的用户体验很简单。在我们看到对操作系统进行了哪些其他更改后,我们将引导您了解在Windows11中打开或关闭动画效果的方法。我们还有一篇关于如何在Windows
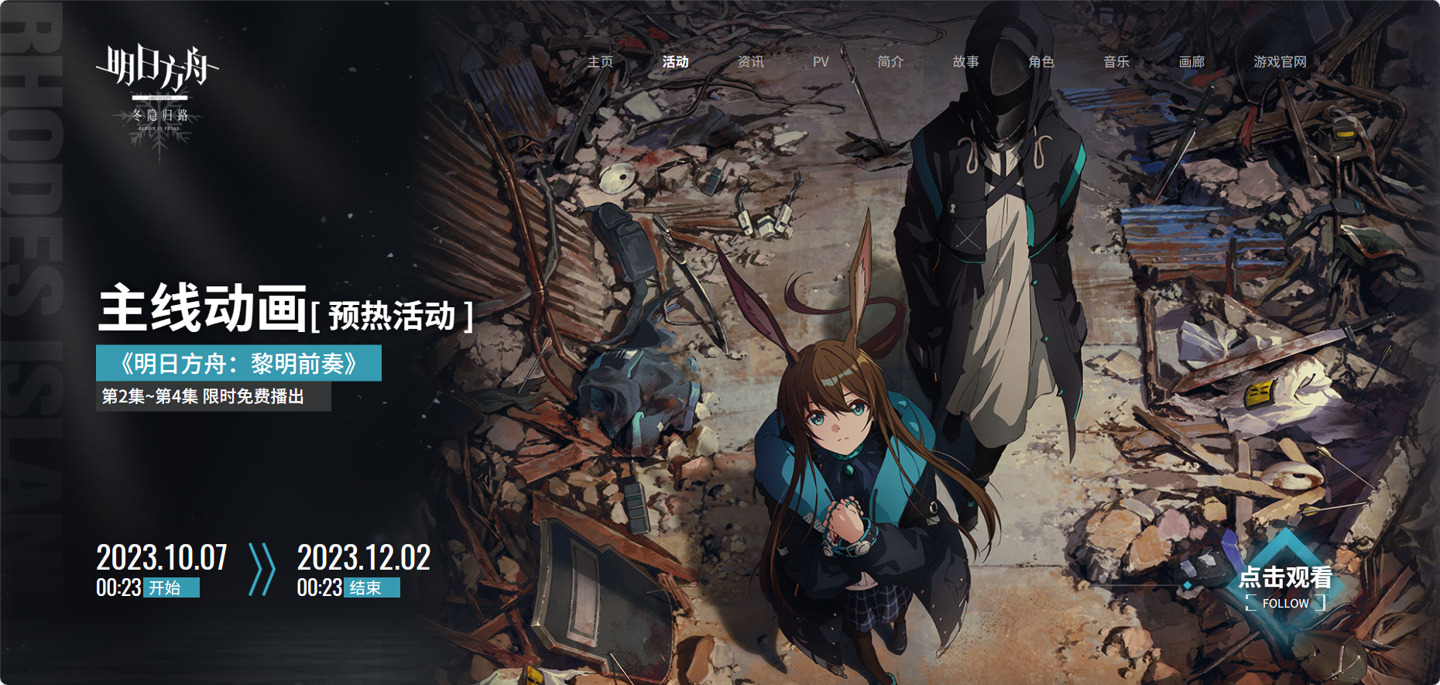
本站需要重新写作的内容是:9需要重新写作的内容是:月需要重新写作的内容是:23需要重新写作的内容是:日消息,动画剧集《明日方舟》的第二季主线剧《明日方舟:冬隐归路》公布定档需要重新写作的内容是:PV,将于需要重新写作的内容是:10需要重新写作的内容是:月需要重新写作的内容是:7需要重新写作的内容是:日需要重新写作的内容是:00:23需要重新写作的内容是:正式上线,点此进入主题官网。需要重新写作的内容是:本站注意到,《明日方舟:冬隐归路》是《明日方舟:黎明前奏》的续作,剧情简介如下:为阻止感染者组
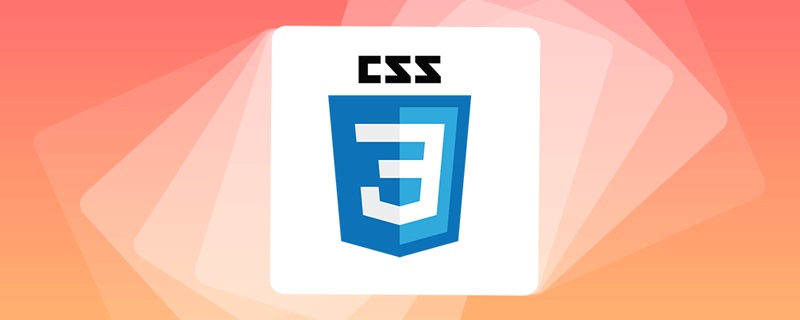
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
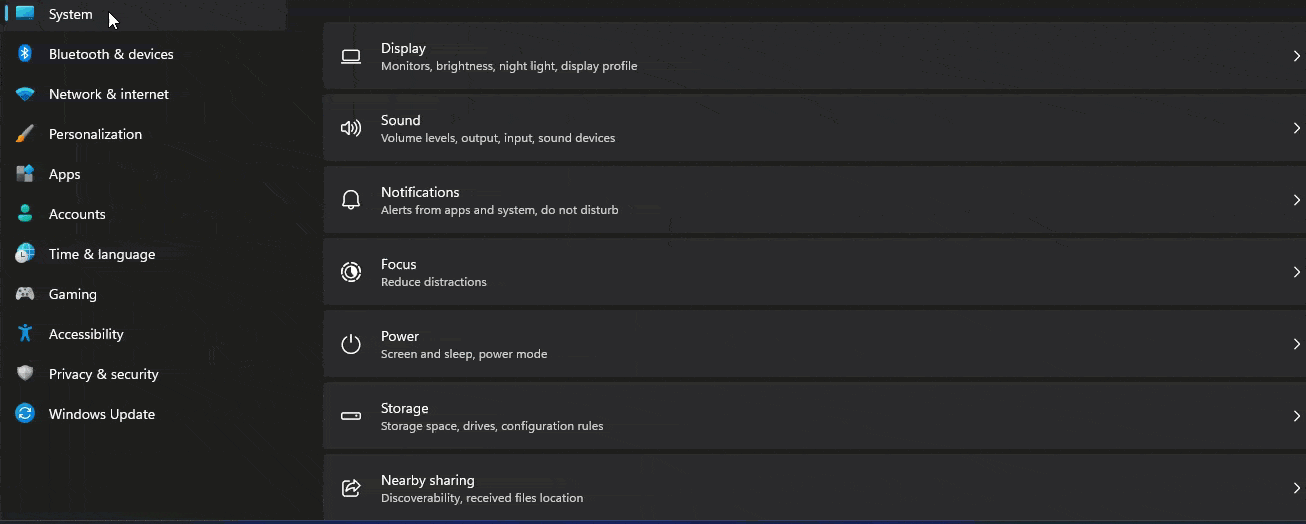
微软正在Windows11中试验新的任务栏动画,这是这家软件巨头正在进行的另一项新测试。这一次在设置应用程序中,当您单击相应部分时,图标会显示动画。以下是如何在Windows11中为“设置”应用启用图标动画。您可以在Windows11中看到特殊的动画和动画效果。例如,当您最小化和最大化设置应用程序或文件资源管理器时,您会注意到动画。说到图标,当您最小化窗口时,您会看到一个图标会向下弹起,而在您最大化或恢复时,它会弹起。Windows11设置可能会新收到左侧显示的导航图标动画,这是您
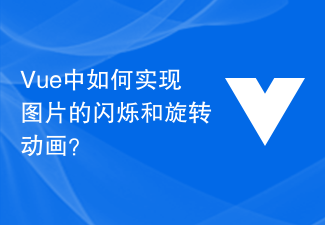
Vue中如何实现图片的闪烁和旋转动画Vue.js是目前非常流行的前端框架之一,它提供了强大的工具来管理和展示页面中的数据。在Vue中,我们可以通过添加CSS样式和动画来使元素产生各种各样的效果。本文将介绍如何使用Vue和CSS来实现图片的闪烁和旋转动画。首先,我们需要准备一张图片,可以是本地的图片文件或者网络上的图片地址。我们将使用<img>标
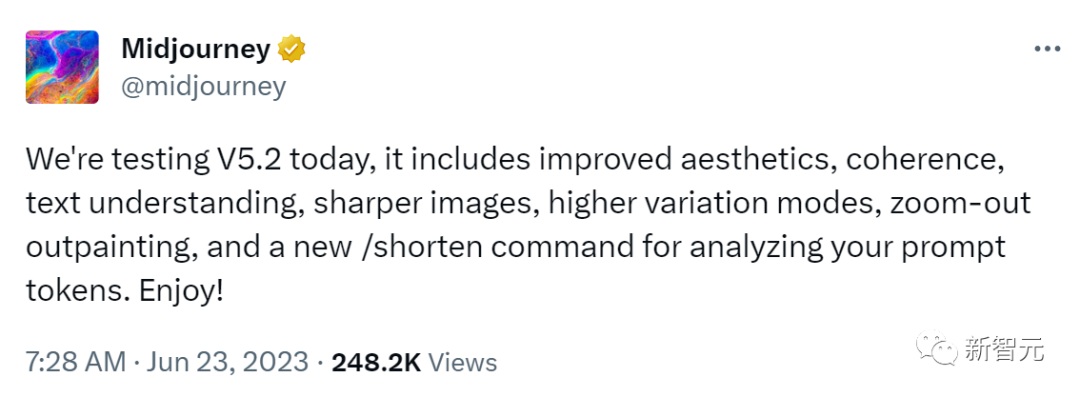
Midjourney和StableDiffusion,已经卷到没边了!几乎在StableDiffusionXL0.9发布的同一时间,Midjourney宣布推出了5.2版本。此次5.2版本最亮眼的更新在于zoomout功能,它可以无限扩展原始图像,同时保持跟原始图像的细节相同。用zoomout做出的无垠宇宙动画,直接让人震惊到失语,可以说,Midjourney5.2看得比詹姆斯韦伯太空望远镜还要远!这个极其强大的功能,可以创造出非常神奇的图片,甚至还能被用来拍摄毫无破绽的高清变焦视频!这个「核弹


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
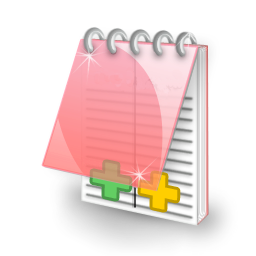
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use