Build web applications using Golang's web framework Echo
In today's Internet era, Web applications have become an indispensable part of people's daily lives. Web applications are applications accessed through a browser, which allow users to complete most tasks in the browser. Building web applications often requires the use of web frameworks, which can help us quickly develop applications and reduce repetitive code writing. In this article, we will introduce how to use the Echo framework in Golang to build web applications.
1. Introduction to Echo Framework
Echo is a high-performance, lightweight Web framework based on the Golang language and has the characteristics of simplicity, ease of use, and high performance. The characteristics of the Echo framework are as follows:
1. Simple and easy to use: The Echo framework provides a simple, easy-to-use way to handle HTTP requests and responses.
2. High performance: The Echo framework has excellent performance, can handle a large number of requests and responses, and also supports concurrent processing.
3. Routing: The Echo framework provides routing functionality to easily route URLs and handle HTTP requests.
2. Install the Echo framework
The Echo framework can be installed through the go command:
go get -u github.com/labstack/echo/...
The above command will get the latest version of the Echo framework from the GitHub repository so that you can Used in local development environment.
3. Build a simple web application
Before we start building a web application with the Echo framework, we need to understand some concepts first.
Routing: Routing is a mechanism in web applications that is used to specify the mapping between URLs and handlers. Routes can be easily built using the Echo framework.
Handler: A handler refers to a block of code used in a web application to handle a specific HTTP request. It can be a function, method, class, etc.
Before we start building a simple web application, we need to follow the following steps to set it up:
1. Import the Echo framework
import "github.com/labstack/echo"
2. Create an Echo instance
e := echo.New()
3. Define the handler
func hello(c echo.Context) error { return c.String(http.StatusOK, "Hello, World!") }
4. Use the Echo framework to create a route and point it to the handler
e.GET("/", hello)
Now that we have completed the basic setup of the web application, here is the complete Code:
package main import ( "net/http" "github.com/labstack/echo" ) func hello(c echo.Context) error { return c.String(http.StatusOK, "Hello, World!") } func main() { e := echo.New() e.GET("/", hello) e.Logger.Fatal(e.Start(":8000")) }
In the above code, we have defined a handler called hello and created a route using the Echo framework to point it to the handler.
Finally, we use Logger to start the Echo framework and bind it to port 8000. Now, we can launch the application and visit http://localhost:8000 in the browser and see the "Hello, World!" output.
4. Add middleware
Middleware is the code block between HTTP requests and responses. They can be used to log requests, validate requests, response formatting, etc.
In the Echo framework, we can use a single middleware or a stack of middleware to handle requests. Here is an example of how to add middleware in the Echo framework:
e := echo.New() //单个中间件 e.Use(middleware.Logger()) //多个中间件 e.Use(middleware.Logger(), middleware.Recover())
The above example adds Echo's Logger and Recover middleware to the application. Logger middleware is used to record HTTP requests and responses, while Recover middleware is used to handle any errors that occur during HTTP requests.
5. Conclusion
The Echo framework is a high-performance, lightweight Web framework, which is implemented based on the Golang language. Using the Echo framework allows us to easily build web applications and also add middleware to handle HTTP requests. Through the introduction of this article, you have learned how to use the Echo framework to build web applications in Golang.
The above is the detailed content of Build web applications using Golang's web framework Echo. For more information, please follow other related articles on the PHP Chinese website!
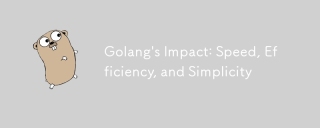
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
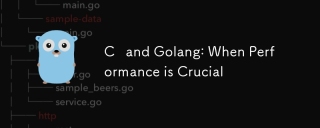
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
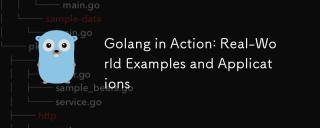
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
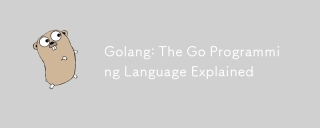
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
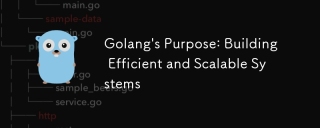
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
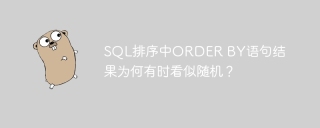
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
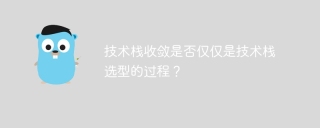
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
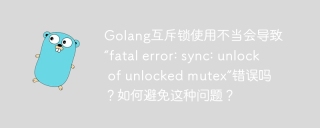
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
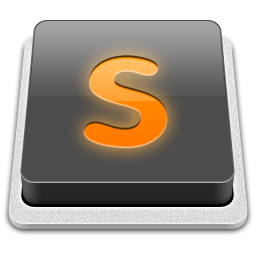
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
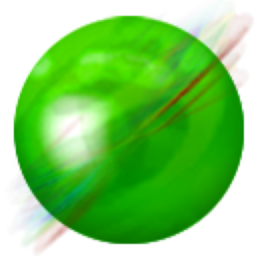
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment