


Practical tips for using caching in Golang to improve web application calls.
Practical tips for using caching to improve web application calls in Golang
Golang is an efficient, concise, and concurrent programming language that is increasingly favored by developers. As web applications become more complex and data volumes increase, program performance may suffer. To solve these problems, using caching techniques can significantly improve the efficiency of web application calls. This article will introduce how to use caching techniques in Golang to improve the performance of web applications.
1. Why use cache?
In web applications, in order to meet user needs, data needs to be read from a database or other data sources, and then processed and calculated. Typically, this process is time-consuming and may consume a large amount of system resources, affecting the response time and performance of the web application. Using caching techniques can avoid this situation, store data in the cache, and increase the speed of data reading and processing, thereby speeding up the response time and performance of web applications. The advantages of caching techniques include:
1. Improve the response speed of web applications
Using caching techniques can reduce the time of reading data from the data source and increase the speed of data reading and processing. Speed up the response time of web applications.
2. Reduce the usage of system resources
Using caching techniques can reduce the number of times data is read from the data source, thereby reducing the usage of system resources and improving system performance.
3. Improve user experience
Improvements in web application response speed will bring a better user experience and make users more satisfied, thereby increasing user retention and conversion rates.
2. Cache implementation
There are many ways to implement caching in Golang. Two commonly used methods are introduced below:
1. Memory-based caching
Memory-based caching is a caching method that stores data in memory. Its advantage lies in reading data. Very fast and suitable for scenarios that require high real-time data. However, memory is limited, and for applications with large amounts of data, memory overflow may occur, causing the program to crash. In this case, you can avoid memory overflow by setting the cache expiration time.
There are many ways to implement memory-based cache, including using sync.Map, map, struct, etc. Take using map as a cache implementation as an example:
// 定义缓存结构体 type Cache struct { data map[string]interface{} lock sync.RWMutex // 读写锁,保证并发安全 } // 获取缓存 func (c *Cache) Get(key string) interface{} { c.lock.RLock() defer c.lock.RUnlock() return c.data[key] } // 设置缓存 func (c *Cache) Set(key string, value interface{}) { c.lock.Lock() defer c.lock.Unlock() c.data[key] = value } // 删除缓存 func (c *Cache) Delete(key string) { c.lock.Lock() defer c.lock.Unlock() delete(c.data, key) }
In the above code, the Cache structure implements methods such as Get, Set, and Delete, and achieves concurrency security through read-write locks. In the code, map is used as the cache implementation.
2. Disk-based caching
Disk-based caching is a caching method that stores data on disk. Its advantage is that it can store a large amount of data and is suitable for real-time processing of data. Scenes where sex is not required. However, it is slower to read than the in-memory cache, which may have an impact on the performance of your web application.
Golang can use GCache to implement disk-based caching. It is a high-performance, disk-based caching library that can cache any object that can be encoded by Gob. The usage method is as follows:
// 创建缓存 fileCache := gcache.NewFileCache("/tmp/cache") // 设置缓存 err := fileCache.Set("key", "value", time.Hour) if err != nil { // 处理错误 } // 获取缓存 value, err := fileCache.Get("key") if err != nil { // 处理错误 } // 删除缓存 err := fileCache.Delete("key") if err != nil { // 处理错误 }
In the above code, a disk-based cache is created, the Set method is used to set the cache value, the Get method is used to obtain the cache value, and the Delete method is used to delete the cache value. The cache expiration time is set to one hour. If you need to store large amounts of data, consider using disk-based caching.
3. Practical skills of caching
1. Choose the appropriate cache type
When using cache, you need to choose the appropriate cache based on the characteristics of the data and the characteristics of the application. type. For applications with a relatively small amount of data, you can use memory-based caching. For applications with a large amount of data, you can consider using a disk-based cache. When choosing a cache type, you also need to consider factors such as the cache's read speed and the real-time nature of the data.
2. Set the appropriate cache expiration time
The cache expiration time is an important parameter. If the time set is too long, the cache data may not be invalidated in time; if the time set is too short, the cache may be updated frequently and the system load may be increased. Therefore, it is necessary to set an appropriate cache expiration time based on the frequency of use and real-time nature of the data.
3. Use consistent hashing algorithm
The consistent hashing algorithm is an algorithm that solves the problem of distributed cache data consistency. When using distributed cache, cached data may be inconsistent. Using consistent hashing algorithms can effectively solve this problem. The basic idea of the consistent hashing algorithm is to map data to a ring, calculate the position of each node based on parameters such as the number of nodes and the number of virtual nodes, and correspond to the position of the node based on the key value of the data. In this way, when the node changes, only part of the data will be affected, but not all data, thereby solving the problem of distributed cache data consistency.
4. Use cache penetration technology
Cache penetration refers to data that does not exist in the cache and is often requested maliciously, causing application performance degradation. In order to solve this problem, cache penetration technology can be used to cache non-existent data. In this way, when the next request comes, the data can be obtained directly from the cache, avoiding frequent database requests. When using cache penetration technology, it is necessary to use algorithms such as Bloom filters to filter to avoid unnecessary data caching.
5. Periodically clear the cache
There may be some problems with the cache expiration time. Expired cache data may remain in the cache and occupy system resources. Therefore, expired cached data needs to be cleaned periodically to release system resources. When clearing cached data, you need to pay attention to concurrency safety to avoid problems such as data race conditions.
In short, using caching techniques can improve the performance and response speed of web applications, giving users a better experience. In practice, it is necessary to choose the appropriate cache type, set appropriate cache expiration time, use consistent hashing algorithm, use cache penetration technology and periodically clean cache and other techniques. I hope this article can help readers better use caching techniques and optimize the performance of web applications.
The above is the detailed content of Practical tips for using caching in Golang to improve web application calls.. For more information, please follow other related articles on the PHP Chinese website!
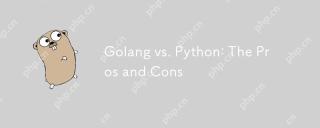
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
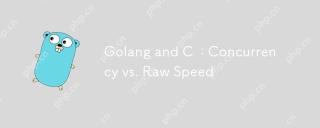
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
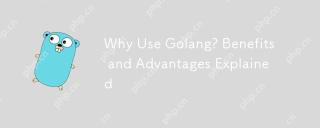
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
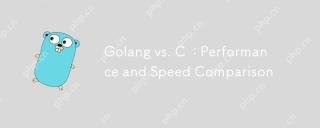
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
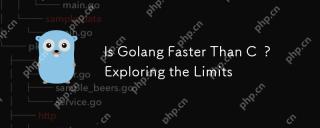
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
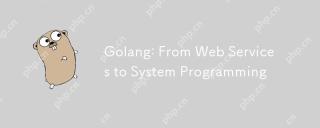
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
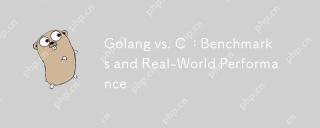
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
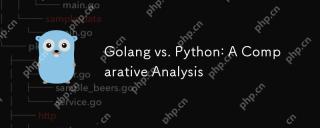
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
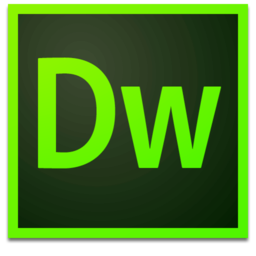
Dreamweaver Mac version
Visual web development tools
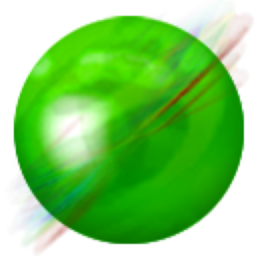
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software