


With the continuous development of modern Internet applications, more and more applications need to handle large amounts of data communication. The traditional way of handling these data communications is to use polling or blocking I/O, but these methods can no longer meet the needs of modern applications because they are very inefficient. In order to solve this problem, the industry has developed a technology called message queue and distribution system.
In the message queue and distribution system, the producer of the message sends the message to the queue, and the consumer of the message obtains the message from the queue and performs corresponding operations. This approach can greatly improve the efficiency of data communication because it can avoid problems such as polling and blocking I/O.
In this article, we will discuss how to achieve efficient message queuing and distribution using PHP and Apache Kafka integration.
Introduction to Apache Kafka
Apache Kafka is a high-throughput, low-latency, scalable distributed messaging system. It can handle large volumes of messages and scale horizontally to accommodate higher loads. The main components of Apache Kafka include:
- Broker: Each node in the Kafka cluster is a broker, and they are responsible for the storage and forwarding of messages.
- Topic: Each message must be assigned to a topic, which is the logical concept of message production and consumption.
- Partition: Each topic can be divided into multiple partitions, and each partition contains multiple ordered messages.
- Producer: Message producer, sends messages to broker.
- Consumer: Message consumer, reads messages from the broker.
- Consumer Group: A group of consumers jointly consume messages in one or more partitions.
- Offset: The number of the message, used to uniquely identify a message.
PHP integrates Apache Kafka
In order to use Apache Kafka, we need to use the Kafka extension of PHP. This extension provides all the APIs required by PHP to operate Kafka.
First, we need to install the Kafka extension. We can install it from PECL:
pecl install kafka
After installing the extension, you can start using it. The following is a simple example of message production and consumption using PHP and Apache Kafka:
<?php $brokers = 'kafka:9092'; // Kafka集群地址 $topic = 'test'; // Topic名称 // 创建一个Kafka生产者 $producer = new RdKafkaProducer(); $producer->setLogLevel(LOG_DEBUG); $producer->addBrokers($brokers); // 创建一个Kafka消费者 $conf = new RdKafkaConf(); $conf->set('group.id', 'myGroup'); $consumer = new RdKafkaConsumer($conf); $consumer->addBrokers($brokers); // 生产消息 $topicProducer = $producer->newTopic($topic); for ($i = 0; $i < 10; $i++) { $topicProducer->produce(RD_KAFKA_PARTITION_UA, 0, 'Message ' . $i); } // 消费消息 $topicConsumer = $consumer->newTopic($topic); $topicConsumer->consumeStart(0, RD_KAFKA_OFFSET_BEGINNING); while (true) { $message = $topicConsumer->consume(0, 1000); if (null === $message) { continue; } if ($message->err) { throw new Exception('Error occurred while consuming message'); } echo $message->payload . PHP_EOL; }
In this example, we first create a Kafka producer and a Kafka consumer. Then, in the producer, we sent 10 messages to the specified topic; in the consumer, we consumed the messages from the specified topic and output their contents.
At this point, we have successfully implemented simple message production and consumption using PHP and Apache Kafka. Next, we'll discuss how to implement more advanced functionality using PHP and Apache Kafka.
Advanced application examples
In actual applications, we usually need to implement some advanced functions, such as:
- Message distribution: send messages to specified consumers .
- Consumer group: allows multiple consumers to jointly consume messages in one or more topics.
- offset configuration: allows controlling the reading position of messages.
Here we will discuss how to implement these functions.
Message Distribution
In practical applications, we usually need to control the flow of messages. For example, we may want only certain consumers to consume certain messages. To achieve this functionality, we can create a queue for each consumer and then assign specific messages to specific queues.
The following is an example that uses two consumers to consume two different tasks.
<?php $brokers = 'kafka:9092'; // Kafka集群地址 $topic = 'test'; // Topic名称 // 创建一个Kafka消费者组 $conf = new RdKafkaConf(); $conf->set('group.id', 'myGroup'); $consumer = new RdKafkaKafkaConsumer($conf); $consumer->subscribe([$topic]); // 创建两个Kafka生产者,一个生产者用于向消费者1发送消息,另一个生产者用于向消费者2发送消息 $producer1 = new RdKafkaProducer(); $producer1->addBrokers($brokers); $producer1Topic = $producer1->newTopic($topic . '_1'); $producer2 = new RdKafkaProducer(); $producer2->addBrokers($brokers); $producer2Topic = $producer2->newTopic($topic . '_2'); // 消费消息 while (true) { $message = $consumer->consume(1000); if (null === $message) { continue; } if ($message->err) { throw new Exception('Error occurred while consuming message'); } echo 'Received message: ' . $message->payload . PHP_EOL; // 根据消息内容分配给不同的生产者 if ($message->payload === 'task1') { $producer1Topic->produce(RD_KAFKA_PARTITION_UA, 0, $message->payload); } elseif ($message->payload === 'task2') { $producer2Topic->produce(RD_KAFKA_PARTITION_UA, 0, $message->payload); } }
In this example, we use two producers to distribute messages to two different consumers. When a consumer receives a message, we can assign it to a specific producer based on the message content. This method can help us control the flow of messages and avoid redundant processing of messages.
Consumer Group
In ordinary Kafka consumers, different consumers in the same group consume the same topic and they will receive the same message. This is because Kafka automatically balances partitions and ensures that each partition is processed by only one consumer.
In PHP, we can use group.id to group consumers to realize the function of consumer groups.
The following is an example of a Kafka consumer group that can process messages within the same group in parallel:
<?php $brokers = 'kafka:9092'; // Kafka集群地址 $topic = 'test'; // Topic名称 // 创建一个Kafka消费者组 $conf = new RdKafkaConf(); $conf->set('group.id', 'myGroup'); $conf->set('metadata.broker.list', $brokers); $conf->set('enable.auto.commit', 'false'); $consumer = new RdKafkaKafkaConsumer($conf); // 添加需要订阅的topic $consumer->subscribe([$topic]); // 处理消息 while (true) { $message = $consumer->consume(1000); if (null === $message) { continue; } if ($message->err) { throw new Exception('Error occurred while consuming message'); } echo 'Received message: ' . $message->payload . PHP_EOL; // 处理完消息后手动提交offset $consumer->commit(); }
In this example, we create a Kafka consumer group and send it to Added topics that require subscription. We can then process messages within the same group in parallel.
Note: In a consumer group, multiple consumers consume one or more partitions together. When consuming data, you need to pay attention to the issue of multi-thread processing of the same data.
Offset configuration
In Kafka, each partition has an independent offset. The consumer can control where in the partition it reads and thus which messages it reads. The consumer can start reading from the last message or the latest message.
In PHP, we can use offset to control the reading position of messages. The following is an example of Offset configuration:
<?php $brokers = 'kafka:9092'; // Kafka集群地址 $topic = 'test'; // Topic名称 // 创建一个Kafka消费者 $conf = new RdKafkaConf(); $conf->set('group.id', 'myGroup'); $consumer = new RdKafkaKafkaConsumer($conf); // 订阅topic $topicConf = new RdKafkaTopicConf(); $topicConf->set('auto.offset.reset', 'earliest'); $topic = $consumer->newTopic($topic, $topicConf); $topic->consumeStart(0, RD_KAFKA_OFFSET_STORED); // 消费消息 while (true) { $message = $topic->consume(0, 1000); if (null === $message) { continue; } if ($message->err) { throw new Exception('Error occurred while consuming message'); } echo 'Received message: ' . $message->payload . PHP_EOL; }
In this example, we use auto.offset.reset to set the offset configuration. This configuration tells the consumer to start consuming messages from the earliest offset.
In actual applications, different offsets can be configured according to needs. For example, after the producer fails to process some messages, we may need to restart reading messages from the point where the failed message was previously processed.
Conclusion
In this article, we discussed how to achieve efficient message queuing and distribution using PHP and Apache Kafka integration. We first introduced the basics of Apache Kafka and then discussed how to use the Kafka extension for PHP to implement the production and consumption of messages. Finally, we discussed how to implement some advanced features such as message distribution, consumer groups, and offset configuration.
Using PHP and Apache Kafka integration allows us to implement efficient message queuing and distribution, thereby improving application response speed and throughput. If you are developing an application that needs to handle large amounts of data communication, Apache Kafka and the Kafka extension for PHP may be a good choice.
The above is the detailed content of PHP and Apache Kafka integration for efficient message queuing and distribution. For more information, please follow other related articles on the PHP Chinese website!
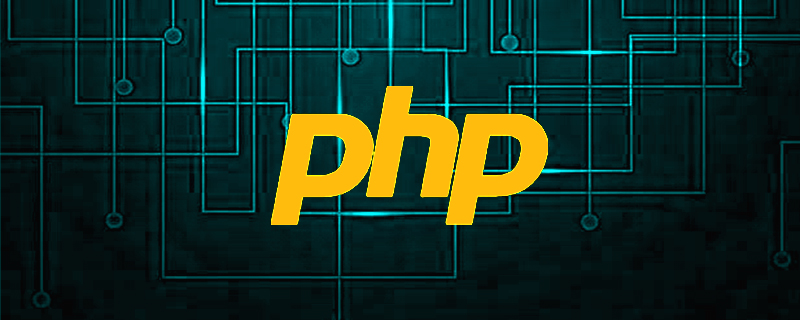
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
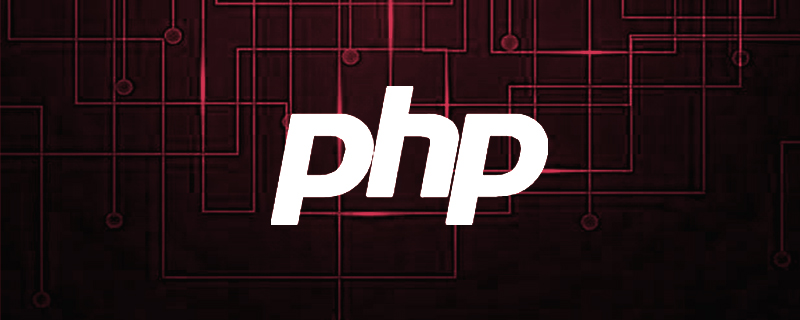
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
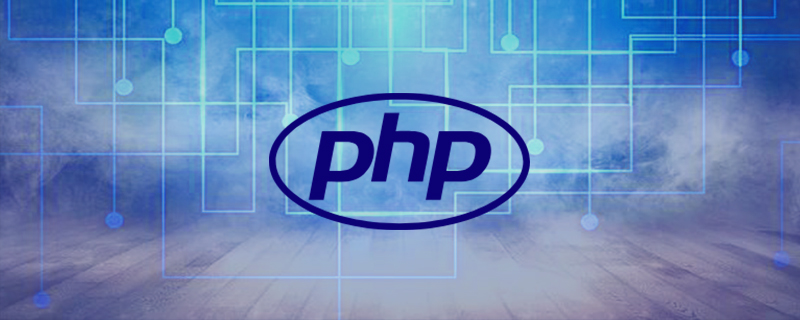
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
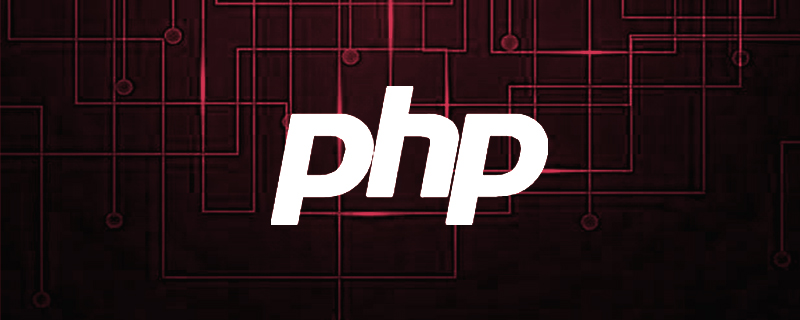
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
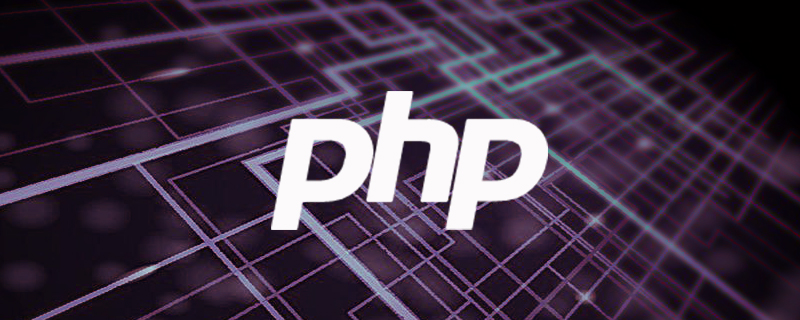
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
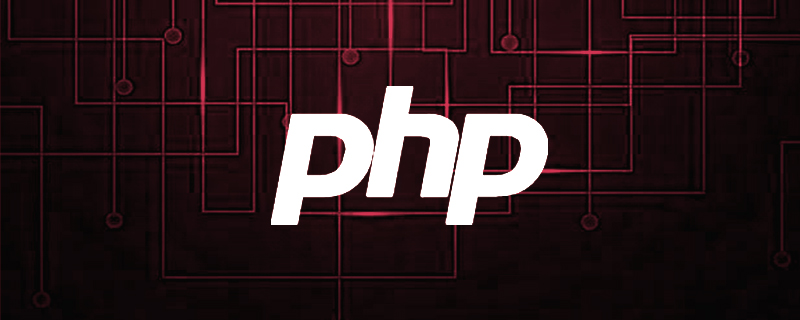
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
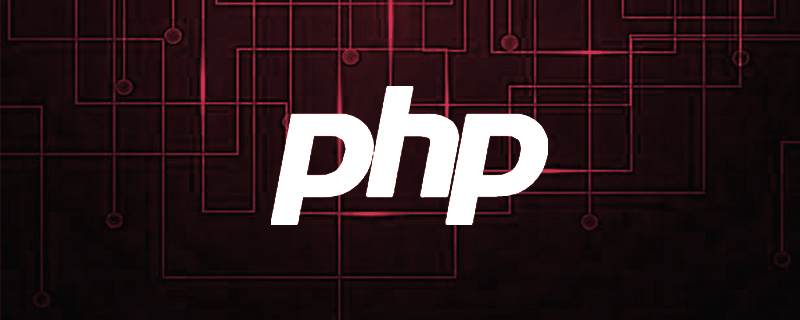
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
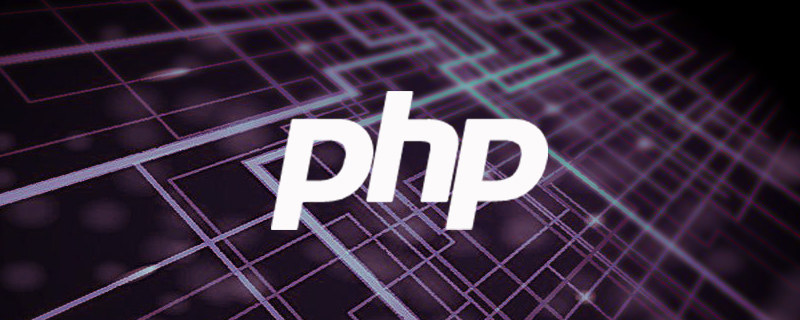
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
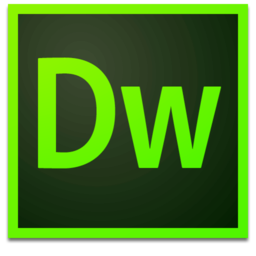
Dreamweaver Mac version
Visual web development tools
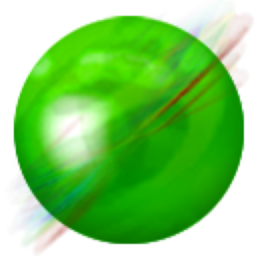
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
