How to get weather data using PHP and OpenWeatherMap API
As climate change intensifies, weather forecasts have become increasingly important in our daily lives. And how to get accurate weather forecast data on your own website? PHP and OpenWeatherMap API are two popular choices. The following will describe how to use PHP and the OpenWeatherMap API to obtain weather data.
1. Obtain API key
Using the OpenWeatherMap API requires obtaining an API key. It's completely free to register an account and get an API key. Log in to the OpenWeatherMap website (https://openweathermap.org/) and register. On the profile page, go to the API Keys tab and click the "Generate Key" button. Then you can get the API key.
2. Create PHP file
The next step is to write the code. Open your Code editor and create a PHP file, for example weather.php. First, you need to introduce the OpenWeatherMap API library. This can be achieved by adding the following code in the header:
$httpRequestURI = 'https://api.openweathermap.org/data/2.5/weather?q=London&appid={YOUR_API_KEY}'; $response = file_get_contents($httpRequestURI); $data = json_decode($response); echo $data->weather[0]->description;
Note that in the request URL, you need to replace {YOUR_API_KEY} with your API key.
Explanation code:
The first line defines the URL address to be requested, where q=London is the city you want to search for. You can replace the name of any city here.
The second line uses the PHP built-in function file_get_contents() to obtain the response data.
The third line converts the response data into JSON format and decodes it using json_decode.
The echo statement in the fourth line outputs the weather data of the queried city.
3. Improve the code
The above code can already obtain weather data, but in order to make it more complete and easy to understand, the code is improved below.
<?php if(isset($_POST['city'])) { $cityName = $_POST['city']; $apikey = "{YOUR_API_KEY}"; $url = "http://api.openweathermap.org/data/2.5/weather?q=".$cityName."&appid=".$apikey."&lang=zh-cn"; $data = file_get_contents($url); $weatherData = json_decode($data); } ?> <!DOCTYPE html> <html> <head> <title>Weather Report Using PHP and OpenWeatherMap API</title> <style> #card { background-color: #F1F1F1; padding: 20px; border-radius: 10px; width: fit-content; margin: 0 auto; margin-top: 10%; } .headtext { margin: 0; padding: 0; font-size: 30px; font-weight: 500; margin-bottom: 10px; } .subtext { margin: 0; padding: 0; font-size: 20px; color: #F44336; } .data { width: 100%; margin-top: 20px; border-top: 1px solid #000; border-collapse: collapse; } .data th { border-bottom: 1px solid #000; padding: 10px; text-align: left; } .data td { padding: 10px; } input[type=text]{ width: 50%; border-radius: 5px; padding: 10px; } button { padding: 10px; background-color: #F44336; border: none; color: #fff; border-radius: 5px; cursor: pointer; } </style> </head> <body> <div id="card"> <p class="headtext">天气预报</p> <form method="post" action=""> <input type="text" name="city" placeholder="输入需要查询的城市" /> <button type="submit">查询</button> </form> <?php if(isset($weatherData)) { ?> <table class="data"> <tr> <th>城市名</th> <th>天气状况</th> <th>温度</th> <th>风速</th> </tr> <tr> <td><?php echo $weatherData->name; ?></td> <td><?php echo $weatherData->weather[0]->description; ?></td> <td><?php echo $weatherData->main->temp; ?> ℃</td> <td><?php echo $weatherData->wind->speed; ?> m/s</td> </tr> </table> <?php } else { ?> <p class="subtext">请输入城市名以获取天气状况。</p> <?php } ?> </div> </body> </html>
This is a more complete weather forecast program, which contains the following parts:
1. Determine whether the user enters a city name, if so, query the weather of the entered city, if not Prompt information is output.
2. Display the weather data of the queried city in the table, including name, weather conditions, temperature and wind speed.
3. Use CSS styles to beautify the page.
4. Ending
That’s it for implementing the use of PHP and OpenWeatherMap API to obtain weather data. As long as you register an OpenWeatherMap account, you can easily obtain an API key and start obtaining real-time weather data. Whether you're building a website, an app, or even need weather data to help you plan, these steps will help you achieve your goals with ease.
The above is the detailed content of How to get weather data using PHP and OpenWeatherMap API. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
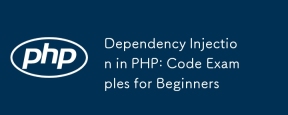
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
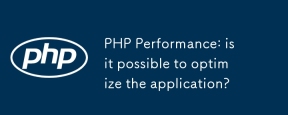
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
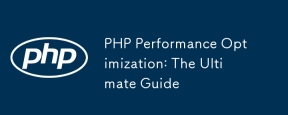
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
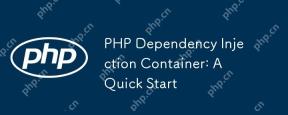
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
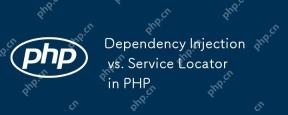
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
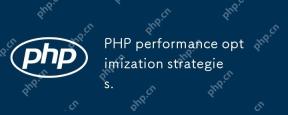
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
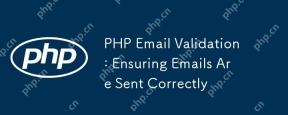
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
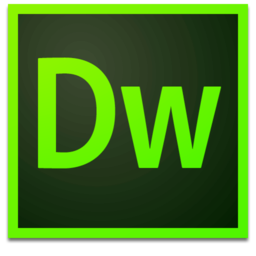
Dreamweaver Mac version
Visual web development tools
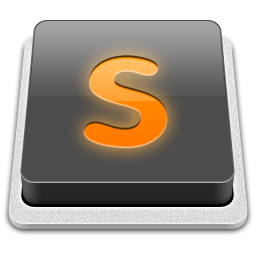
SublimeText3 Mac version
God-level code editing software (SublimeText3)
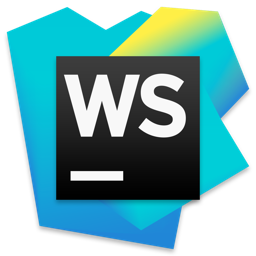
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
