


PHP Regular Expression: How to extract a substring with a specific ending from a string
In many PHP programs, we need to extract a substring with a specific ending from a string, such as extracting all file names ending with ".txt" from a set of file names. At this time, using regular expressions can help us implement this function quickly and effectively.
PHP provides many regular expression functions, the most commonly used of which are preg_match, preg_match_all and preg_replace. In this article, we will introduce how to use the preg_match and preg_match_all functions to extract substrings with specific endings from a string.
First, we need to specify the regular expression used to match a specific ending substring. In this example, we want to match all substrings ending with ".txt", so the matching regular expression should be "/.txt$/" . The regular expression consists of the following parts:
- "/": the start tag of the regular expression.
- ".": Matches the "." character. Since "." has a special meaning in regular expressions, it needs to be escaped with a backslash.
- "txt": Matches the "txt" string.
- "$": Matches the end of the string.
- "/": The end tag of the regular expression.
Next, let’s see how to use the preg_match function to match the first substring ending with ".txt" from a string:
$string = "file1.txt file2.php file3.txt file4.html"; $pattern = "/.txt$/"; if (preg_match($pattern, $string, $match)) { echo "找到匹配的子字符串:".$match[0]; } else { echo "没有找到匹配的子字符串!"; }
In this example, We first define a string $string that contains multiple file names. Then, we define the regular expression $pattern to match the substring ending in ".txt" and call the preg_match function. The first parameter of the function is the regular expression, the second parameter is the string to be matched, and the third parameter is the matched result. If no result is found, the function returns false.
Finally, we determine whether a specific ending substring is matched by determining whether there are elements in the $match array. If there is, the substring is output. Otherwise, "No matching substring found!" is output.
Next, let’s see how to use the preg_match_all function to match all substrings ending with ".txt" from a string:
$string = "file1.txt file2.php file3.txt file4.html"; $pattern = "/.txt$/"; if (preg_match_all($pattern, $string, $matches)) { echo "找到 ".count($matches[0])." 个匹配的子字符串:<br>"; foreach ($matches[0] as $match) { echo $match."<br>"; } } else { echo "没有找到匹配的子字符串!"; }
In this example, we also use $ The two variables string and $pattern define the strings and regular expressions that need to be matched. Then, we called the preg_match_all function. Unlike the preg_match function, this function can match all qualified substrings in a string, and the results are returned in an array.
When looping to output the matching results, we used the foreach loop to traverse the first element in the $matches array. Since this element is also an array, we use the $match variable to save the currently traversed substring and output it.
If no matching result is found, "No matching substring found!" will also be output.
To summarize, using regular expressions can help us extract specific ending substrings in strings quickly and efficiently. In practical applications, we can define different regular expressions according to actual needs and flexibly apply them in combination with functions such as preg_match, preg_match_all and preg_replace.
The above is the detailed content of PHP Regular Expression: How to extract a substring with a specific ending from a string. For more information, please follow other related articles on the PHP Chinese website!
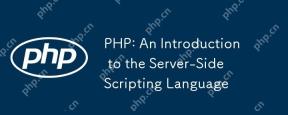
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
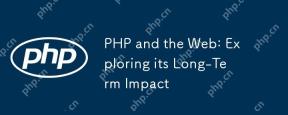
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
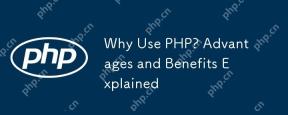
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
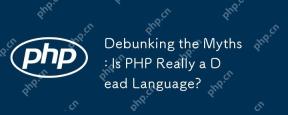
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
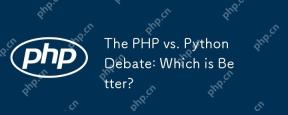
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
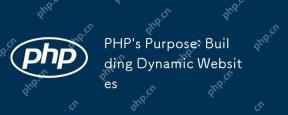
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
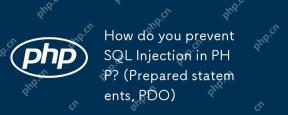
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
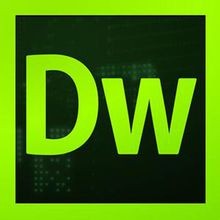
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
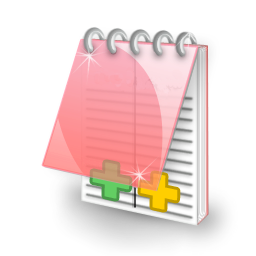
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.