PHP is a widely used open source server-side programming language that is ideal for file operations. In PHP, file copying is a relatively common operation. It can not only back up files, but also perform operations such as data transfer and backup.
This article will introduce you to various copy methods in PHP, I hope it will be helpful to you.
1. Copy the entire folder
If you need to copy the entire folder, you can use the following method:
function copyDir($dirFrom, $dirTo) { // 检查是否存在目录。 if(!is_dir($dirTo)) mkdir($dirTo); // 打开目录。 $handle = opendir($dirFrom); // 读取目录中的文件。 while(false !== ($file = readdir($handle))) { // 忽略 . 和 .. 文件夹。 if($file != "." && $file != "..") { // 复制文件。 if(is_file($dirFrom."/".$file)) { copy($dirFrom."/".$file, $dirTo."/".$file); } // 递归复制子目录。 if(is_dir($dirFrom."/".$file)) { copyDir($dirFrom."/".$file, $dirTo."/".$file); } } } // 关闭目录。 closedir($handle); }
This method will copy the entire folder, including all subdirectories and documents.
2. Copy a single file
If you only need to copy a single file, you can use the following code:
// 复制文件。 $srcFile = "file1.txt"; $destFile = "file2.txt"; if(!copy($srcFile, $destFile)) { echo "无法复制 $srcFile... "; }
3. Copy the relative path
below The code will copy the file using a relative path (for example, if both files are in the same folder):
// 复制文件。 $srcFile = "file1.txt"; $destFile = "file2.txt"; if(!copy("../".$srcFile, "../".$destFile)) { echo "无法复制 $srcFile... "; }
4. Use file stream copy
If you wish to copy the file contents to another In a file, you can use the following code:
// 打开源文件。 $srcFile = fopen("file1.txt", "r"); // 打开目标文件。 $destFile = fopen("file2.txt", "w"); // 从源文件读取,并写入目标文件。 while (($buffer = fgets($srcFile, 4096)) !== false) { fwrite($destFile, $buffer); } // 关闭文件。 fclose($srcFile); fclose($destFile);
5. Copy to remote server
The following code will copy the file to the remote server:
// 远程服务器地址。 $remoteServer = "example.com"; // 远程服务器用户名。 $remoteUser = "user"; // 远程服务器密码。 $remotePass = "password"; // 远程服务器文件夹。 $remotePath = "/path/to/remote/folder/"; // 本地文件。 $localFile = "file1.txt"; // 连接到远程服务器。 $conn = ftp_connect($remoteServer); // 登录到远程服务器。 $login = ftp_login($conn, $remoteUser, $remotePass); // 上传文件。 if (ftp_put($conn, $remotePath."/".basename($localFile), $localFile, FTP_BINARY)) { echo "文件上传成功"; } else { echo "文件上传失败"; } // 关闭FTP连接。 ftp_close($conn);
6. Copy multiple files
If you wish to copy multiple files, you can use the following code:
// 复制多个文件。 $srcFiles = array("file1.txt", "file2.txt", "file3.txt"); $destFolder = "backup/"; // 循环复制文件。 foreach($srcFiles as $file) { $destFile = $destFolder.basename($file); if(!copy($file, $destFile)) { echo "无法复制 $file... "; } }
As mentioned above, here are some common PHP file copy methods. Each method is specific to different situations and I hope this article can help you.
The above is the detailed content of PHP file operation tips: various copy methods. For more information, please follow other related articles on the PHP Chinese website!
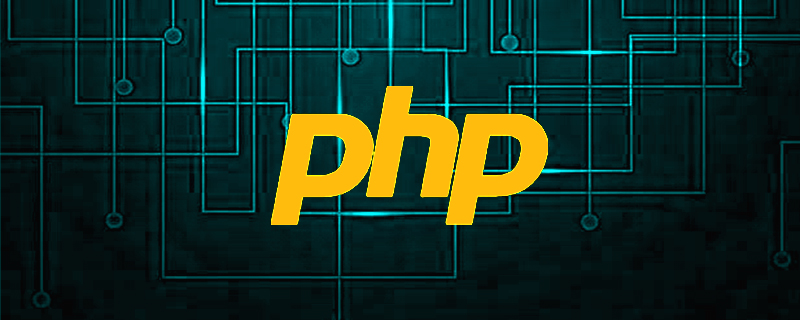
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
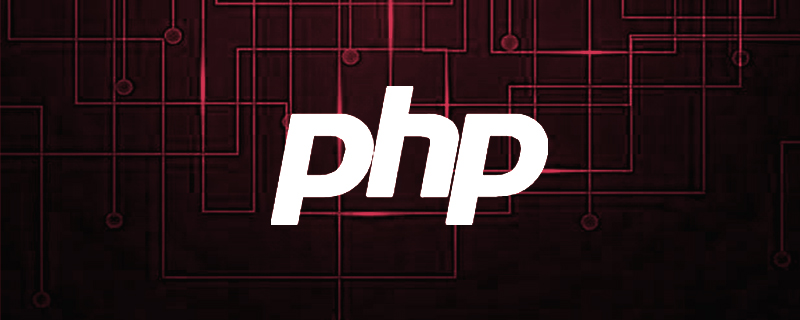
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
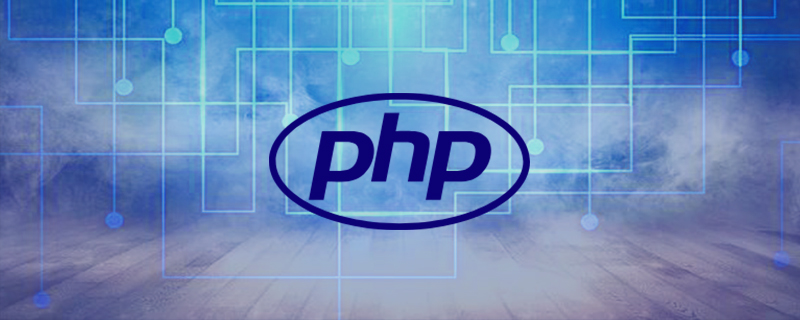
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
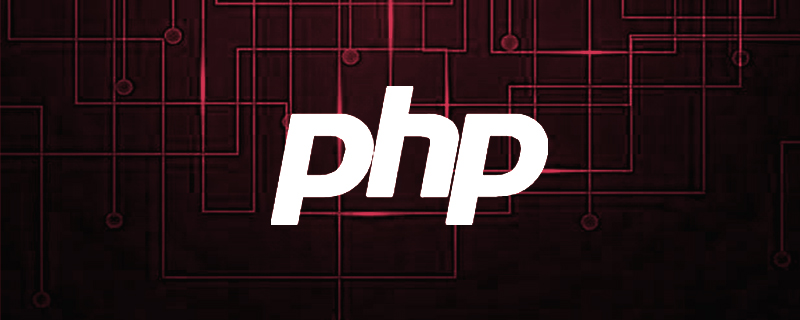
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
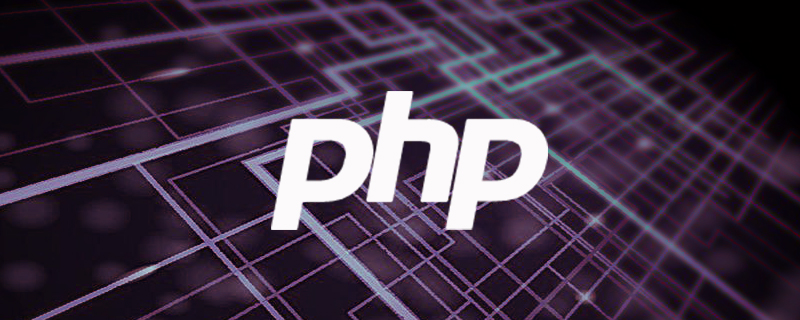
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
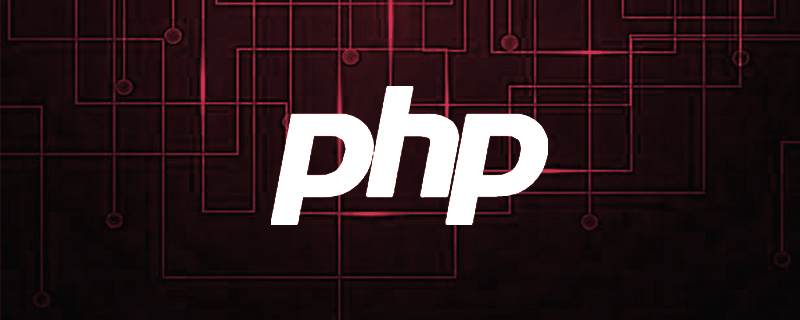
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
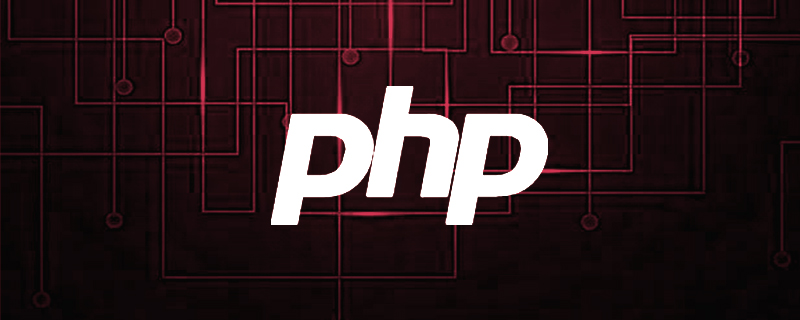
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
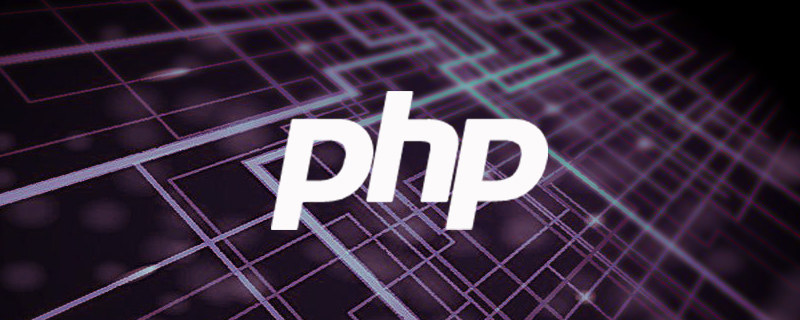
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment
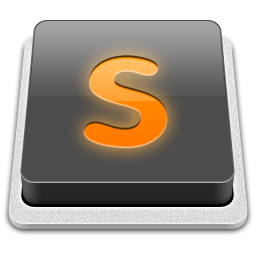
SublimeText3 Mac version
God-level code editing software (SublimeText3)
