Solution to UnsupportedEncodingException exception in Java
UnsupportedEncodingException may occur in Java, mainly because the encoding is not supported. When processing text data, it is often necessary to perform encoding conversion, that is, to convert the content of one encoding format into the content of another encoding format. If the encoding type used for encoding conversion is not supported, an UnsupportedEncodingException exception will be thrown. This article will introduce the solution to this exception.
1. Understand Encoding
In Java, if the encoding method is not specified, the system default encoding method will be used by default. This may lead to situations where the encoding is not supported. Therefore, before performing encoding conversion, you must first understand the different encoding methods.
Common encoding methods include ASCII, ISO-8859-1, UTF-8, UTF-16, etc. Among them, ASCII and ISO-8859-1 are single-byte encoding methods that can only represent English letters and some common symbols, but cannot represent characters in other languages such as Chinese. UTF-8 and UTF-16 are multi-byte encodings that can represent all characters in different languages.
2. Solve the UnsupportedEncodingException exception
- Check the encoding format
When converting the encoding, first check whether the encoding format of the converted content is supported . If an unsupported encoding format is used, an UnsupportedEncodingException exception will be thrown. Therefore, in order to avoid the occurrence of this exception, you need to check the encoding format of the converted content to ensure that a supported encoding method is used.
- Specify the correct encoding method
When performing encoding conversion, you must specify the correct encoding method. If no encoding is specified or an incorrect encoding is specified, an UnsupportedEncodingException will be thrown.
In Java, you can use the getBytes method of a string for encoding conversion, which can obtain a byte array in a specified encoding format from a string. For example:
String str = "中国"; byte[] utf8Bytes = str.getBytes("UTF-8");
The above code will obtain the UTF-8 encoded byte array of the str string. If the specified encoding format is not supported, an UnsupportedEncodingException exception will be thrown.
- Handling exceptions
If an UnsupportedEncodingException exception occurs in the program, the exception needs to be handled. Usually, you can use try-catch in the method to handle the exception to avoid program crash. For example:
public static void main(String[] args) { try { String str = "中国"; byte[] utf8Bytes = str.getBytes("UTF-8"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } }
In the above code, if the specified encoding method is not supported, an UnsupportedEncodingException exception will be thrown. Use try-catch to catch the exception and print the exception information. When the program is running, if an exception occurs, it will not crash, but will output exception information.
Summary
When performing encoding conversion, pay attention to whether the encoding format of the content being converted is supported, and specify the correct encoding method. If an UnsupportedEncodingException exception occurs, you need to handle the exception to ensure that the program does not crash. In actual development, coding-related issues need to be handled carefully to ensure the stability and reliability of the program.
The above is the detailed content of Solution to UnsupportedEncodingException exception in Java. For more information, please follow other related articles on the PHP Chinese website!
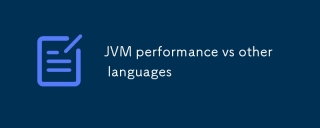
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
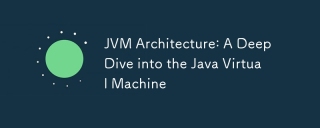
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
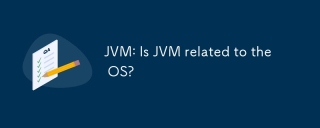
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
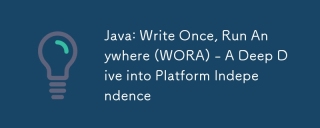
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
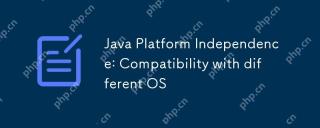
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
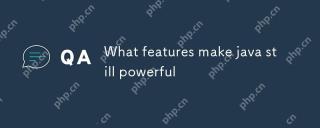
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
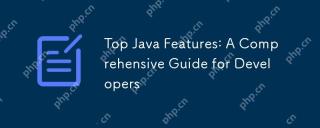
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
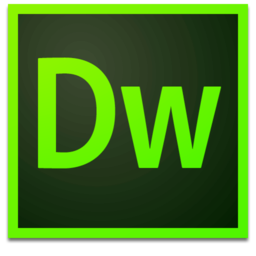
Dreamweaver Mac version
Visual web development tools
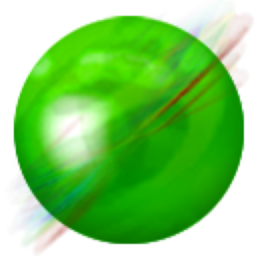
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
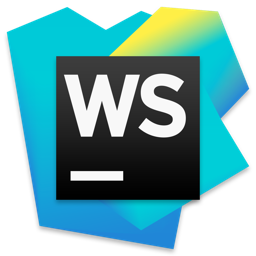
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
