


Golang is an efficient and powerful programming language that supports the use of regular expressions and can easily verify whether user input complies with specifications and security requirements. This article will introduce how to use regular expressions in Golang to verify whether the input is an attribute value in an HTML tag.
First, we need to understand the basic concepts of HTML tags and attributes. HTML is a markup language used to create web pages. It consists of various tags and attributes. Tags are used to describe the structure and content of the document, and attributes are used to describe the properties of the tags.
For example, the following is an example of a simple HTML tag and attribute:
<div class="container"></div>
Where, <div> is an HTML tag, <code>class
is its attribute name, container
is its attribute value. Before using regular expressions to verify whether the input is an attribute value in an HTML tag, we need to understand the basic usage of regular expressions.
Regular expressions in Golang are implemented using the regexp
package. Its basic usage is as follows:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile("hello") fmt.Println(re.MatchString("hello world")) // true fmt.Println(re.MatchString("world")) // false }
In the above example, use regexp.MustCompile
The function creates a regular expression object and then uses the MatchString
method to match the string. If the string match is successful, true
is returned, otherwise false
is returned.
When using regular expressions to verify whether the input is an attribute value in an HTML tag, we need to match the following two situations:
- The attribute value is quoted (single or double quotes ) surrounded.
- The attribute value is not surrounded by quotes.
For the first case, we can use the following regular expression:
"[^"]*"
This regular expression can match all strings surrounded by double quotes. [^"]*
means matching any number of non-double quote characters. Since double quotes are also special characters in regular expressions, they need to be escaped as "
. The complete sample code is as follows:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile(`"([^"]*)"`) fmt.Println(re.MatchString(`class="container"`)) // true fmt.Println(re.MatchString(`class='container'`)) // false fmt.Println(re.MatchString(`class=container`)) // false }
In the above example, using "
to create a regular expression object separately may cause parsing errors, so we use `
to Surrounded by regular expressions. Use the re.MatchString
method to verify whether the string matches the regular expression. If it matches, it returns true
, otherwise it returns false
.
For the second case, we need to use the following regular expression:
[^ "]+
This regular expression can match any number of non-space characters, that is, attribute values surrounded by non-quotes. In the regular expression In the expression, [^ ]
means matching any non-space character,
means matching one or more times. If the attribute value contains spaces, the match will not succeed. Complete sample code As follows:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile(`[^ "]+`) fmt.Println(re.MatchString(`class="container"`)) // false fmt.Println(re.MatchString(`class='container'`)) // false fmt.Println(re.MatchString(`class=container`)) // true }
When using regular expressions to verify input, you need to pay attention to the special characters and escape characters of regular expressions. When writing regular expressions, you can use online tools to test and debug them to Ensure the correctness and performance of regular expressions.
In summary, this article introduces how to use regular expressions in Golang to verify whether the input is an attribute value in an HTML tag. In actual development, you can use Appropriate regular expressions are required to validate user input to ensure the security and reliability of your application.
The above is the detailed content of Use regular expressions in golang to verify whether the input is an attribute value in the HTML tag. For more information, please follow other related articles on the PHP Chinese website!
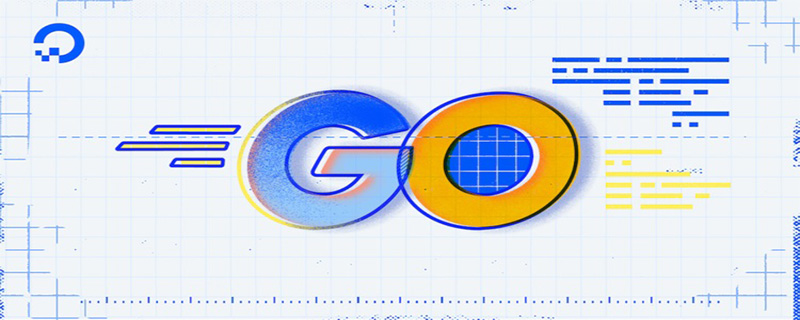
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
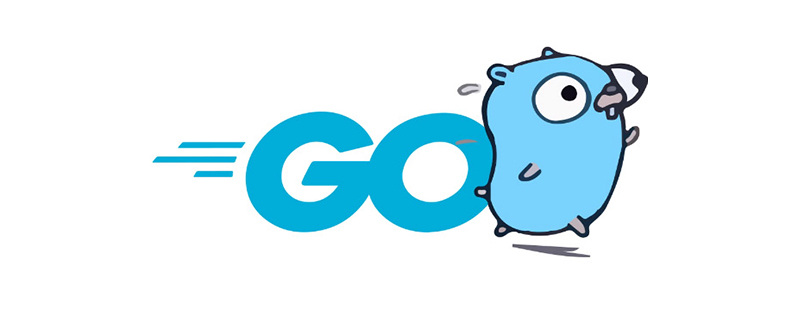
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
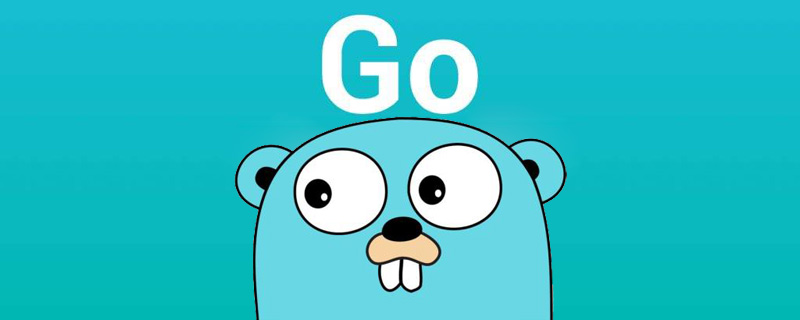
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
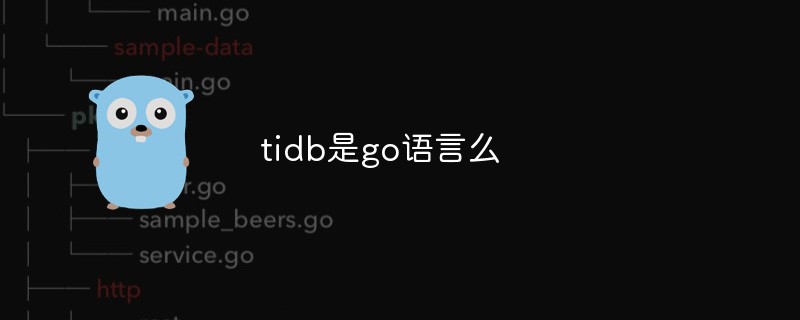
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
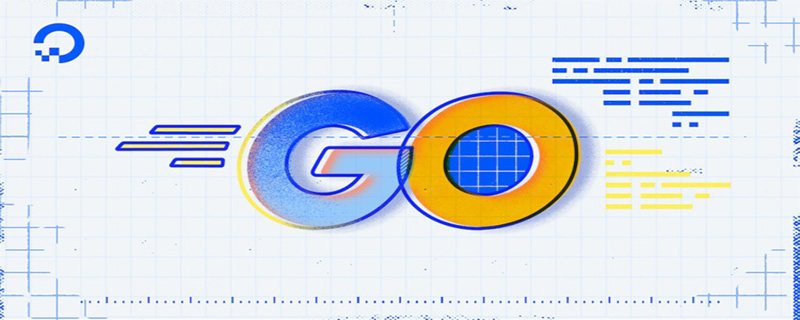
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
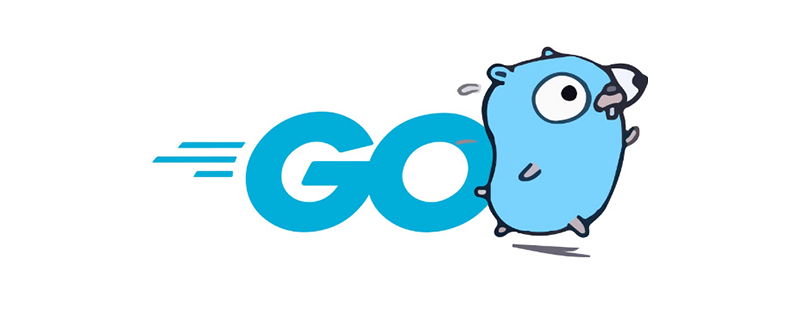
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
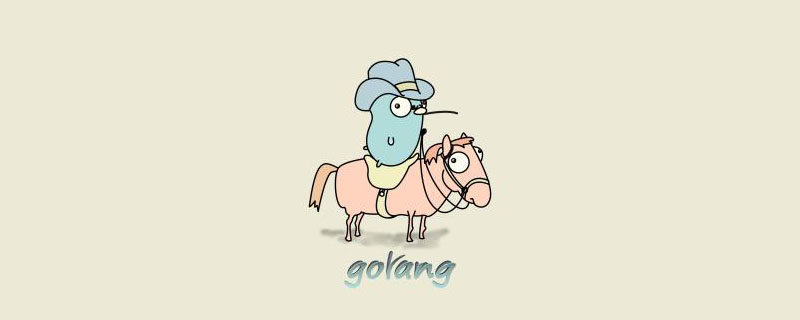
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
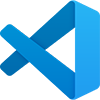
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
