


In modern enterprise applications, data analysis and visualization are crucial functions. Data helps us understand the actual situation of business operations and customer needs, and visualization allows us to understand and display the data more intuitively. In this article, we will introduce how to use the Gin framework to implement data analysis and visualization functions.
Gin is a web framework written in Go language. It is a framework that is lightweight, efficient, easy to learn and use, and therefore is increasingly popular in enterprise-level applications. We can use Gin to develop web applications, such as data display platforms, API servers, etc. At the same time, the Gin framework provides many powerful functions, such as routing, middleware, etc., which can be used to develop various applications.
Below we will introduce how to use the Gin framework to implement data analysis and visualization.
- Developing Web applications using the Gin framework
First, we need to use Gin to develop Web applications. For this we need to install the Gin library. Gin can be installed in the terminal with the following command:
go get -u github.com/gin-gonic/gin
Once the installation is complete, we can start writing our application. The following is a simple example:
package main import "github.com/gin-gonic/gin" func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello World!", }) }) r.Run(":8080") }
The above code creates a route named "/". When the user accesses the route, a JSON response containing "Hello World!" will be returned. information.
- Connect to the database
In order to perform data analysis, we need to obtain data from the database. We can use the database/sql package provided by Go to connect to our database and execute queries. Here is an example:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func connectToDB() (*sql.DB, error) { db, err := sql.Open("mysql", "user:password@/database") if err != nil { return nil, err } err = db.Ping() if err != nil { return nil, err } return db, nil } func getDataFromDB(db *sql.DB) ([]Data, error) { rows, err := db.Query("SELECT * FROM data") if err != nil { return nil, err } defer rows.Close() var data []Data for rows.Next() { var d Data err := rows.Scan(&d.Field1, &d.Field2, &d.Field3) if err != nil { return nil, err } data = append(data, d) } return data, nil }
The above code snippet will connect to a MySQL database, get data from the data table "data", and then store it in a struct slice.
- Data Visualization
Once we have the data from the database, we need to visualize it. We can create visualization charts using Data Visualization API (D3.js). Here is an example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>D3.js Example</title> </head> <body> <svg width="500" height="300"></svg> <script src="https://d3js.org/d3.v5.min.js"></script> <script> d3.csv("data.csv", function(data) { var svg = d3.select("svg"); var xScale = d3.scaleLinear() .domain([0, d3.max(data, function(d) { return +d.x; })]) .range([0, 500]); var yScale = d3.scaleLinear() .domain([0, d3.max(data, function(d) { return +d.y; })]) .range([300, 0]); var line = d3.line() .x(function(d) { return xScale(+d.x); }) .y(function(d) { return yScale(+d.y); }); svg.append("path") .datum(data) .attr("d", line) .attr("fill", "none") .attr("stroke", "steelblue") .attr("stroke-width", 2); }); </script> </body> </html>
The above code will read the data from a CSV file and then plot it into a simple line chart.
- Combined with the Gin framework
Now we have seen how to use Gin to develop web applications, how to connect to the database and how to use D3.js for data visualization. Finally we need to put this together.
The following is a sample code that will get data from a MySQL database, convert it to JSON format, and then pass it to the front end for visualization.
package main import ( "database/sql" "encoding/json" "log" "net/http" "github.com/gin-gonic/gin" ) type Data struct { Field1 string `json:"field1"` Field2 string `json:"field2"` Field3 int `json:"field3"` } func getDataFromDB(db *sql.DB) ([]Data, error) { rows, err := db.Query("SELECT * FROM data") if err != nil { return nil, err } defer rows.Close() var data []Data for rows.Next() { var d Data err := rows.Scan(&d.Field1, &d.Field2, &d.Field3) if err != nil { return nil, err } data = append(data, d) } return data, nil } func main() { db, err := sql.Open("mysql", "user:password@/database") if err != nil { log.Fatal(err) } defer db.Close() r := gin.Default() r.GET("/", func(c *gin.Context) { data, err := getDataFromDB(db) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } jsonData, err := json.Marshal(data) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } c.HTML(http.StatusOK, "index.tmpl", gin.H{ "title": "Data Visualization", "data": string(jsonData), }) }) r.Run(":8080") }
This code will get the data from the database and convert it into JSON format. The JSON data is then passed back to the front end for drawing visualization charts.
Summary
In this article, we introduced how to use the Gin framework to implement data analysis and visualization functions. We can use Gin to develop web applications, use the database/sql package to connect to the database and execute queries, and use D3.js to draw visual charts. Combining these, we can use data analytics and visualization to better understand our business operations and customer needs.
The above is the detailed content of Use the Gin framework to implement data analysis and visualization functions. For more information, please follow other related articles on the PHP Chinese website!
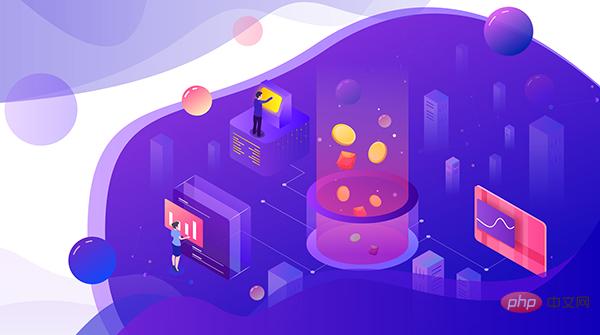
介绍编程和技术应用于金融领域的激增是不可避免的,增长似乎从未下降。应用编程的最有趣的部分之一是历史或实时股票数据的解释和可视化。现在,为了在python中可视化一般数据,matplotlib、seaborn等模块开始发挥作用,但是,当谈到可视化财务数据时,Plotly将成为首选,因为它提供了具有交互式视觉效果的内置函数。在这里我想介绍一个无名英雄,它只不过是mplfinance库matplotlib的兄弟库。我们都知道matplotlib包的多功能性,并且可以方便地绘制任何类型的数据。
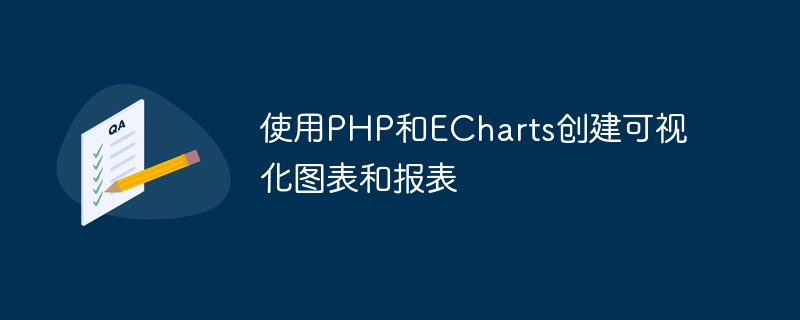
随着大数据时代的来临,数据可视化成为企业决策的重要工具。千奇百怪的数据可视化工具层出不穷,其中ECharts以其强大的功能和良好的用户体验受到了广泛的关注和应用。而PHP作为一种主流的服务器端语言,也提供了丰富的数据处理和图表展示功能。本文将介绍如何使用PHP和ECharts创建可视化图表和报表。ECharts简介ECharts是一个开源的可视化图表库,它由
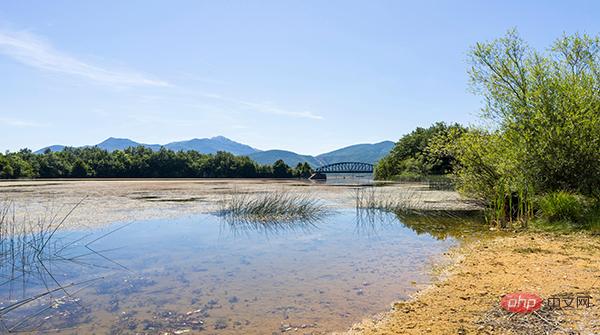
一、简介Plotly是一个非常著名且强大的开源数据可视化框架,它通过构建基于浏览器显示的web形式的可交互图表来展示信息,可创建多达数十种精美的图表和地图。二、绘图语法规则2.1离线绘图方式Plotly中绘制图像有在线和离线两种方式,因为在线绘图需要注册账号获取APIkey,较为麻烦,所以本文仅介绍离线绘图的方式。离线绘图又有plotly.offline.plot()和plotly.offline.iplot()两种方法,前者是以离线的方式在当前工作目录下生成html格式的图像文件,并自动打开;
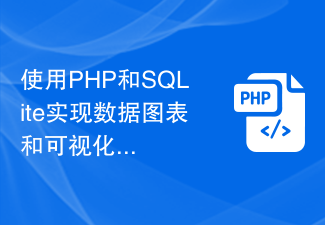
使用PHP和SQLite实现数据图表和可视化概述:随着大数据时代的到来,数据图表和可视化成为了展示和分析数据的重要方式。在本文中,将介绍如何使用PHP和SQLite实现数据图表和可视化的功能。以一个实例为例,展示如何从SQLite数据库中读取数据,并使用常见的数据图表库来展示数据。准备工作:首先,需要确保已经安装了PHP和SQLite数据库。如果没有安装,可
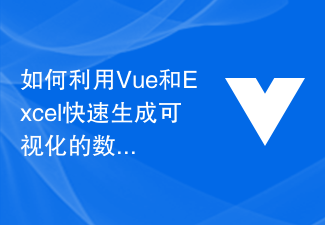
如何利用Vue和Excel快速生成可视化的数据报告随着大数据时代的到来,数据报告成为了企业决策中不可或缺的一部分。然而,传统的数据报告制作方式繁琐而低效,因此,我们需要一种更加便捷的方法来生成可视化的数据报告。本文将介绍如何利用Vue框架和Excel表格来快速生成可视化的数据报告,并附上相应的代码示例。首先,我们需要创建一个基于Vue的项目。可以使用Vue
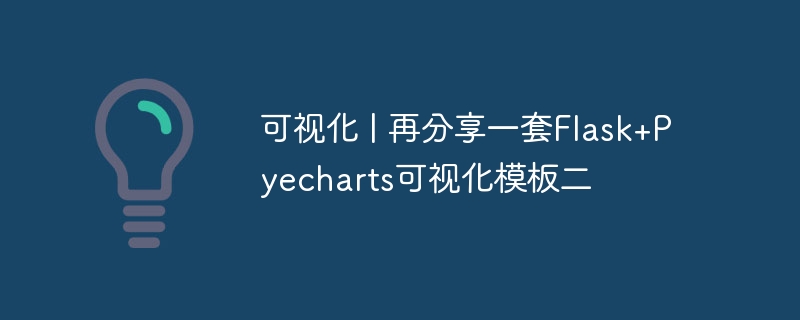
本期再给大家分享一套适合初学者的<Flask+Pyecharts可视化模板二>,希望对你有所帮助
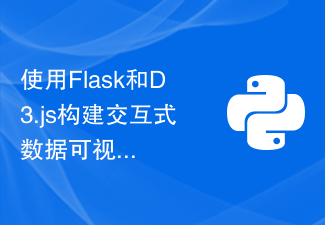
近年来,数据分析和数据可视化已经成为了许多行业和领域中不可或缺的技能。对于数据分析师和研究人员来说,将大量的数据呈现在用户面前并且让用户能够通过可视化手段来了解数据的含义和特征,是非常重要的。为了满足这种需求,在Web应用程序中使用D3.js来构建交互式数据可视化已经成为了一种趋势。在本文中,我们将介绍如何使用Flask和D3.js构建交互式数据可视化Web
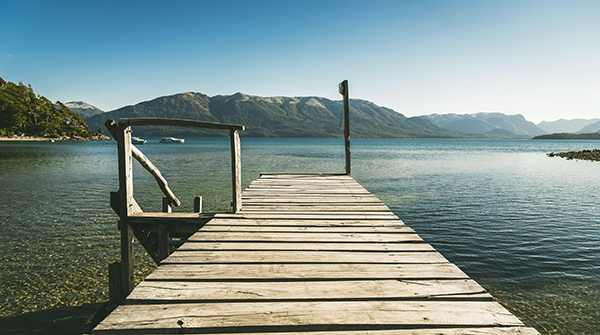
关于界面的大致模样其实和先前的相差不大,大家应该都看过上一篇的内容。界面大体的样子整体GUI的界面如下图所示:用户在使用的时候可以选择将证件照片替换成是“白底背景”或者是“红底背景”,那么在前端的界面上传完成照片之后,后端的程序便会开始执行该有的操作。去除掉背景颜色首先我们需要将照片的背景颜色给去除掉,这里用到的是第三方的接口removebg,官方链接是:我们在完成账号的注册之后,访问下面的链接获取api_key:https://www.remove.bg/api#remove-backgrou


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
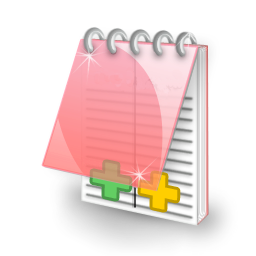
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
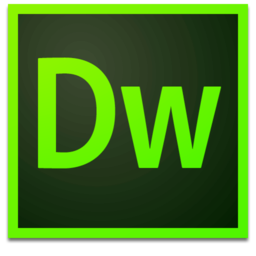
Dreamweaver Mac version
Visual web development tools
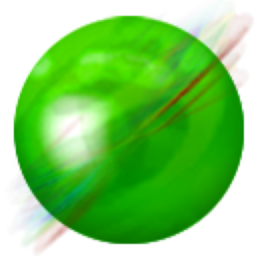
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
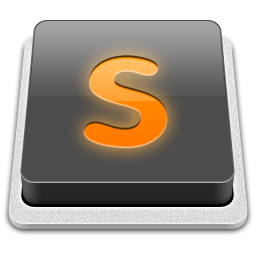
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
