Use go-zero to implement distributed cross-language RPC calls
With the growth of business scale, the existence of single applications can no longer meet the needs of the system, and distributed architecture has gradually become the mainstream. In distributed systems, RPC has become an indispensable part. It provides a convenient, efficient, and reliable way to remotely call services, enabling fast and stable data interaction and calls between various services.
For cross-language RPC calls, both the communication protocol and the serialization protocol need to be compatible with multiple programming languages, so it is relatively difficult to implement. This article will introduce how to use the go-zero framework to implement cross-language distributed RPC calls, aiming to provide readers with a practical solution.
- Introduction to go-zero framework
go-zero is a lightweight Web framework that uses the native net/http module of the go language and provides a set of A simple, easy-to-use, high-performance API development method that can easily combine HTTP services with microservices. go-zero can help us quickly build distributed, high-concurrency server applications, and the code and documentation can be obtained for free on GitHub.
- Realize cross-language RPC calls
2.1 Define services
When we define services in go-zero, we need to first write a proto file and define Communication interface between server and client. Suppose we define a service named Example, which contains two methods:
syntax = "proto3"; package rpc; service Example { rpc SayHello (Request) returns (Response); rpc GetUser (UserRequest) returns (UserResponse); } message Request { string name = 1; } message Response { string message = 1; } message UserRequest { string id = 1; } message UserResponse { string name = 1; string email = 2; }
After defining the proto file, we need to use the protobuf compiler to compile it into a go language source file and execute the following command:
protoc --go_out=. --go-grpc_out=. rpc.proto
This will generate two files, rpc.pb.go and rpc_grpc.pb.go.
2.2 Implementing the server
In the go-zero framework, we can use the go-grpc module to implement the grpc service. When implementing the server, you need to implement the interface defined in the proto file, use server.NewServer provided by go-zero and call the AddService method to add the service, and then start the grpc service in the Init method.
package server import ( "context" "rpc" "github.com/tal-tech/go-zero/core/logx" "github.com/tal-tech/go-zero/core/stores/sqlx" "github.com/tal-tech/go-zero/core/syncx" "github.com/tal-tech/go-zero/zrpc" "google.golang.org/grpc" ) type ExampleContext struct { Logx logx.Logger SqlConn sqlx.SqlConn CacheConn syncx.SharedCalls } type ExampleServer struct { Example rpc.ExampleServer } func NewExampleServer(ctx ExampleContext) *ExampleServer { return &ExampleServer{ Example: &exampleService{ ctx: ctx, }, } } func (s *ExampleServer) Init() { server := zrpc.MustNewServer(zrpc.RpcServerConf{ BindAddress: "localhost:7777", }) rpc.RegisterExampleServer(server, s.Example) server.Start() } type exampleService struct { ctx ExampleContext } func (s *exampleService) SayHello(ctx context.Context, req *rpc.Request) (*rpc.Response, error) { return &rpc.Response{ Message: "Hello, " + req.Name, }, nil } func (s *exampleService) GetUser(ctx context.Context, req *rpc.UserRequest) (*rpc.UserResponse, error) { // 查询数据库 return &rpc.UserResponse{ Name: "name", Email: "email", }, nil }
On the server, we can use the Init method to start the RPC server and use MustNewServer to create the RPC server. We must pass in an RpcServerConf structure containing the address we want to bind.
2.3 Implementing the client
In the go-zero framework, we can use the zrpc module to implement the grpc client. Use zrpc.Dial to create a connection and instantiate the rpc client.
package client import ( "context" "rpc" "google.golang.org/grpc" ) type ExampleClient struct { client rpc.ExampleClient } func NewExampleClient(conn *grpc.ClientConn) *ExampleClient { return &ExampleClient{ client: rpc.NewExampleClient(conn), } } func (c *ExampleClient) SayHello(name string) (string, error) { resp, err := c.client.SayHello(context.Background(), &rpc.Request{ Name: name, }) if err != nil { return "", err } return resp.Message, nil } func (c *ExampleClient) GetUser(id string) (*rpc.UserResponse, error) { return c.client.GetUser(context.Background(), &rpc.UserRequest{ Id: id, }) }
On the client, we only need to use the NewExampleClient function to create an RPC client. The function of the SayHello method is to obtain a response from the server and return it. The GetUser method obtains the user information response from the server and returns it in the form of UserResponse.
2.4 Test
Now that we have implemented the server and client code, we can test it through the following code:
package main import ( "fmt" "log" "rpc_example/client" "rpc_example/server" "google.golang.org/grpc" ) func main() { ctx := server.ExampleContext{} conn, err := grpc.Dial("localhost:7777", grpc.WithInsecure()) if err != nil { log.Fatalf("grpc.Dial err :%v", err) } defer conn.Close() client := client.NewExampleClient(conn) resp, err := client.SayHello("Alice") if err != nil { log.Fatalf("client.SayHello err : %v", err) } fmt.Println(resp) user, err := client.GetUser("123") if err != nil { log.Fatalf("client.GetUser err : %v", err) } fmt.Println(user) }
In the above code, we create Open a grpc connection and call the SayHello and GetUser methods to test our RPC service. We can successfully respond with the correct data and confirm that the RPC service is working normally.
- Summary
In this article, we introduced how to use the go-zero framework to implement distributed cross-language RPC calls, which involves go-zero's Def module , grpc, protobuf and zrpc and other technologies. When implementing RPC services, we first define the RPC interface, and then write server and client code based on the interface. Finally add the Init method to start the RPC service. If you are looking for a lightweight, easy-to-use distributed system framework, go-zero is definitely a good choice.
The above is the detailed content of Use go-zero to implement distributed cross-language RPC calls. For more information, please follow other related articles on the PHP Chinese website!
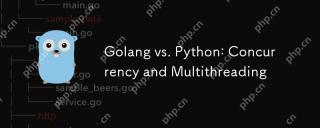
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
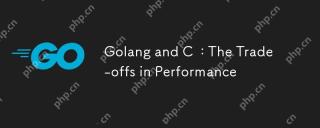
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
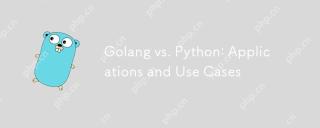
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
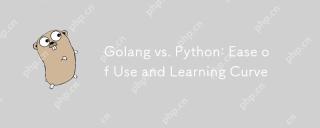
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
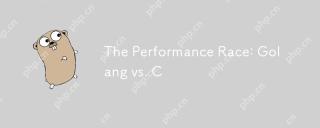
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
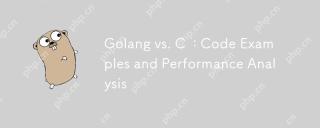
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
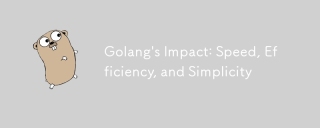
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
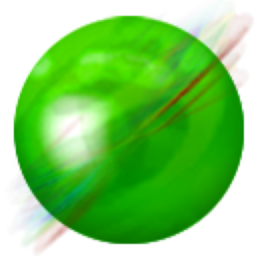
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
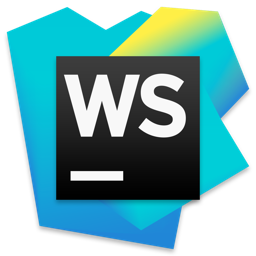
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version