In Java development, caching technology is very common and can help improve application performance. Caching technology reduces access to external storage devices such as disks by storing commonly used data in memory. However, in a multi-threaded scenario, how to maintain cache consistency has become one of the problems that developers need to solve. At this time, Cache Read-Write Lock becomes a good solution.
1. Thread safety issues of cache
When multiple threads access a cache at the same time, since the cached data is stored in memory, not on disk like a database, therefore For the same data, multiple threads may be reading and writing at the same time. If thread safety is not taken into consideration, the following problems may occur:
1. Data inconsistency: When multiple threads write data in the cache at the same time, data inconsistency may occur. For example, thread A writes data to the cache, but before the writing is completed, thread B also writes the same data to the cache. At this time, the data written by thread A is overwritten by B.
2. Performance issues: When multiple threads perform read and write operations at the same time, performance issues may occur. For example, thread A is reading data in the cache. If thread B wants to write the same data at this time, it needs to wait for thread A to complete the reading operation before performing the writing operation. If this happens frequently, it can affect application performance.
2. Solution to cache read-write lock
In order to solve the thread safety problem when multi-threads access the cache, Java provides a solution to cache read-write lock. Cache read-write locks are divided into read locks and write locks. Multiple threads can hold read locks at the same time for read operations, but only one thread can hold a write lock for write operations. In this way, data consistency and performance can be guaranteed when multiple threads access the cache.
The specific implementation is as follows:
1. Read operation
When reading, you need to acquire the read lock first. If there is no write lock currently, you can directly acquire the read lock. lock; if there is already a write lock, you need to wait for the write lock to be released before acquiring the read lock. The operation of obtaining a read lock is as follows:
readLock.lock(); try { //读取缓存中的数据 //... } finally { readLock.unlock(); }
2. Write operation
During the write operation, you need to obtain the write lock first. If there is currently no read lock or write lock, you can obtain it directly. Write lock; if there is already a read lock or write lock, you need to wait for all read locks and write locks to be released before acquiring the write lock. The operation of obtaining the write lock is as follows:
writeLock.lock(); try { //写入缓存中的数据 //... } finally { writeLock.unlock(); }
By using the cache read-write lock, thread safety issues when multi-threads access the cache can be guaranteed, and the performance of the application will not be affected. However, it should be noted that cached read-write locks cannot solve all thread safety issues. For example, when multiple threads write different data at the same time, race conditions and other problems may occur.
3. Summary
Cache read-write lock is a solution to ensure thread safety in Java cache technology. It ensures the consistency and performance of data when multi-threads access the cache through the control of read locks and write locks. However, it should be noted that cache read-write locks cannot solve all thread safety issues. It is necessary to comprehensively consider the use of cache read-write locks and other thread safety measures according to specific scenarios during the development process.
The above is the detailed content of Cache read-write lock in Java caching technology. For more information, please follow other related articles on the PHP Chinese website!
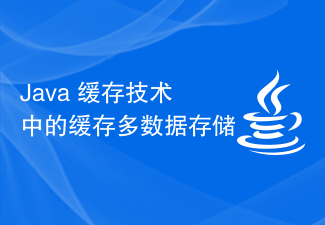
随着互联网应用的不断发展,数据量急剧增加,如何高效地读写数据成为了每个开发人员都需要面对的问题。而缓存技术正是解决这个问题的重要方法之一。而在Java缓存技术中,缓存多数据存储是一种常见的技术手段。一、什么是缓存多数据存储?缓存多数据存储是一种多级缓存机制,将缓存按照使用频率、数据大小、数据类型等因素进行分层存储,以提高缓存的存取效率。一般情况下,缓存数据分
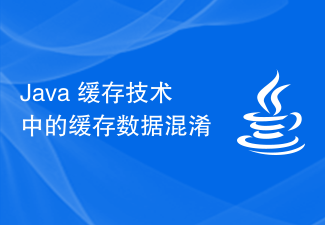
随着互联网技术的不断发展,越来越多的应用程序采用了缓存技术来提高数据的访问速度和减少数据库压力。Java作为一种流行的编程语言,也提供了多种缓存框架,如Ehcache、GuavaCache、Redis等。然而,在使用缓存技术的过程中,我们常常会遇到一个问题:缓存数据混淆。这篇文章将介绍缓存数据混淆的原因、影响以及如何解决。一、缓存数据混淆的原因缓存数据混淆
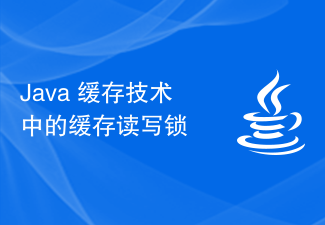
在Java开发中,缓存技术是非常常见的,可以帮助提高应用程序的性能。缓存技术通过在内存中存储常用的数据,来减少对磁盘等外部存储设备的访问。但是,在多线程的场景下,如何维护缓存的一致性,成为了开发人员需要解决的问题之一。这时候,缓存读写锁(CacheRead-WriteLock)就成为了一种很好的解决方案。一、缓存的线程安全性问题当多个线程同时访问一个
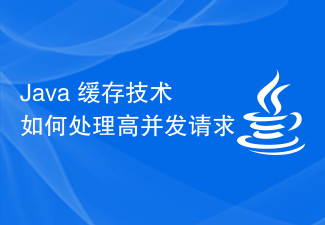
随着互联网的高速发展,越来越多的网站和应用程序开始面临高并发请求的挑战。对于Web应用程序来说,许多的请求会涉及到从数据库中读取数据,这将导致数据库变得极为繁忙,降低整个应用程序的性能。这时,为了优化应用程序的性能和响应时间,采用Java缓存技术已成为一种非常流行的解决方案。Java缓存技术能够极大地提升系统性能和响应速度,尤其当系统面临高并发请求的时候,更
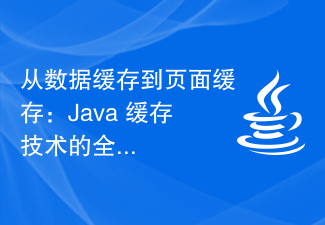
在现代软件开发中,缓存技术已经成为了关键的技术之一。Java作为目前最流行的编程语言之一,也有着非常丰富的缓存技术库。本文将从数据缓存到页面缓存,介绍Java缓存技术的全面攻略。一、数据缓存技术数据缓存技术是应用最广泛的一种缓存技术。其原理是将经常使用的数据存储在内存中,程序在访问该数据时,首先在内存中查找数据,如果找到则直接返回,否则从磁盘或者网络中
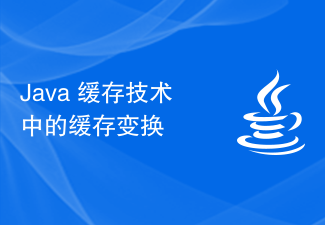
随着互联网应用的不断发展和用户量的增加,数据访问的性能一直是一个热门话题。为了提高数据的访问速度,各种缓存技术应运而生。Java作为一种广泛使用的编程语言,有着丰富的缓存机制可以用于优化应用程序的性能。其中,缓存变换作为重要的缓存技术之一,在实际应用中也具有重要的意义。一、缓存变换是什么在介绍缓存变换之前,先要了解一下缓存的基本概念。简单来说,缓存是一种用来
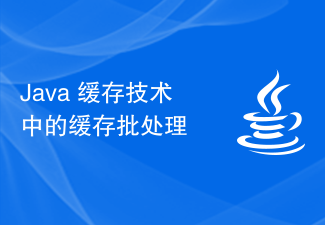
随着互联网应用的不断发展,Java缓存技术也成为了很多应用中不可或缺的一部分,可以提高程序的执行效率并减轻服务器的负担。而在Java缓存技术中,缓存批处理是一个很重要的概念,本文将重点介绍缓存批处理的相关知识。一、缓存批处理概述缓存批处理是指将多个缓存操作组合在一起执行,而不是单独执行每一个缓存操作。这样做可以提升程序执行效率,减少服务器的压力。在实际
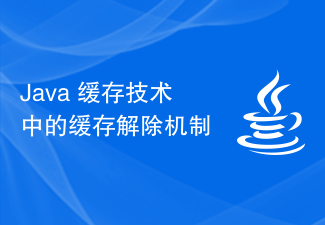
随着互联网技术的不断发展,Java缓存技术在web应用程序中得到了广泛应用。缓存技术能够大幅度提升web应用程序的运行速度,降低网络延迟,缓解服务器的压力。然而,在开发中,也经常会遇到缓存不更新或缓存数据过期的问题,其中缓存解除机制就是缓存技术中一个非常重要的环节。本文将介绍Java缓存技术中的缓存解除机制以及如何保证缓存的准确性。一、缓存解除


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
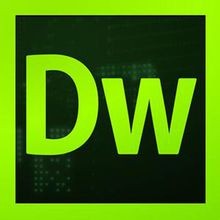
Dreamweaver CS6
Visual web development tools
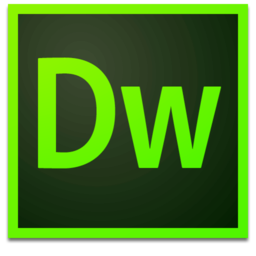
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.