Detailed explanation of code splitting and lazy loading techniques in Vue
With the rapid development of front-end technology, modern web applications have become more and more complex. These applications need to process more data and more complex business logic, which makes JavaScript applications become larger and larger. This may result in slower application startup and poor user experience. To this end, Vue provides some advanced features to help optimize your application. The most important of these are code splitting and lazy loading techniques.
This article will explain in detail the code splitting and lazy loading techniques in Vue. We’ll explore what code splitting is, why you need to use it in a Vue application, and how to implement it. Along the way, we’ll also discuss what lazy loading techniques are, why they’re important, and how to use them in Vue.
Code Splitting
Code splitting is a technique that splits your code into small pieces and loads them on demand when needed. Code splitting can help reduce initial load times and improve access speed, especially as your application grows larger.
Vue provides two methods of code splitting: through dynamic import (Dynamic Import) or using a third-party library.
Dynamic import solution
Using dynamic import can split the application into smaller code blocks, so that they can be loaded on demand when needed. Dynamic import needs to be used with Webpack. Webpack can package the required chunks into separate files and load these chunks only when the user requests them.
Now let’s look at a simple example. Suppose we have an App component that contains a User component that displays user information. We can split the User component into a code block. When the user accesses the App component, the User component will only be loaded when it is needed:
const App = { data() { return { user: null } }, methods: { async loadUserData() { const userModule = await import('./user.js') this.user = userModule.default } }, template: ` <div> <button @click="loadUserData()">Load User Data</button> <div v-if="user"> <h2 id="user-name">{{ user.name }}</h2> <p>{{ user.bio }}</p> </div> </div> ` }
In this example, we use the import function to dynamically import user.js module. When the user clicks the "Load User Data" button, the module is loaded and set as the user data for the App component. If the user doesn't click the button, the User component will never be loaded.
Third-party library solution
In addition to dynamic import, you can also use third-party libraries to achieve code splitting. Vue recommends using the @babel/plugin-syntax-dynamic-import
plugin to support dynamic import.
After using this plug-in, you can use dynamic import syntax in your application. For example:
const Foo = () => import('./Foo.vue')
This statement will return a Promise, which will output an ES modular object after loading is complete.
Lazy loading
Lazy loading is similar to dynamic import. The difference is that lazy loading is a method of on-demand loading that splits your code into small chunks and loads them only when needed. This reduces initial load time and improves access speed.
Lazy loading in Vue can be implemented using webpack and ES modules. Below are two ways to achieve this.
Lazy loading through vue-router
Vue router can implement lazy loading by defining components as asynchronous components. Asynchronous components return a Promise, which will output a Vue component when loading is complete.
Here is an example of using an asynchronous component to load a User component:
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) const router = new VueRouter({ routes: [ { path: '/user/:id', component: () => import('./components/User.vue') } ] })
In this example, we use an asynchronous component in the router configuration. When a user accesses the "/user/:id" path, the User component will be loaded asynchronously.
Lazy loading through webpack
Any component in a Vue application can be defined as an asynchronous component. Webpack packages asynchronous components into separate files and loads them when the user requests them.
Here is an example of loading a User component using lazy loading in Webpack:
const User = () => import(/* webpackChunkName: "user" */ './User.vue')
In this example, we create a User component and define it as an asynchronous component. Webpack packages user components into a separate file named "user.chunk.js".
Finally, we use Vue Router or Webpack to achieve code splitting and lazy loading, thereby speeding up the loading speed of the application and optimizing the user experience. You just need to use the above tips to split your code into small pieces and load them on demand.
The above is the detailed content of Detailed explanation of code splitting and lazy loading techniques in Vue. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
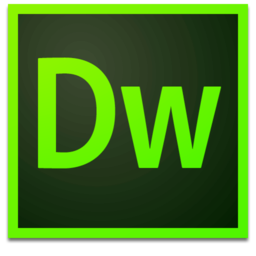
Dreamweaver Mac version
Visual web development tools
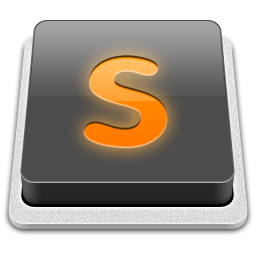
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
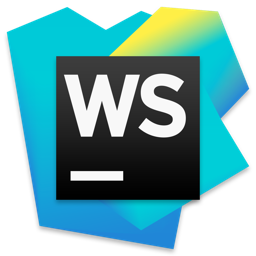
WebStorm Mac version
Useful JavaScript development tools