


How to solve the non-standard file reading error in Python code?
In Python, file reading is a very common operation. However, due to irregularities or sloppiness on the part of the code writer, errors in the way the file is read may result. These errors can cause program crashes, waste time, and even create security risks. This article will introduce how to solve common non-standard file reading errors in Python code.
- Use absolute paths
In Python, file paths are often used to locate files when reading files. However, using relative paths may cause file reading errors because file paths are calculated relative to the current working directory. The solution to this problem is to use absolute paths. You can use the function os.path.abspath() to get the absolute path of the file, as shown below:
import os path = os.path.abspath('file.txt')
'file.txt' here represents the file name. This function can ensure that the file is always correctly located and will not cause reading errors due to changes in the file path.
- Check whether the file exists
Before reading the file, it is best to check whether the file exists to avoid causing program errors if the file does not exist. You can use the function os.path.exists() to check whether the file exists, as shown below:
import os path = 'file.txt' if os.path.exists(path): with open(path, 'r') as f: # 读取文件内容 else: print('File does not exist!')
Here path is the file path. If the file exists, open it for reading; otherwise, print an error message.
- Use the with statement
When using Python to read a file, use the with statement to ensure that the file is closed correctly after use and avoid resource leaks. Inside the with statement, you can perform a series of file operations such as reading and writing, as shown below:
path = 'file.txt' with open(path, 'r') as f: # 读取文件内容
'file.txt' here is the file name, and 'r' means opening the file in read-only mode . At the end of the with statement, the file is automatically closed without manual closing.
- Use try-except block
During the process of reading a file, the file may encounter problems unexpectedly, such as the file is occupied, the file does not exist, etc. Use a try-except block to avoid these problems and cause the program to crash. Here is an example of a try-except block that reads a file:
path = 'file.txt' try: with open(path, 'r') as f: # 读取文件内容 except FileNotFoundError: print('File not found!') except Exception as e: print('Error:', e)
This code block can catch FileNotFoundError and other exceptions. If the file cannot be found, the program will output an error message; if it encounters other exceptions, it will also output an error message and record the exception type.
- Use binary mode
In some cases, you may need to use binary mode when reading files, such as reading binary files such as images and sounds. When using binary mode, you need to use the 'b' identifier in the file opening mode, as shown below:
path = 'image.png' with open(path, 'rb') as f: # 读取二进制文件内容
Here'image.png' is the image file name, and 'rb' means opening in binary mode document. When reading a binary file, you can convert the read content into a byte array for continued processing.
- Avoid Hardcoding
When writing code, avoiding hardcoding file names and paths can make the code more flexible and maintainable. File paths can be specified using configuration files, command line parameters, etc. to make the code more versatile. The configuration file can contain multiple file paths, and the code can choose one of the paths to read according to the situation to avoid hard-coding problems.
- Avoid security issues
When reading a file, you may be attacked by malicious code in the file. For example, the files read may contain malicious scripts, viruses, etc. To avoid security issues, appropriate permissions should be used to restrict file reading, writing, and other operations. In addition, you can also use third-party libraries, such as PyPDF2, Pillow, python-docx, etc., to read specific types of files to avoid security risks caused by directly reading files.
Summary
In Python, file reading is a common operation. However, during the file reading process, code writers may make mistakes, resulting in non-standard reading methods, resulting in program crashes, time consuming, and even security risks. To avoid these problems, use absolute paths, check if the file exists, use with statements, use try-except blocks, use binary mode, avoid hardcoding, and avoid security issues. These methods can make the code more standardized, correct, maintainable and safe.
The above is the detailed content of How to solve the non-standard file reading error in Python code?. For more information, please follow other related articles on the PHP Chinese website!
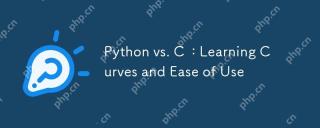
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
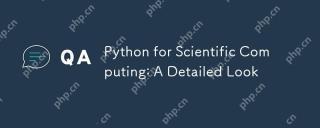
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
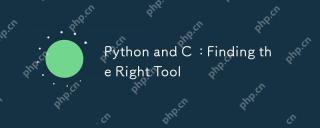
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
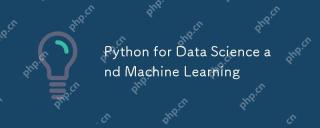
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
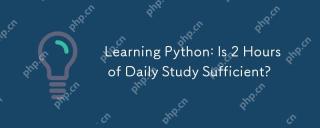
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
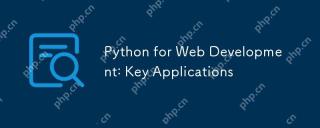
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
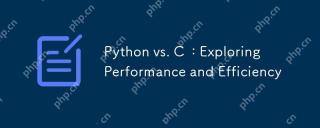
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
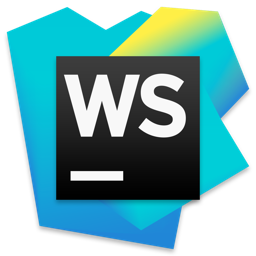
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.