Golang is a strongly typed, compiled language. Its speed, powerful concurrency features and concise syntax make it a popular language in cloud native application development. However, when developing with Golang, you may come across some bugs. One of the common errors is "undefined: fmt.Errorf". In this article, we will explore the causes and solutions to this error.
Error reason
In Golang, the fmt package is a very commonly used package, which provides formatting and output functions. Among them, fmt.Errorf is a function used to create a new error that implements the error interface. For example:
import "fmt" func someFunc() error { if err := someOtherFunc(); err != nil { return fmt.Errorf("Something went wrong: %v", err) } return nil }
In the above example, the someFunc function calls the someOtherFunc function and returns the error as the parameter of the fmt.Errorf function. The function then returns a new error containing some description of the original error. This is the typical way of handling errors in Golang.
However, when you compile the code, you may encounter a compilation error: "undefined: fmt.Errorf". This is because you are using a function that is not defined in the fmt package.
Solution
To solve this problem, there are several methods you can try.
Method 1: Confirm Golang version
First, make sure you are using a version that supports fmt.Errorf. fmt.Errorf was introduced starting in Go 1.13. Therefore, if your Golang version is lower than 1.13, fmt.Errorf is not available.
To check your Golang version, open a terminal and run the following command:
go version
If your Golang version is lower than 1.13, try upgrading it to the latest version. You can download the latest version on the [official Golang website](https://golang.org/dl/).
Method 2: Use the errors package
If your Golang version still does not support fmt.Errorf, you can use the errors package instead. The errors package provides a New function, which is used to create a new error that implements the error interface. For example:
import "errors" func someFunc() error { if err := someOtherFunc(); err != nil { return errors.New(fmt.Sprintf("Something went wrong: %v", err)) } return nil }
In the above example, we use the errors.New function to create a new error containing some description about the original error.
Method 3: Manually implement an error type
Finally, you can also manually create an error type and implement the error interface. For example:
type MyError struct { message string } func (e *MyError) Error() string { return fmt.Sprintf("MyError: %s", e.message) } func someFunc() error { if err := someOtherFunc(); err != nil { return &MyError{message: fmt.Sprintf("%v", err)} } return nil }
In the above example, we created a custom MyError error type and implemented the Error() method in the error interface. Now we can use this error type in some functions to return errors.
Summary
In Golang, fmt.Errorf is one of the common ways to create new errors that implement the error interface. However, when encountering the error "undefined: fmt.Errorf", you can solve the problem by confirming the Golang version, using the errors package, or manually implementing an error type. I believe the content of this article can help you handle this type of error correctly.
The above is the detailed content of How to solve 'undefined: fmt.Errorf' error in golang?. For more information, please follow other related articles on the PHP Chinese website!
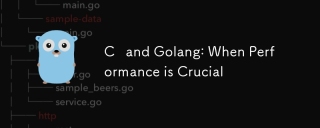
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
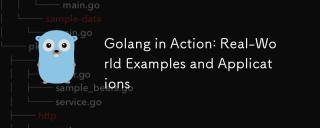
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
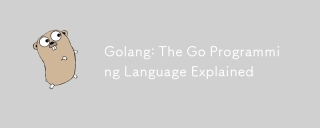
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
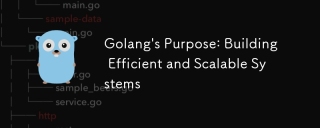
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
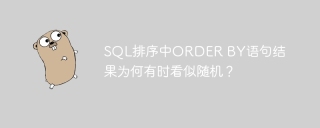
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
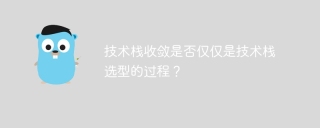
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
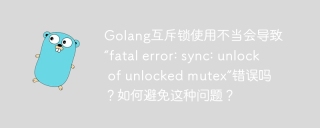
Golang ...
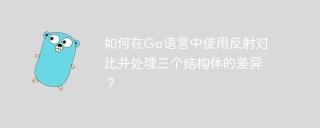
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
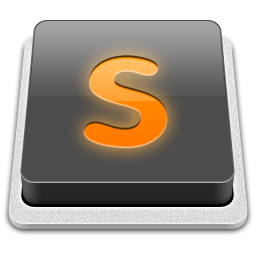
SublimeText3 Mac version
God-level code editing software (SublimeText3)