With the rapid development of the Internet and mobile Internet, more and more applications require authentication and permission control, and JWT (JSON Web Token), as a lightweight authentication and authorization mechanism, is used in WEB applications is widely used in.
Beego is an MVC framework based on the Go language, which has the advantages of efficiency, simplicity, and scalability. This article will introduce how to use JWT to implement authentication in Beego.
1. Introduction to JWT
JSON Web Token (JWT) is an open standard (RFC 7519) for transmitting identity and claim information over the network. It can securely transfer information between various systems because it can encrypt and digitally sign information. A JWT consists of three parts: header, claim and signature. Where headers and claims are encoded using base64, the signature uses a key to encrypt the data.
2. Beego integrates JWT
1. Install dependencies
First we need to install two dependency packages:
go get github.com/dgrijalva/ jwt-go
go get github.com/astaxie/beego
2. Create a JWT tool class
We can create a JWT tool class by encapsulating the JWT operation for generating , verify JWT and other operations. These include methods such as issuing tokens, verifying tokens, and obtaining information stored in tokens. The code is as follows:
package utils import ( "errors" "github.com/dgrijalva/jwt-go" "time" ) // JWT构造体 type JWT struct { signingKey []byte } // 定义JWT参数 type CustomClaims struct { UserID string `json:"userId"` UserName string `json:"userName"` jwt.StandardClaims } // 构造函数 func NewJWT() *JWT { return &JWT{ []byte("jwt-secret-key"), } } // 生成token func (j *JWT) CreateToken(claims CustomClaims) (string, error) { token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims) return token.SignedString(j.signingKey) } // 解析token func (j *JWT) ParseToken(tokenString string) (*CustomClaims, error) { token, err := jwt.ParseWithClaims(tokenString, &CustomClaims{}, func(token *jwt.Token) (interface{}, error) { if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok { return nil, errors.New("签名方法不正确") } return j.signingKey, nil }) if err != nil { return nil, err } if claims, ok := token.Claims.(*CustomClaims); ok && token.Valid { return claims, nil } return nil, errors.New("无效的token") }
3. Use JWT for authentication
In Beego, we can use middleware to verify the user's identity, for example:
package controllers import ( "myProject/utils" "github.com/astaxie/beego" "github.com/dgrijalva/jwt-go" ) type BaseController struct { beego.Controller } type CustomClaims struct { UserID string `json:"userId"` UserName string `json:"userName"` jwt.StandardClaims } func (c *BaseController) Prepare() { // 获取请求头中的token tokenString := c.Ctx.Request.Header.Get("Authorization") // 创建JWT实例 jwt := utils.NewJWT() // 解析token,获取token中存储的用户信息 claims, err := jwt.ParseToken(tokenString) if err != nil { c.Data["json"] = "无效的token" c.ServeJSON() return } // 验证token中的用户信息 if claims.UserID != "123456" || claims.UserName != "test" { c.Data["json"] = "用户信息验证失败" c.ServeJSON() return } }
In In the above code, we first obtain the token in the request header, and then parse the token through JWT to obtain the user information stored in it. Finally, we verify the user information in the token with the user information stored in our database. Only after passing the verification can we access the relevant interfaces normally.
3. Summary
Through the above steps, we have successfully integrated the JWT authentication mechanism and implemented user identity verification, permission control and other operations in the Beego application. However, it should be noted that in actual applications, we need to ensure the security of the JWT key, and we also need to consider whether the information stored in the JWT is reasonable.
The above is the detailed content of Using JWT to implement authentication in Beego. For more information, please follow other related articles on the PHP Chinese website!
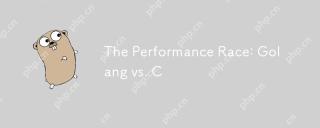
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
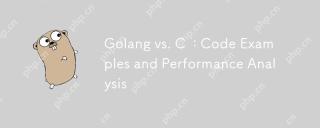
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
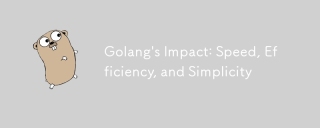
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
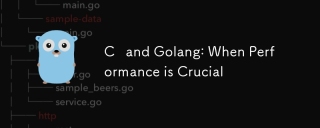
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
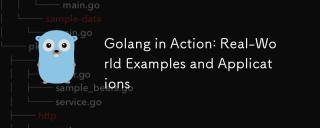
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
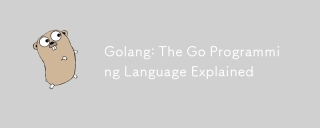
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
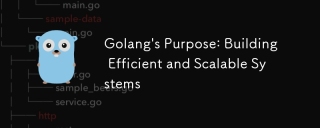
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
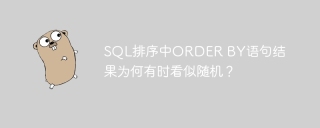
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
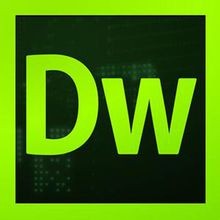
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
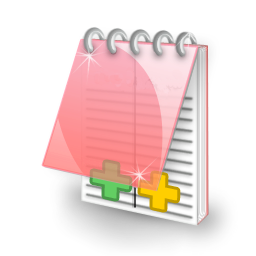
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.