


In recent years, with the rapid development of the Internet and mobile Internet and the popularization of 5G networks, the demand for audio and video processing has become higher and higher, and Golang, as an efficient programming language, is adopted by more and more developers. Using cache to improve audio and video processing performance has become a classic problem in Golang development. This article will introduce how to use caching technology in Golang to improve audio and video processing performance.
1. What is caching technology?
Caching technology is a way to optimize data access, with the goal of speeding up data reading and writing. In programming, caches are commonly used to store frequently used data for quick access. The cache can be regarded as an intermediary storage layer for data. When data needs to be obtained, the cache is accessed first. If the required data is in the cache, it is returned directly. Otherwise, the data is obtained from the data source and stored in the cache for next time. Quick access.
2. Bottlenecks in audio and video processing
One of the most common bottlenecks in audio and video processing is the IO bottleneck. Due to the large amount of audio and video data, processing is very time-consuming. Data often needs to be read from disk or network, and these IO operations are very time-consuming. Therefore, the performance of IO operations is usually the main factor affecting audio and video processing efficiency.
3. How to use caching to improve audio and video processing performance?
In order to optimize audio and video processing performance, we can use caching technology to reduce IO operations to improve audio and video processing efficiency. Specifically, memory cache or disk cache can be used to accelerate audio and video processing.
3.1. Memory cache
Memory cache can greatly reduce the number of IO operations, thereby improving audio and video processing efficiency. In Golang, we can use sync.Map or LRU cache to implement memory caching. Among them, sync.Map is a thread-safe hash table that supports concurrent reading and writing, while LRU cache is a caching method based on the least recently used principle (Least Recently Used), which is suitable for large amounts of data but difficult access. Relatively low frequency application scenarios.
Below, we take video screenshots as an example to illustrate how to use sync.Map to implement memory caching:
import "sync" var cache sync.Map func GetThumbnailFromCache(videoID string) ([]byte, error) { if v, ok := cache.Load(videoID); ok { return v.([]byte), nil } else { thumbnail, err := GetThumbnailFromVideo(videoID) if err != nil { return nil, err } cache.Store(videoID, thumbnail) return thumbnail, nil } }
In the above code, we first try to get the video screenshots in the cache. If Once obtained, return directly, otherwise, obtain the video screenshot from the video file and store it in the cache for subsequent quick access.
3.2. Disk cache
When the memory capacity is insufficient, we can use disk cache to expand the cache. Disk cache stores data on the hard disk, which can effectively avoid data loss and memory leak problems, but compared to memory cache, disk cache has slower access speed. If the access frequency is high, it is recommended to use memory cache. If the access frequency is low, disk cache can be used.
In Golang, we can use go-cache or bigcache to implement disk caching. Among them, go-cache is a general memory and disk caching library, and most data types can be cached. Bigcache is specially used to cache structures and other complex types, and has higher performance.
Below, we take video transcoding as an example to illustrate how to use go-cache to implement disk caching:
import ( "github.com/patrickmn/go-cache" "os" ) var c = cache.New(24*time.Hour, 24*time.Hour) func Transcode(videoID string) error { var result error if v, ok := c.Get(videoID); ok { result = DoTranscode(v.([]byte)) } else { videoFile, err := os.Open("path/to/video") if err != nil { return err } defer videoFile.Close() videoData, err := ioutil.ReadAll(videoFile) if err != nil { return err } result = DoTranscode(videoData) c.Set(videoID, videoData, cache.DefaultExpiration) } return result }
In the above code, we first try to obtain the video data in the cache, If it is obtained, it will be transcoded directly. Otherwise, the data will be read from the video file and transcoded. Finally, the transcoded result will be stored in the cache for subsequent quick access.
4. Summary
Caching technology is a way to optimize data access, which can effectively improve audio and video processing efficiency. In Golang, we can use sync.Map, LRU cache, go-cache, bigcache and other tools to implement memory or disk caching. Which method to choose needs to be evaluated based on the actual situation. Finally, I hope this article can help Golang developers improve audio and video processing performance.
The above is the detailed content of How to use caching to improve audio and video processing performance in Golang?. For more information, please follow other related articles on the PHP Chinese website!
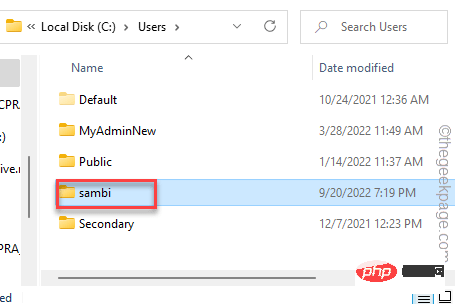
团队在Outlook中有一个非常有用的加载项,当您在使用Outlook2013或更高版本的应用程序时安装以前的应用程序时,它会自动安装。安装这两个应用程序后,只需打开Outlook,您就可以找到预装的加载项。但是,一些用户报告了在Outlook中找不到Team插件的异常情况。修复1–重新注册DLL文件有时需要重新注册特定的Teams加载项dll文件。第1步-找到MICROSOFT.TEAMS.ADDINLOADER.DLL文件1.首先,您必须确保
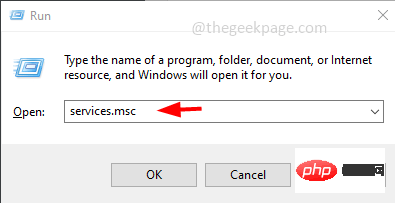
地址解析协议 (ARP) 用于将 MAC 地址映射到 IP 地址。网络上的所有主机都有自己的 IP 地址,但网络接口卡 (NIC) 将有 MAC 地址而不是 IP 地址。ARP 是用于将 IP 地址与 MAC 地址相关联的协议。所有这些条目都被收集并放置在 ARP 缓存中。映射的地址存储在缓存中,它们通常不会造成任何损害。但是,如果条目不正确或 ARP 缓存损坏,则会出现连接问题、加载问题或错误。因此,您需要清除 ARP 缓存并修复错误。在本文中,我们将研究如何清除 ARP 缓存的不同方法。方法

根据几位Windows10和Windows11用户的说法,他们在尝试安装Windows更新时遇到了错误0x80070246。此错误阻止他们升级PC并享受最新功能。值得庆幸的是,在本指南中,我们列出了一些最佳解决方案,可帮助您解决Windows0PC上80070246x11的Windows更新安装错误。我们还将首先讨论可能引发问题的原因。让我们直接进入它。为什么我会收到Windows更新安装错误0x80070246?您可能有多种原因导致您在PC上收到Windows11安装错误0x80070246。
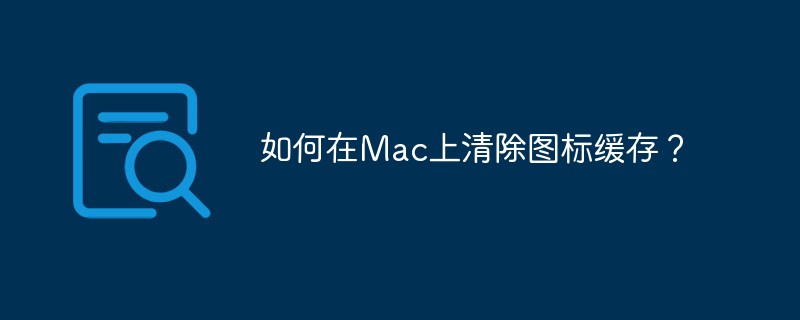
如何在Mac上清除和重置图标缓存警告:因为您将使用终端和rm命令,所以在继续执行任何操作之前,最好使用TimeMachine或您选择的备份方法备份您的Mac。输入错误的命令可能会导致永久性数据丢失,因此请务必使用准确的语法。如果您对命令行不满意,最好完全避免这种情况。启动终端并输入以下命令并按回车键:sudorm-rfv/Library/Caches/com.apple.iconservices.store接下来,输入以下命令并按回车键:sudofind/private/var
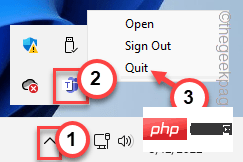
尝试在其设备上启动 Microsoft Teams 桌面客户端的用户在空白应用页面中报告了错误代码 caa70004。错误代码说:“我们很抱歉——我们遇到了问题。”以及重新启动 Microsoft Teams 以解决问题的选项。您可以尝试实施许多解决方案并再次加入会议。解决方法——1. 您应该尝试的第一件事是重新启动 Teams 应用程序。只需在错误页面上点击“重新启动”即可。
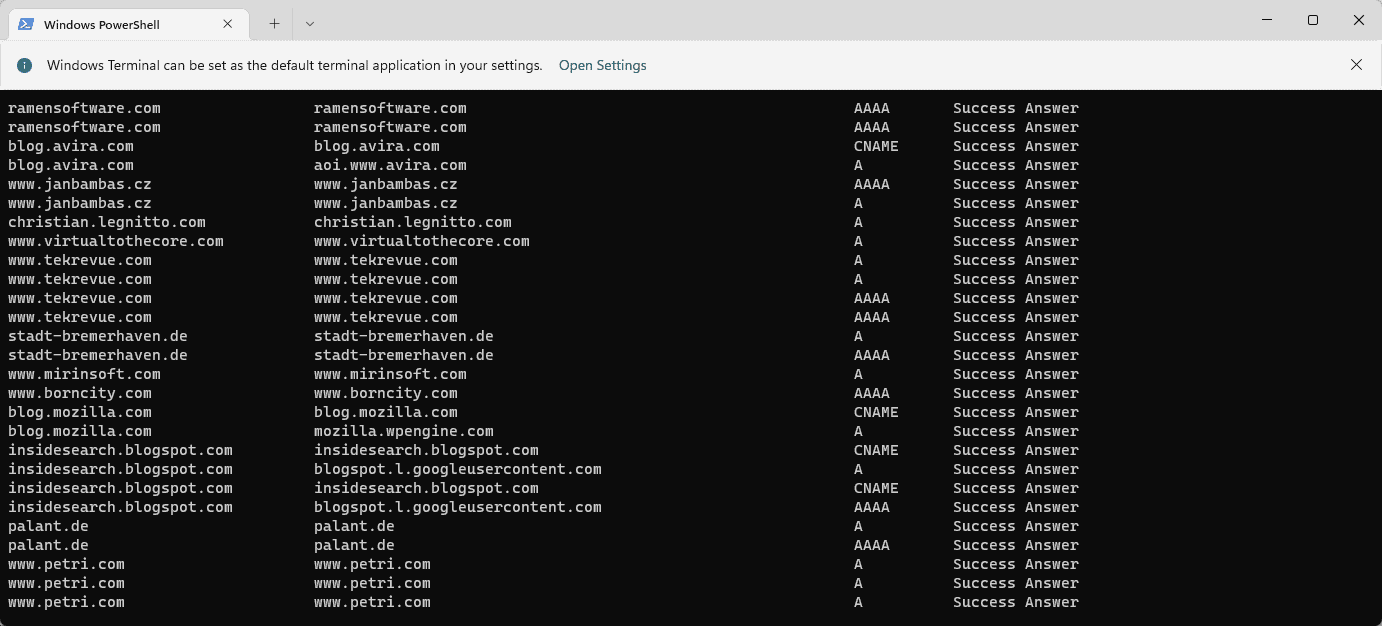
Windows操作系统使用缓存来存储DNS条目。DNS(域名系统)是用于通信的互联网核心技术。特别是用于查找域名的IP地址。当用户在浏览器中键入域名时,加载站点时执行的首要任务之一是查找其IP地址。该过程需要访问DNS服务器。通常,互联网服务提供商的DNS服务器会自动使用,但管理员可能会切换到其他DNS服务器,因为这些服务器可能更快或提供更好的隐私。如果DNS用于阻止对某些站点的访问,则切换DNS提供商也可能有助于绕过Internet审查。Windows使用DNS解
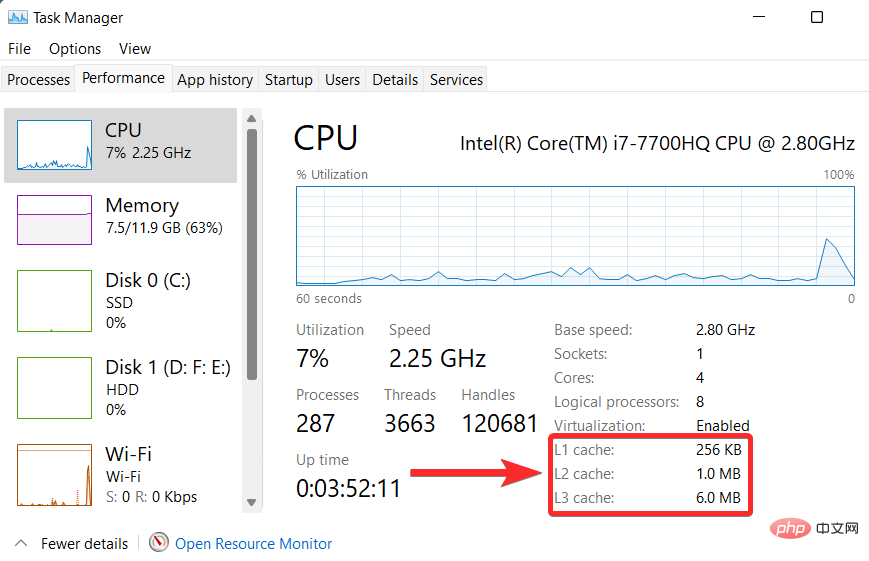
什么是缓存?缓存(发音为ka·shay)是一种专门的高速硬件或软件组件,用于存储经常请求的数据和指令,这些数据和指令又可用于更快地加载网站、应用程序、服务和系统的其他部分。缓存使最常访问的数据随时可用。缓存文件与缓存内存不同。缓存文件是指经常需要的文件,如PNG、图标、徽标、着色器等,多个程序可能需要这些文件。这些文件存储在您的物理驱动器空间中,通常是隐藏的。另一方面,高速缓存内存是一种比主内存和/或RAM更快的内存类型。它极大地减少了数据访问时间,因为与RAM相比,它更靠近CPU并且速度
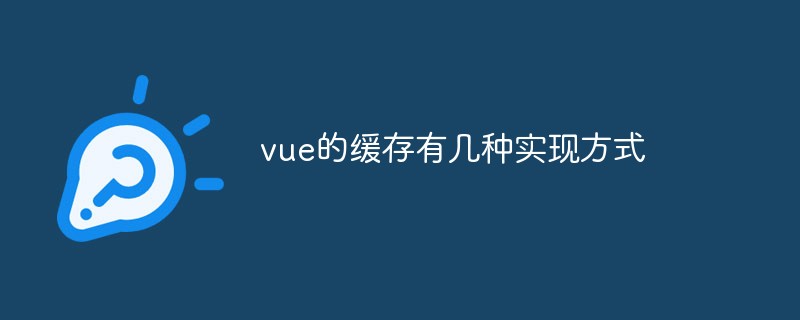
vue缓存数据有4种方式:1、利用localStorage,语法“localStorage.setItem(key,value)”;2、利用sessionStorage,语法“sessionStorage.setItem(key,value)”;3、安装并引用storage.js插件,利用该插件进行缓存;4、利用vuex,它是一个专为Vue.js应用程序开发的状态管理模式。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
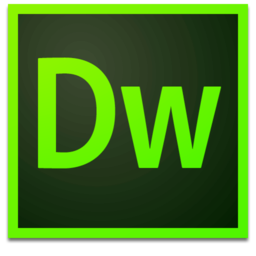
Dreamweaver Mac version
Visual web development tools
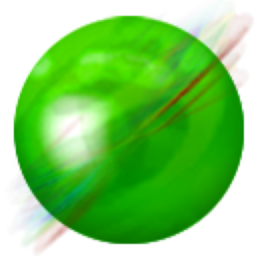
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
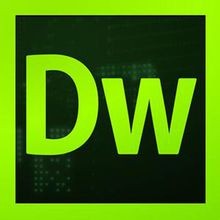
Dreamweaver CS6
Visual web development tools