Using GraphQL for API development in Beego
GraphQL is a modern API query language developed by Facebook that provides a more efficient and flexible way to build APIs. Different from traditional RESTful API, GraphQL allows the client to define the data it needs, and the server only returns the data requested by the client, thus reducing unnecessary data transmission.
Beego is an open source web framework written in Go language. It provides a series of tools and libraries to quickly develop high-performance web applications. Beego's built-in ORM and template engine make it very convenient and efficient when developing web applications.
In this article, we will introduce how to use GraphQL for API development in Beego.
- Installing GraphQL-go
First, we need to install GraphQL-go (a GraphQL server library implemented in Go language).
Install using the following command:
go get github.com/graphql-go/graphql
go get github.com/graphql-go/handler
- Create GraphQL Schema
When using GraphQL in Beego, we need to define a Schema to describe the structure of the data. Schema consists of types, properties and methods.
The following is a simple User type definition:
var userType = graphql.NewObject( graphql.ObjectConfig{ Name: "User", Fields: graphql.Fields{ "id": &graphql.Field{ Type: graphql.NewNonNull(graphql.Int), }, "name": &graphql.Field{ Type: graphql.String, }, "email": &graphql.Field{ Type: graphql.String, }, }, }, )
The above code defines a User type and contains three attributes: id, name and email. Among them, the id attribute is a non-empty integer type, and the name and email attributes are string types.
We can also define a query method containing multiple Users:
var rootQuery = graphql.NewObject( graphql.ObjectConfig{ Name: "Query", Fields: graphql.Fields{ "users": &graphql.Field{ Type: graphql.NewList(userType), Resolve: func(p graphql.ResolveParams) (interface{}, error) { return getUsers() }, }, }, }, ) func getUsers() ([]User, error) { // 获取用户数据 }
The above code defines a query method named users, which will return a list containing multiple Users. In the Resolve method, we will call the getUsers method to obtain user data and return it to the client. Since GraphQL-go provides the function of automatically parsing Graphql parameters, we do not need to manually obtain the request parameters.
- Create GraphQL Handler
Next, we need to bind the GraphQL Schema with Beego and create a GraphQL handler.
We can use the custom HTTP handler provided by GraphQL-go for processing. Here is a simple example:
h := handler.New(&handler.Config{ Schema: &graphql.Schema{Query: rootQuery}, Pretty: true, GraphiQL: true, })
The above code creates a GraphQL handler h that uses the Schema we defined (rootQuery), has GraphiQL (Web IDE for GraphQL) and code formatting features enabled.
- Register GraphQL Handler
Finally, we register the GraphQL Handler we just created in Beego.
The following is the sample code:
beego.Handler("/graphql", h)
The above code binds the GraphQL handler h to the route "/graphql". Now, visit "/graphql" in your browser to open GraphiQL and start testing the API.
Summary
In this article, we introduced how to use GraphQL for API development in Beego. First, we installed GraphQL-go and created a GraphQL Schema, then used the custom HTTP handler provided by GraphQL-go to handle the request, and finally registered the GraphQL handler into the Beego route.
The flexibility and efficiency of GraphQL make it one of the most popular API development methods today. Combining Beego's high performance and easy-to-use features, we can quickly build web applications with excellent performance.
The above is the detailed content of Using GraphQL for API development in Beego. For more information, please follow other related articles on the PHP Chinese website!
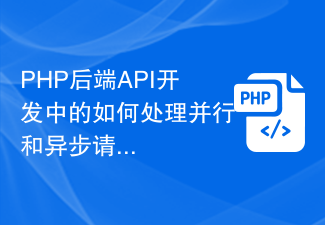
随着网络应用的不断发展和变化,处理并行和异步请求已经成为PHP后端API开发中的一个重要主题。在传统的PHP应用中,请求是同步进行的,即一个请求在收到响应之前会一直等待,这会影响应用的响应速度和性能。但是,PHP现在已经拥有了并行和异步请求处理的能力,这些功能让我们可以更好地处理大量并发请求,提高应用的响应速度和性能。本文将讨论PHP后端API开发中的如何处
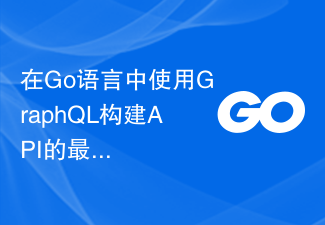
随着前后端分离的趋势越来越普及,API的设计和使用也变得越来越重要。在Go语言中使用GraphQL构建API是一种流行的选择,因为GraphQL允许前端开发人员按照自己的需求从后端获取数据。然而,GraphQL具有独特的设计和属性,开发人员需要遵循一些最佳实践才能确保良好的性能和可维护性。以下是在Go语言中使用GraphQL构建API的最佳实践:定义Grap
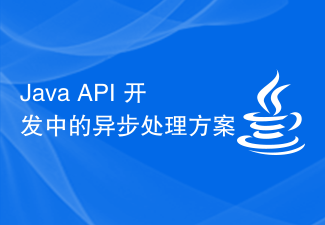
随着Java技术的不断发展,JavaAPI已经成为许多企业开发的主流方案之一。在JavaAPI开发过程中,常常需要对大量的请求和数据进行处理,但是传统的同步处理方式无法满足高并发、高吞吐量的需求。因此,异步处理成为了JavaAPI开发中的重要解决方案之一。本文将介绍JavaAPI开发中常用的异步处理方案及其使用方法。一、Java异
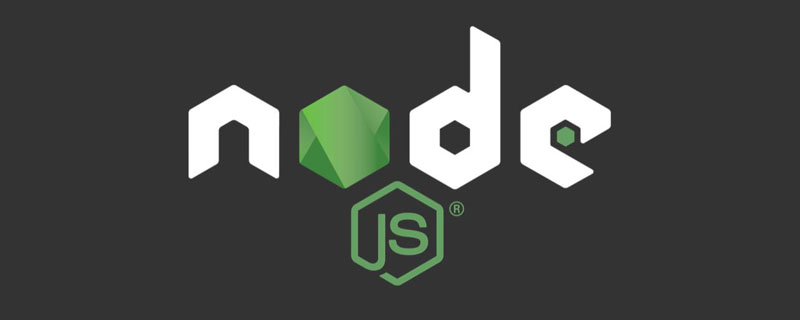
如何使用 Node.js 进行前端应用的开发?下面本篇文章给大家介绍一下Node开发前端应用的方法,涉及到表现层应用的开发。我今天分享的方案是针对简单场景的,旨在让前端开发人员不必掌握太多关于 Node.js 的背景知识和专业知识,即使没有代码编写经验,也能完成一些简单的服务端开发任务。
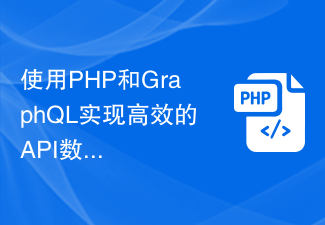
随着互联网技术的不断发展,API已经成为了各种软件之间相互交流的重要方式。API能够提供统一的数据接口,使得不同的软件可以互相访问和使用。然而,随着API的数量和规模的不断增大,如何快速高效地处理API数据的查询和操作成为了重要的问题。在这个问题上,PHP和GraphQL可以提供一种非常有效的解决方案。本文将针对这个解决方案进行一些简单的介绍和分析。PHP概

GraphQL 是 API 的开发人员和消费者以及他们背后的组织的强大推动者。GraphQL 实现的所有细节和功能都在 GraphQL Schema 中列出。
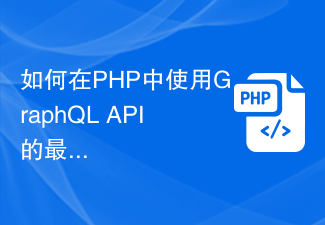
GraphQL是一个强大的API查询语言,可以极大地简化数据获取和操作过程。PHP作为一种广泛使用和支持的编程语言,也可以轻松地使用GraphQLAPI来访问各种数据源。但是,对于PHP开发人员来说,如何在PHP应用程序中使用GraphQLAPI的最佳实践方法还是需要一些指导。在本文中,我们将深入探讨如何在PHP中使用GraphQLAPI。1.安装和配
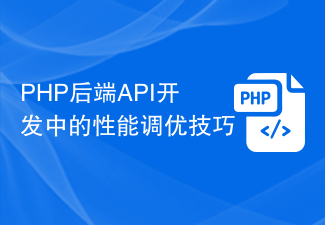
随着互联网的快速发展,越来越多的应用程序采用了Web架构,而PHP作为一种广泛应用于Web开发中的脚本语言,也日益受到了广泛的关注与应用。随着业务的不断发展与扩展,PHPWeb应用程序的性能问题也逐渐暴露出来,如何进行性能调优已成为PHPWeb开发人员不得不面临的一项重要挑战。接下来,本文将介绍PHP后端API开发中的性能调优技巧,帮助PHP开发人员更好


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
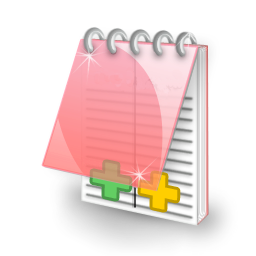
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
