As modern enterprises continue to evolve, efficient asynchronous messaging has become critical. In this case, message queue is a reliable and scalable solution that can help developers communicate between different systems. In this article, we will introduce how to implement message queue using NATS in Beego.
What is NATS
NATS is an open source, lightweight, fast messaging system that can be used to communicate across a variety of environments. It is a high-performance messaging system that can be used for simple point-to-point communication, publish-subscribe patterns, and queues.
The bottom layer of NATS is based on the TCP/IP protocol, and the language used is the Go language. It provides some basic messaging functions such as persistence, backup and failover.
Using NATS in Beego
NATS is a lightweight cross-language messaging system that can be seamlessly integrated with many back-end frameworks. Here we will introduce how to use NATS to implement message queues in Beego.
Step 1: Install NATS client
To use the NATS messaging system, we need to install the corresponding client. You can use the command line interface tool of the Go language to complete the installation through the following command:
go get github.com/nats-io/nats.go
Step 2: Establish a connection
Establishing a connection is the first step in using the NATS client library. A new NATS connection can be created by the following code:
nc, err := nats.Connect("nats://localhost:4222") if err != nil { log.Fatal(err) } defer nc.Close()
Step 3: Send the message
After the connection is established, we can send the message. Messages can be sent to the specified topic through the following code:
err := nc.Publish("subject", []byte("message")) if err != nil { log.Fatal(err) }
Step 4: Receive the message
Receiving the message requires subscribing to a specified topic. You can use the following code to subscribe:
_, err := nc.Subscribe("subject", func(m *nats.Msg) { log.Printf("Received a message: %s ", string(m.Data)) }) if err != nil { log.Fatal(err) }
Step 5: Process the message
After receiving the message, we can process it. This requires creating a handler function that will receive messages on the subscribed topic and then perform the specified action. For example:
func handleMsg(msg []byte) { fmt.Printf("Received message: %s", string(msg)) }
Step 6: Using NATS in Beego
Now that we know how to use NATS, how do we apply it in Beego? The simple way is to create a Controller and establish a connection to NATS, and then delegate the tasks of subscribing and processing messages to the corresponding methods. For example:
package controllers import ( "github.com/beego/beego/v2/server/web" "github.com/nats-io/nats.go" ) type MessageController struct { web.Controller nc *nats.Conn } func (this *MessageController) Prepare() { this.nc, _ = nats.Connect("nats://localhost:4222") } func (this *MessageController) Get() { this.TplName = "message.tpl" } func (this *MessageController) Post() { text := this.GetString("text") err := this.nc.Publish("subject", []byte(text)) if err != nil { this.Abort("500") } this.Redirect("/", 302) } func (this *MessageController) WebSocket() { this.TplName = "websocket.tpl" _, err := this.nc.Subscribe("subject", func(m *nats.Msg) { this.Data["text"] = string(m.Data) this.Render() }) if err != nil { this.Abort("500") } }
In this example, we define a Controller named MessageController. It has three methods: Get, Post and WebSocket.
The Get method is a simple HTTP GET request handler used to display a message page containing a text box and submit button.
The Post method is an HTTP POST request handler used to send the text in the text box to NATS.
The WebSocket method is an HTTP request handler upgraded to the WebSocket protocol. It subscribes to a specified topic and then receives messages on the WebSocket and presents them to the client.
Summary
In this article, we learned about the NATS messaging system and how to use it in Beego to implement asynchronous messaging. By using NATS, we can easily decouple various systems and achieve reliable asynchronous communication, which is very important for modern enterprises. We hope this article was helpful and helped you understand how to implement a message queue using NATS in Beego.
The above is the detailed content of Implement message queue using NATS in Beego. For more information, please follow other related articles on the PHP Chinese website!
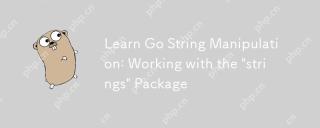
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
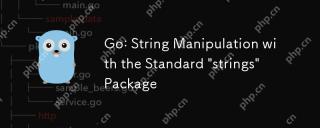
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
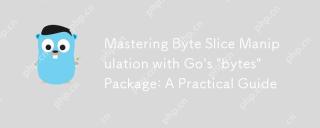
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
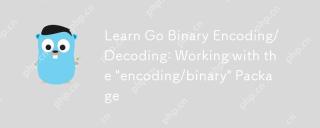
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
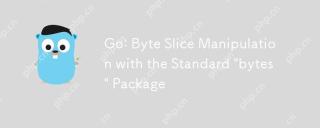
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
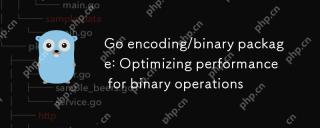
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
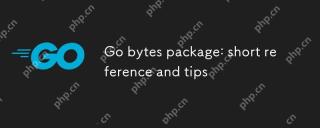
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
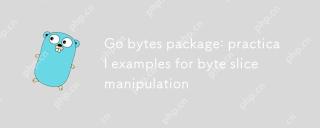
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
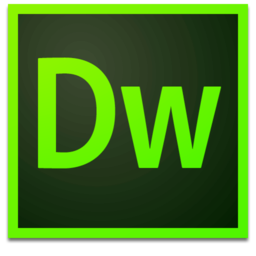
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
