Using WebSocket for real-time communication in Beego
In today's Internet era, real-time communication has become an indispensable function in many application scenarios. From online chat, real-time gaming to surveillance systems, real-time communication is in increasing demand. The most common way to achieve real-time communication is to use the WebSocket protocol.
The WebSocket protocol is a new network communication protocol in the HTML5 specification. It can provide a full-duplex communication channel on a TCP connection. Real-time communication functions can be easily achieved using the WebSocket protocol, and Beego, as a fast and efficient Web framework, naturally supports the WebSocket protocol.
This article will introduce the process of using WebSocket to achieve real-time communication in Beego. First, we need to install the Beego framework:
go get github.com/astaxie/beego
After the installation is complete, we can start using the Beego framework. Before using Beego to implement WebSocket, we need to first understand the WebSocket protocol. The WebSocket protocol has three phases: handshake, data transfer and closure.
In the handshake phase, the client sends an HTTP request to the server, requesting an upgrade to the WebSocket protocol. After receiving the request, the server returns a 101 Switching Protocols response. At this time, the WebSocket connection has been established. During the data transfer phase, the client and server can transfer data to each other. During the shutdown phase, the client or server can send a shutdown frame to close the WebSocket connection.
In Beego, we can implement the three stages of WebSocket by implementing the WebSocket function of beego.Controller.
First, we need to create a WebSocket controller:
package controllers import ( "github.com/astaxie/beego" "github.com/gorilla/websocket" ) type WebSocketController struct { beego.Controller } var upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, } func (this *WebSocketController) Get() { conn, err := upgrader.Upgrade(this.Ctx.ResponseWriter, this.Ctx.Request, nil) if err != nil { beego.Error("WebSocket协议升级失败:", err) return } // 处理连接 }
In the above code, we created an Upgrader through the gorilla/websocket package and called the Upgrader's Upgrade in the Get method Method upgrade protocol. At this point, the WebSocket connection has been successfully established. Next, we need to handle the WebSocket connection.
For the processing of WebSocket connections, we can use goroutine. Whenever there is a new connection, we open a new goroutine to handle the connection:
func (this *WebSocketController) Get() { conn, err := upgrader.Upgrade(this.Ctx.ResponseWriter, this.Ctx.Request, nil) if err != nil { beego.Error("WebSocket协议升级失败:", err) return } go this.handleConnection(conn) } func (this *WebSocketController) handleConnection(conn *websocket.Conn) { defer conn.Close() // 处理连接 }
In the method of processing the connection, we can use a for loop to continuously read the data from the client:
func (this *WebSocketController) handleConnection(conn *websocket.Conn) { defer conn.Close() for { _, msg, err := conn.ReadMessage() if err != nil { beego.Error("读取消息失败:", err) return } // 处理消息 } }
In the method of processing messages, we can perform corresponding processing based on the data sent by the client, and send the processing results back to the client:
func (this *WebSocketController) handleConnection(conn *websocket.Conn) { defer conn.Close() for { _, msg, err := conn.ReadMessage() if err != nil { beego.Error("读取消息失败:", err) return } // 处理消息 reply := "收到消息:" + string(msg) err = conn.WriteMessage(websocket.TextMessage, []byte(reply)) if err != nil { beego.Error("发送消息失败:", err) return } } }
The above code implements a simple The WebSocket echo server directly returns the data as it is after receiving the data from the client. In practical applications, we can perform corresponding data processing as needed and push the processing results to the client in real time.
Finally, we need to register the WebSocket controller in the routing:
beego.Router("/websocket", &controllers.WebSocketController{})
At this point, we have completed the process of using WebSocket to achieve real-time communication in the Beego framework. It should be noted that Beego uses the gorilla/websocket package to implement the WebSocket function, so this package needs to be imported into the project.
In summary, Beego is a powerful and easy-to-use Web framework that can easily realize real-time communication functions when combined with the WebSocket protocol. When using WebSocket to implement real-time communication, we need to pay attention to the three phases of the protocol and use goroutine for processing.
The above is the detailed content of Using WebSocket for real-time communication in Beego. For more information, please follow other related articles on the PHP Chinese website!
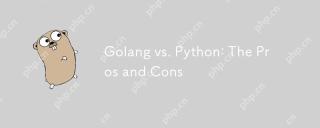
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
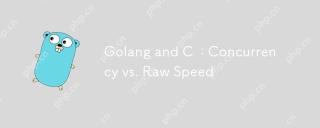
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
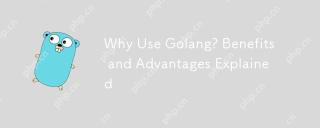
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
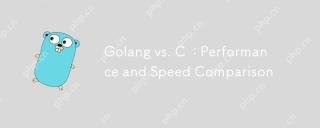
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
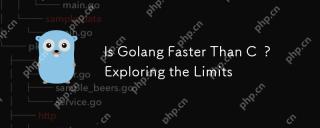
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
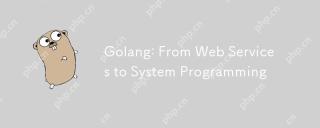
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
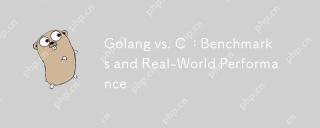
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
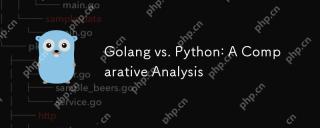
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
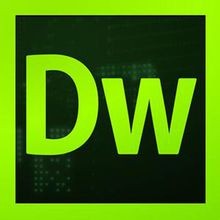
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
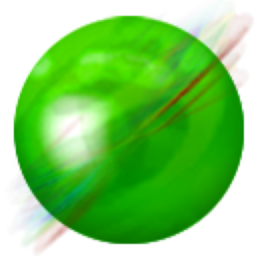
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment