With the development of the Internet, Web server caching has become one of the important means to improve the performance of Web applications. In previous development, we mainly used some specialized caching middleware such as Memcached and Redis. However, now we can use Golang's own concurrency library to implement web server caching to solve the performance problems of some small-scale applications. . This article will focus on how to use Golang to implement web server caching.
- Commonly used concurrency libraries in Golang
Golang has a wealth of concurrency libraries, including sync, channel, context and other commonly used libraries. Among them, the most commonly used ones are Mutex and RWMutex provided in the sync library. Mutex is the most basic kind of lock, which can only be held by one goroutine at the same time. Using it can ensure the security of multiple goroutines concurrently accessing shared resources. RWMutex is a read-write lock based on Mutex, which can limit the number of goroutines that read resources at the same time and improve concurrent reading efficiency.
- Steps to implement Web server cache
Next, we will follow the following steps to implement Web server cache:
2.1 Create a cache structure Body
We can use RWMutex in the sync library to implement a concurrently safe cache structure, in which map is used as the cache storage structure. The specific implementation is as follows:
type Cache struct { cache map[string]interface{} rw sync.RWMutex } func NewCache() *Cache { return &Cache{ cache: make(map[string]interface{}), } }
2.2 Implementation of cache operation functions
Implement the basic operation functions of cache, such as Get, Set, Delete, etc. Among them, we use RWMutex to ensure the security of cache concurrent access.
func (c *Cache) Get(key string) (interface{}, bool) { c.rw.RLock() defer c.rw.RUnlock() item, ok := c.cache[key] return item, ok } func (c *Cache) Set(key string, value interface{}) { c.rw.Lock() defer c.rw.Unlock() c.cache[key] = value } func (c *Cache) Delete(key string) { c.rw.Lock() defer c.rw.Unlock() delete(c.cache, key) }
2.3 Cache expiration processing
The data in the cache exists for different lengths of time. We can ensure the freshness of the cached data by regularly deleting it by setting the cache time. To do this, we need to use goroutine to periodically delete expired cache data.
func (c *Cache) deleteExpired() { for { time.Sleep(time.Second * 30) c.rw.Lock() for key, value := range c.cache { item, ok := value.(*item) if ok && item.expired.Before(time.Now()) { delete(c.cache, key) } } c.rw.Unlock() } } type item struct { data interface{} expired time.Time } func (c *Cache) SetWithExpiration(key string, value interface{}, expiration time.Duration) { item := &item{ data: value, expired: time.Now().Add(expiration), } c.rw.Lock() defer c.rw.Unlock() c.cache[key] = item } func NewCache() *Cache { c := &Cache{ cache: make(map[string]interface{}), } go c.deleteExpired() return c }
- Summary
This article introduces how to use Golang’s own concurrency library to implement web server caching. By implementing the basic operation functions of cache and goroutine that periodically deletes expired cache data, we can build a lightweight web server cache by ourselves to solve the performance problems of small-scale applications. At the same time, we can also learn the basic methods of using the Golang concurrency library, which will help us better leverage Golang's concurrency performance advantages in future development.
The above is the detailed content of How to use Golang to implement web server caching. For more information, please follow other related articles on the PHP Chinese website!
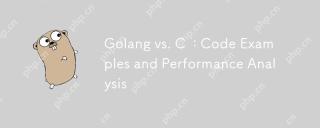
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
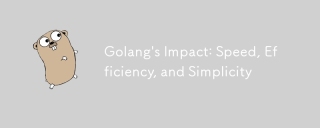
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
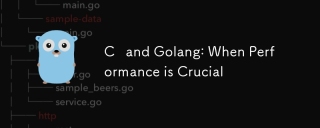
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
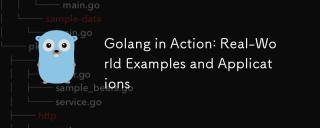
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
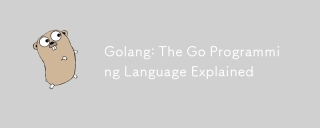
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
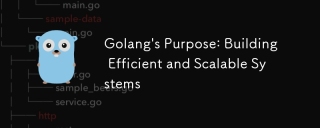
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
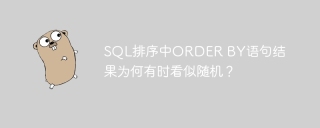
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
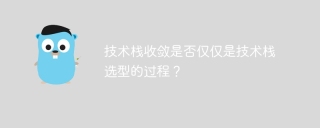
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
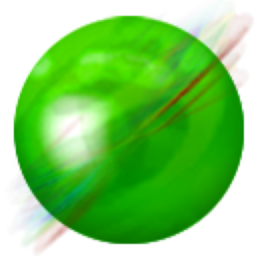
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
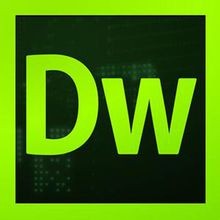
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version