


With the development of the Internet, more and more companies are beginning to adopt microservice architecture to build distributed systems to improve application reliability, scalability, maintainability and other capabilities. Under the microservice architecture, a task scheduling system is a very important component. It can be used to regularly execute some asynchronous tasks, call other microservice interfaces, etc.
Spring Cloud is an open source microservice framework that provides some very powerful tools and frameworks, such as Spring Cloud Netflix, Spring Cloud Config, Spring Cloud Stream, Spring Cloud Security, etc. Among them, Spring Cloud Netflix is a very popular microservice framework. It provides some core components and extensions, such as service registration and discovery, client load balancing, configuration management, circuit breakers, API gateways, etc.
In this article, we will introduce how to use Spring Cloud to develop a task scheduling system, which mainly includes the following content:
- Requirements analysis of task scheduling system
- Usage Spring Scheduler implements task scheduling
- Put the task scheduling system into the Spring Cloud microservice architecture
1. Requirements analysis of the task scheduling system
In the microservice architecture Under the circumstances, a task scheduling system needs to support the following functions:
- can trigger the execution of a task at a specified time point or moment.
- Supports triggering the execution of a task at fixed time intervals or periodic time intervals.
- Support retry after task execution failure.
- Supports asynchronous execution of tasks and does not affect the running of the main program.
- Supports dynamic addition and deletion of tasks.
2. Use Spring Scheduler to implement task scheduling
Spring Scheduler is a module of the Spring framework. It provides a lightweight task scheduling framework that can easily implement task scheduling. function.
- Add Spring Scheduler dependency
Add Spring Scheduler dependency in the pom.xml file of the Spring Boot project:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> <version>2.4.3</version> </dependency>
- Create task Execution class
Create a task execution class inherited from the Runnable interface to implement specific task logic.
@Component public class JobTask implements Runnable { @Override public void run() { System.out.println("running job..."); } }
- Add task scheduling configuration
Add a task scheduling configuration class to the Spring Boot project to configure the specific task scheduling strategy and executed tasks.
@Configuration @EnableScheduling public class ScheduleConfig { @Autowired private JobTask jobTask; //在每分钟的3秒和6秒执行一次 @Scheduled(cron = "3-6 * * * * ?") public void scheduleJob1() { jobTask.run(); } //在间隔5秒后执行第一次,之后每隔10秒执行一次 @Scheduled(initialDelay = 5000, fixedRate = 10000) public void scheduleJob2() { jobTask.run(); } }
- Test task scheduling function
After starting the Spring Boot application, you can view the console output and the task will be executed according to the specified time interval and periodicity. If you want to modify the task execution strategy, you only need to modify the configuration class.
3. Put the task scheduling system into the Spring Cloud microservice architecture
- Register the task scheduling system to the service registration center
In task scheduling In the system application, service registration and discovery components such as Spring Cloud's Eureka or Consul are used to register the task scheduling system to the service registration center.
spring: application: name: task-scheduler eureka: instance: hostname: localhost client: service-url: defaultZone: http://localhost:8761/eureka/
- Set routing rules in the gateway
Use API gateways such as Spring Cloud Gateway or Zuul to expose the task scheduling system to other microservices.
spring: application: name: api-gateway server: port: 8080 eureka: instance: hostname: localhost client: service-url: defaultZone: http://localhost:8761/eureka/ gateway: routes: - id: task-scheduler uri: lb://task-scheduler predicates: - Path=/schedule/**
- Call the task scheduling system in microservices
In other microservices, use tools such as Feign or RestTemplate to call the RESTful API exposed by the task scheduling system. Execute asynchronous tasks or trigger the execution of tasks.
@Service public class OrderService { @Autowired private TaskSchedulerClient taskSchedulerClient; public void createOrder(Order order) { //... 创建订单逻辑 taskSchedulerClient.scheduleJob(); } }
4. Summary
This article introduces how to use Spring Cloud to develop a task scheduling system under a microservice architecture, mainly including using Spring Scheduler to implement task scheduling and putting the task scheduling system into Spring Cloud In microservice architecture. I hope it will be helpful to developers who are adopting microservice architecture, so that they can more easily build highly reliable and highly scalable distributed systems.
The above is the detailed content of How to use Spring Cloud to develop a task scheduling system under a microservice architecture. For more information, please follow other related articles on the PHP Chinese website!
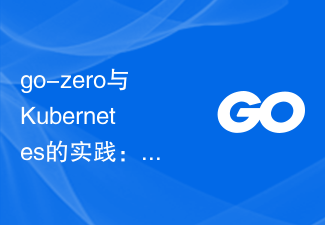
随着互联网规模的不断扩大以及用户需求的不断增加,微服务架构的优势越来越受到重视。随之而来的是,容器化的微服务架构也变得尤为重要,它可以更好地满足高可用性、高性能、高扩展性等方面的需求。而在这个趋势下,go-zero和Kubernetes成为了最受欢迎的容器化微服务框架。本文将介绍如何使用go-zero框架和Kubernetes容器编排工具构建高可用性、高性能
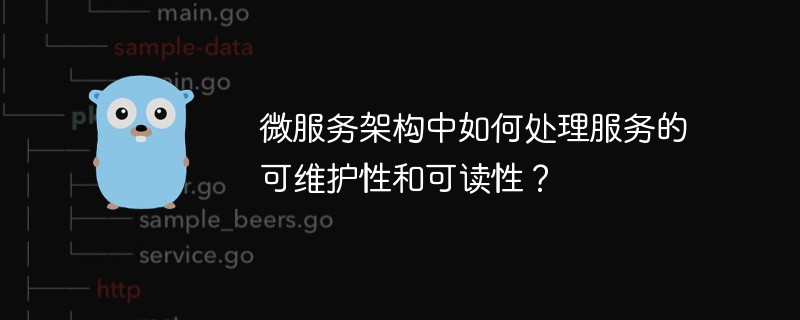
在当前的软件开发中,微服务架构已经逐渐成为了一个关注的焦点。微服务架构是指将应用程序拆分成多个小型的服务,并且每个服务都可以独立部署和运行。这种架构风格可以提高应用程序的可扩展性和可靠性,但也会带来新的挑战。其中最重要的挑战之一就是如何处理微服务的可维护性和可读性问题。微服务的可维护性在微服务架构中,每个服务都要负责单独的业务领域或模块。这样可以使得服务之间
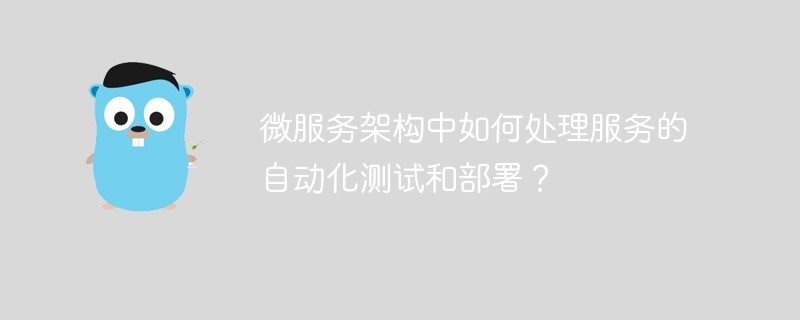
随着互联网技术的快速发展,微服务架构也越来越被广泛应用。使用微服务架构可以有效避免单体应用的复杂度和代码耦合,提高应用的可扩展性和可维护性。然而,与单体应用不同,在微服务架构中,服务数量庞大,每个服务都需要进行自动化测试和部署,以确保服务的质量和可靠性。本文将针对微服务架构中如何处理服务的自动化测试和部署进行探讨。一、微服务架构中的自动化测试自动化测试是保证
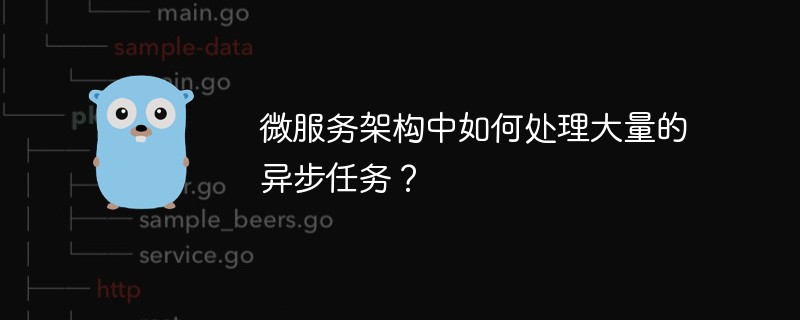
随着云计算和大数据时代的到来,解决并发问题已经成为了互联网架构设计的关键。而微服务架构作为云时代下的一种较为先进的架构方式,其本身的异步任务处理能力成为了其优势之一。但是,当异步任务数量急剧增加时,也会给微服务架构的性能和稳定性带来挑战。本文将从异步任务的定义、微服务架构的异步任务处理原理以及解决方案等方面进行探讨。一、异步任务的定义和类型异步任务,顾名思义
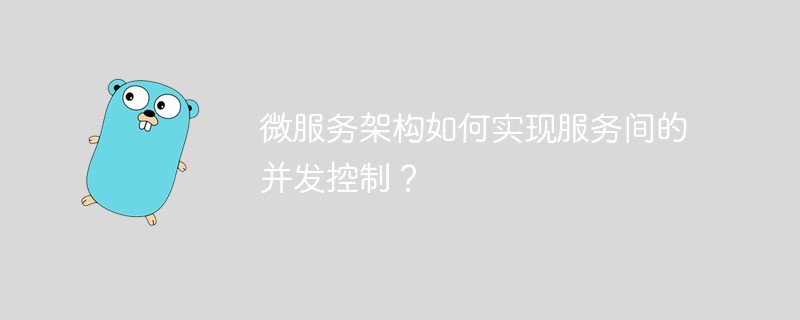
随着信息技术的不断发展,微服务架构已成为当今互联网领域中最受欢迎的架构之一。微服务架构采用小型服务组件化的方式来构建应用程序,每个服务组件都独立运行,并通过轻量级的通信机制相互协作。但是,由于微服务架构中服务之间的高度耦合和紧密联系,服务之间的并发控制问题不可避免地会出现。在微服务架构中,服务之间的并发控制问题主要存在于以下两个方面:相互之间的数据依赖:不同
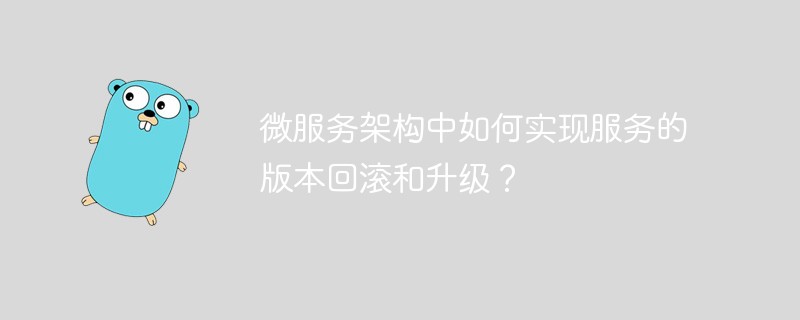
随着互联网技术的快速发展,微服务架构逐渐成为了互联网企业中技术部门的主流选择。相对于单体架构,微服务架构可以更加灵活、高效地进行系统开发和运维。在微服务架构中,每个服务都是一个相对独立的单元,可以独立部署、升级和回滚。因此,在微服务架构中,服务升级和回滚是非常常见的操作。那么,如何在微服务架构中实现服务的版本回滚和升级呢?本文将对此进行探讨和介绍。一、服务版
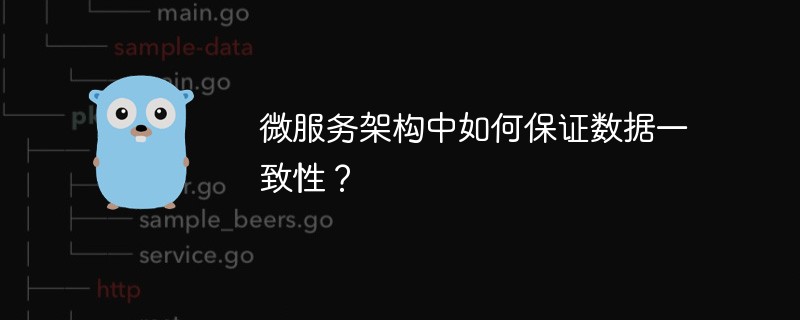
随着云计算和大数据技术的快速发展,微服务架构已经成为很多企业重要的技术选型之一,它通过将应用程序拆分成多个小型的服务来降低应用开发和维护的复杂性,同时可以支持灵活性和可伸缩性,提高应用程序的性能和可用性。然而,在微服务架构中,数据一致性是一个重要的挑战。由于微服务间的相互独立性,每个服务都拥有自己的本地数据存储,因此在多个服务之间保持数据一致性是一个非常复杂
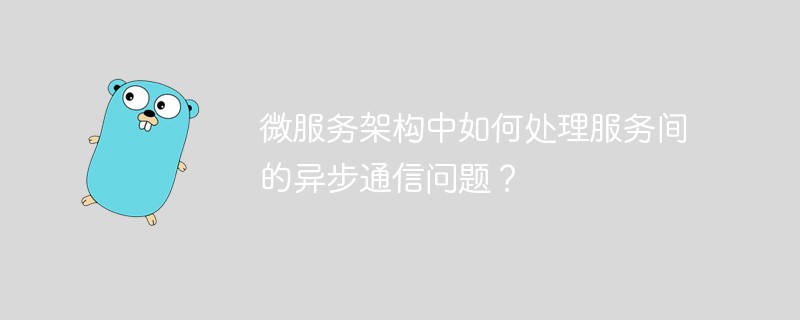
随着互联网技术的发展,各种应用系统的规模和复杂度也在不断增加。传统的单体应用架构难以应对快速增长的访问量和日益复杂的业务逻辑。因此,微服务架构成为了许多企业和开发者的选择。微服务架构将单一的应用拆分成多个独立的服务,通过各自的API接口实现服务间的交互和通信。这种将应用程序划分为小型服务的方式不仅能够方便开发和部署,而且还能够提高整体的可伸缩性和可维护性。但


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
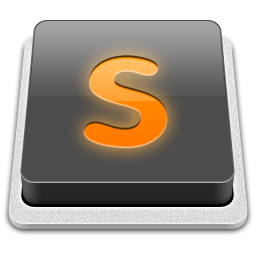
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
