Python is a widely used high-level programming language with a rich set of libraries and tools that make content extraction easier and more efficient. Among them, regular expressions are a very important tool, and Python provides the re module to use regular expressions for content extraction. This article will introduce you to the specific steps on how to use Python regular expressions for content extraction.
1. Understand the basic syntax of regular expressions
Before using Python regular expressions for content extraction, you first need to understand the basic syntax rules of regular expressions. Regular expression is a text pattern used to describe character patterns. Its basic syntax includes the following:
1. Metacharacters: characters that represent special meanings, such as: '.' means matching any character, '^' means matching the beginning of the line, '$' means matching the end of the line, etc.
2. Character set: It means that it can match one of multiple characters. For example: '[abc]' means that it matches any one of 'a', 'b', and 'c' characters.
3. Quantifier: a symbol indicating the number of matches, such as: '*' means matching zero or more times, ' ' means matching one or more times, '?' means matching zero or one time, etc.
4. Grouping: Combine multiple characters into a whole to match, for example: '(abc)' means matching the whole 'abc'.
2. Use the re module for regular expression matching
In Python, the main tool for content extraction using regular expressions is the re module. This module provides a set of functions that facilitate regular expression matching.
1.re.match() function: matches the regular expression at the beginning of the string. If the match is successful, the matching object is returned; if the match fails, None is returned.
Sample code:
import re # 匹配字符串中的数字 text = 'Hello 123456 World' matchObj = re.match(r'd+', text) if matchObj: print("matchObj.group() : ", matchObj.group()) else: print("No match!!")
Output result:
matchObj.group() : 123456
2.re.search() function: Match regular expressions in the entire string. If the match is successful, the matching object is returned; if the match fails, None is returned.
Sample code:
import re # 搜索字符串中的数字 text = 'Hello 123456 World' matchObj = re.search(r'd+', text) if matchObj: print("matchObj.group() : ", matchObj.group()) else: print("No match!!")
Output result:
matchObj.group() : 123456
3.re.findall() function: Find all substrings matching the regular expression in the string, and Return a list.
Sample code:
import re # 查找字符串中的所有数字 text = 'Hello 123456 World' matchList = re.findall(r'd+', text) print(matchList)
Output result:
['123456']
4.re.sub() function: Replace the substring matching the regular expression in the string.
Sample code:
import re # 将字符串中的数字替换为'X' text = 'Hello 123456 World' newText = re.sub(r'd+', 'X', text) print(newText)
Output result:
Hello X World
3. Example analysis
The following uses an example to further understand the use of Python regular expressions. .
On the Internet, many websites have crawler restrictions and require the use of cookies for authentication. So how do you extract cookies from HTTP response headers using Python regular expressions? Please look at the sample code below:
import re # 模拟HTTP响应头 responseHeader = ''' HTTP/1.1 200 OK Content-Type: text/html; charset=utf-8 Set-Cookie: SESSIONID=1234567890abcdef; Domain=example.com; Path=/ Set-Cookie: USERNAME=admin; Domain=example.com; Path=/ ''' # 提取cookie cookiePattern = r'Set-Cookie: (.+?);' cookieList = re.findall(cookiePattern, responseHeader) # 输出cookie print(cookieList)
Output results:
['SESSIONID=1234567890abcdef', 'USERNAME=admin']
By using the re.findall() function and the regular expression pattern 'Set-Cookie: (. ?);', you can Conveniently extract cookie information from HTTP response headers.
4. Summary
This article introduces the basic syntax rules of Python regular expressions and how to use the re module for regular expression matching. Through a specific example, it shows how to use Python regular expressions to extract cookies from HTTP response headers. Regular expressions are a very important tool in Python, which can greatly facilitate content extraction. Hopefully this article can help you get better at using Python for content extraction.
The above is the detailed content of How to use Python regular expressions for content extraction. For more information, please follow other related articles on the PHP Chinese website!
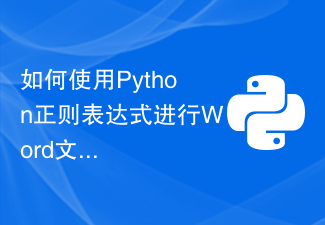
Python正则表达式是一种强大的匹配工具,它可以帮助我们在Word文件处理中快速识别并替换文字、样式和格式。本文将介绍如何使用Python正则表达式进行Word文件处理。一、安装Python-docx库Python-docx是Python中处理Word文档的功能库,使用它可以快速读取、修改、创建和保存Word文档。在使用Python-docx之前,需要保证
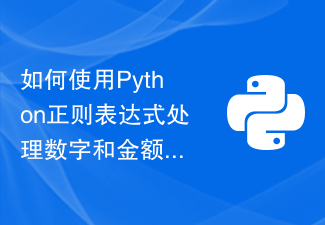
Python正则表达式是一种强大的工具,可帮助我们在文本数据中进行精细、高效的匹配和搜索。在数字和金额的处理中,正则表达式也极为有用,可以准确地找到并提取其中的数字和金额信息。本文将介绍如何使用Python正则表达式处理数字和金额,帮助读者更好地应对实际的数据处理任务。一、处理数字1.匹配整数和浮点数正则表达式中,要匹配整数和浮点数,可以使用d+进行匹配,其
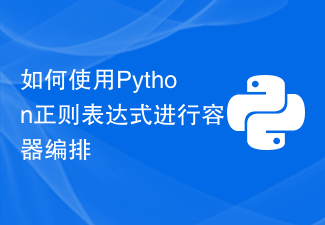
在容器编排中,我们常常需要对一些信息进行筛选、匹配和替换等操作。Python提供了正则表达式这一强大的工具,可以帮助我们完成这些操作。本文将介绍如何使用Python正则表达式进行容器编排,包括正则基础知识、Pythonre模块的使用方法以及一些常见的正则表达式应用。一、正则表达式基础知识正则表达式(RegularExpression)是指一种文本模式,用
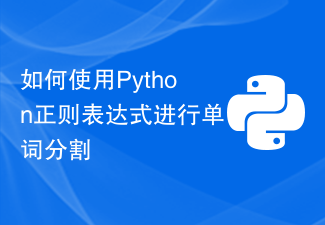
Python正则表达式是一种强大的工具,可用于处理文本数据。在自然语言处理中,单词分割是一个重要的任务,它可以将一段文本分成单个单词。在Python中,我们可以使用正则表达式来完成单词分割的任务。下面将以Python3为例,介绍如何使用正则表达式进行单词分割。导入re模块re模块是Python内置的正则表达式模块,首先需要导入该模块。importre定义文
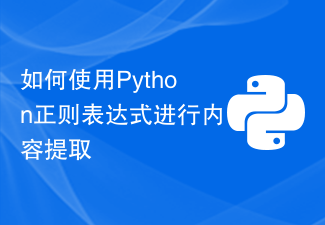
Python是一种广泛使用的高级编程语言,拥有丰富的库和工具,使得内容提取变得更加简单和高效。其中,正则表达式是一种非常重要的工具,Python提供了re模块来使用正则表达式进行内容提取。本文将为您介绍如何使用Python正则表达式进行内容提取的具体步骤。一、了解正则表达式的基本语法在使用Python正则表达式进行内容提取之前,首先需要了解正则表达式的基本语
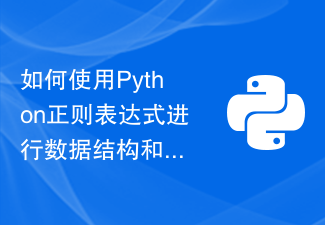
Python正则表达式是一种基于模式匹配的字符串处理工具,它可以帮助我们快速有效地从文本中提取所需信息。在数据结构和算法中,正则表达式可以用来实现文本匹配、替换、分割等功能,为我们的编程提供更加强大的支持。本文将介绍如何使用Python正则表达式进行数据结构和算法。一、正则表达式的基础知识在开始之前,先了解一下正则表达式的一些基础知识:字符集:用方括号表示,
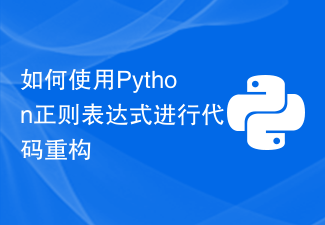
在日常编码中,我们经常需要对代码进行修改和重构,以增加代码的可读性和可维护性。其中一个重要的工具就是正则表达式。本篇文章将介绍如何使用Python正则表达式进行代码重构的一些常用技巧。一、查找和替换正则表达式最常用的功能之一是查找和替换。假设我们需要将代码中所有的print语句替换成logging语句。我们可以使用以下正则表达式进行查找:prints*((.
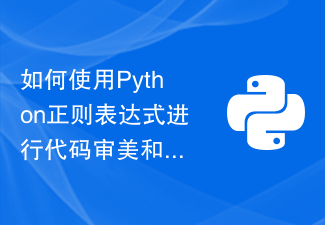
在软件开发中,代码审美和用户体验常常被忽视,这导致许多软件在实际使用中出现问题。Python作为一门强大的编程语言,提供了正则表达式这一强大的工具来帮助我们解决这些问题。本文将介绍如何使用Python正则表达式进行代码审美和用户体验。一、Python正则表达式简介正则表达式是一种描述文本模式的语言,可以用来匹配、查找、替换和拆分文本。Python的re模块提


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
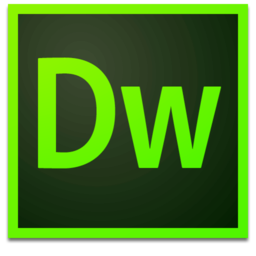
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
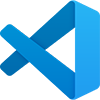
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
