Vue is an excellent front-end framework. When processing large amounts of data, the paging component is essential. The pagination component can make the page tidier and also improve the user experience. In Vue, implementing a paging component is not complicated. This article will introduce how Vue implements a paging component.
1. Requirements analysis
Before implementing the paging component, we need to analyze the requirements. A basic paging component needs to have the following functions:
- Display the current number of pages, the total number of pages and the number of items displayed on each page
- Click the paging button to switch to different pages
- Display the data of the current page
- Highlight the current page number
- Display the paging bar number selector
2. Implementation steps
- Dividing files
To facilitate management, we split the paging component into three files:
- Pagination.vue: the main file of the paging component, mainly Responsible for logic and event handling.
- PaginationItem.vue: A subcomponent of the pagination component, mainly responsible for rendering the pagination button.
- SelectPerPage.vue: paging item selector component, mainly responsible for rendering the item selection drop-down box and processing change events.
- Binding of paging data
We need to define some variables to store paging-related data, including the current page number, the number of items per page, and the total number of pages . These variables should be defined in Pagination.vue. At the same time, we also need to introduce a props attribute to receive the data passed by the parent component.
export default { props: { currentPage: { // 当前页码 type: Number, required: true }, total: { // 总记录数 type: Number, required: true }, perPage: { // 每页展示条数 type: Number, required: true } }, data () { return { pages: Math.ceil(this.total / this.perPage), // 总页数 pageList: [] // 存储当前页面展示的页码 } }, mounted () { this.getPageList(this.currentPage) } }
- Rendering the paging button
We split the paging button into a sub-component, which is mainly used to render the paging button and handle paging switching events. The content of PaginationItem.vue is as follows:
<template> <li :class="{ active: active }"> <a @click.prevent="handleClick" href="#"> {{ label }} </a> </li> </template> <script> export default { props: { label: { // 按钮上的数字或图标 required: true }, pageNum: { // 按钮代表的页码 required: true }, active: { // 判断按钮是否处于激活状态 default: false } }, methods: { // 点击分页按钮时触发 handleClick () { this.$emit('change', this.pageNum) } } } </script>
In Pagination.vue, we need to use the v-for directive to render multiple PaginationItem.vue components. The number of pages should be generated according to certain rules. For this rule, you can refer to the following code:
methods: { // 获取当前页显示的页码 getPageList (currentPage) { let pageList = [] // 存储页码数据 const totalPages = this.pages // 总页数 const visiblePages = 5 // 按钮可见的页码数 const half = Math.floor(visiblePages / 2) // 可见页码数的一半 // 如果总页数小于可显示页数 if (totalPages <= visiblePages) { for (let i = 1; i <= totalPages; i++) { pageList.push(i) } } else { // 如果当前页码小于或等于可见页码数的一半 if (currentPage <= half) { for (let i = 1; i <= visiblePages; i++) { pageList.push(i) } } else if (currentPage >= totalPages - half) { // 如果当前页码大于等于最后一页减去可见页码数的一半 for (let i = totalPages - visiblePages + 1; i <= totalPages; i++) { pageList.push(i) } } else { for (let i = currentPage - half; i <= currentPage + half; i++) { pageList.push(i) } } } this.pageList = pageList } }
- Pagination switching
We need to bind the paging switching event to Pagination.vue on the component. This event should be triggered by the PaginationItem.vue subcomponent and pass the switched page number as a parameter.
In Pagination.vue, we need to add a new method nextPage to update the current page number. At the same time, we also need to introduce a calculated attribute currentIndex to obtain the index of the current page. The code is as follows:
methods: { // 点击页码时触发 handlePageChange (pageNum) { // 如果页码为负数或者大于总页数都停止执行 if (pageNum <= 0 || pageNum > this.pages) return this.currentPage = pageNum this.$emit('change-page', pageNum) // 事件广播向父组件传值 this.getPageList(pageNum) }, // 增加当前页码 nextPage () { if (this.currentIndex < this.pages - 1) { this.currentPage++ this.getPageList(this.currentPage) this.$emit('change-page', this.currentPage) // 事件广播向父组件传值 } } }, computed: { currentIndex () { // 当前页码所在的索引 return this.currentPage - 1 } }
- Page number selection drop-down box
In order to facilitate users to switch the number of records displayed on each page, we need to implement a drop-down box component. This component should be implemented by the SelectPerPage.vue file.
The content of SelectPerPage.vue is as follows:
<template> <div class="select-per-page"> <select :value="perPage" @change="changePerPage"> <option v-for="item in items" :value="item">{{ item }}</option> </select> </div> </template> <script> export default { props: { perPage: { // 每页展示条数 type: Number, required: true }, options: { // 可选的条数设置 type: Array, default () { return [10, 20, 30, 50] } } }, computed: { items () { return this.options.map(option => `${option} 条`) } }, methods: { // 切换每页展示的条数 changePerPage (event) { const perPage = +event.target.value.replace(/[D]/g, '') this.$emit('update:perPage', perPage) this.$emit('change-per-page', perPage) // 事件广播向父组件传值 } } } </script>
The above is the entire content of Vue to implement the paging component. We need to introduce Pagination.vue in the parent component, and then pass the corresponding parameters such as currentPage, total and perPage. In this way, a reusable paging component can be implemented, which improves the efficiency and development experience of front-end development.
The above is the detailed content of How to implement paging component in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
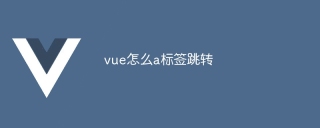
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
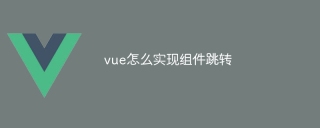
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),