


Python server programming: using Flask-Login to implement user login
Python server programming: Using Flask-Login to implement user login
With the development and popularity of web applications, user login has become an essential function for many applications. In Python server programming, Flask is a widely used web development framework. In addition, Flask also provides many third-party extensions, such as Flask-Login, which can help us quickly implement user login functions.
This article will introduce how to use the Flask-Login extension to implement user login function in Python server. Before that, we need to install Flask and Flask-Login extension. You can use the pip command to install:
pip install Flask Flask-Login
Now let’s implement our user login system.
- Create a Flask application
First, we need to create a Flask application. Create an app.py file in the root directory and write the following code in it:
from flask import Flask app = Flask(__name__) @app.route("/") def index(): return "Hello World!" if __name__ == "__main__": app.run(debug=True)
In this example, we created a Flask application and defined a route. The root route returns "Hello World !". We also use the __name__ == "__main__"
conditional statement to ensure that the Flask application only runs when we run the script.
Now, we have successfully created a simple Flask application.
2. Create a user model
In this example, we assume that the user's username and password have been saved to the database. So we need to define the user model first so that on login we can check if the user exists.
Add the following code in the app.py file:
from flask_login import UserMixin class User(UserMixin): def __init__(self, id): super().__init__() self.id = id def __repr__(self): return f"<User {self.id}>"
In this example, we define a User class, which inherits from Flask-Login’s UserMixin
class . UserMixin
provides some useful properties and methods, including:
-
is_authenticated
: True if the user has been authenticated. -
is_active
: True if the user is active. -
is_anonymous
: True if the user is not authenticated. -
get_id
: Returns the string representation of the user ID.
In the constructor of the User class, we pass in the user's ID. We also define a __repr__
method to see more information about the user object during debugging and testing.
- Writing the Login View
Now, we need to write a login view that lets the user enter their username and password. If the user's username and password are correct, the login is successful and the user object is added to Flask-Login's user session.
Add the following code in the app.py file:
from flask_login import LoginManager, login_user, current_user login_manager = LoginManager() login_manager.init_app(app) @login_manager.user_loader def load_user(user_id): return User(user_id) @app.route("/login") def login(): user_id = request.args.get("user_id") password = request.args.get("password") if user_id and password: # 省略查询数据库的代码 user = User(user_id) login_user(user) return "Login success!" else: return "Login failed!" @app.route("/logout") def logout(): logout_user() return "Logout success!"
In this example, we first create a LoginManager
object. Then, we define a user_loader
callback function that takes the user ID as a parameter and returns the user object corresponding to that ID. In this example, we create a new User
object and pass the ID as a parameter.
Next, we define a login view. In the view function, we use the request.args.get
method to get the user ID and password from the query string. Then we check if the user ID and password are valid. If the user's username and password are correct, a new User
object is created and the object is added to Flask-Login's user session using the login_user
function. If a user's username and password are incorrect, the user cannot log in.
Finally, we define a logout view, which uses the logout_user
function to delete the current user object from Flask-Login's user session and returns a message indicating successful logout.
- Protect other views
Now, we have implemented the user login system, but we are not using it to protect other views. In this example, we will protect a page called "profile" that is only accessible when the user is logged in. If the user is not logged in, they will be redirected to the login page.
Add the following code in the app.py file:
from flask_login import login_required @app.route("/profile") @login_required def profile(): return f"Welcome, {current_user.id}!"
In this example, we use the login_required
decorator to protect the "profile" route. If the user is not logged in, this decorator will redirect to the login page.
When protecting the view, we also use the current_user
global variable to access the information of the currently logged in user. In this example, we add a simple welcome message that contains the ID of the currently logged in user.
- Run the application
Now, we have completed all implementations of the user login system. Let's run the application and test it.
Enter the following command in the terminal to launch the application:
python app.py
Enter the following URL in the browser to access the homepage:
http://localhost:5000/
You should see "Hello World !" string. Now try to access the following URL:
http://localhost:5000/profile
Since you are not an authenticated user, you will be redirected to the login page. Enter a valid user ID and password in the query string, for example:
http://localhost:5000/login?user_id=1&password=password123
If you entered the correct username and password, you should see the "Login success!" message. Now, access the "/profile" route again and it should display a welcome message containing the ID of the currently logged in user.
如果你在浏览器中访问以下URL,则可以注销当前会话:
http://localhost:5000/logout
这样就完成了用户登录系统的所有实现。
总结
在本文中,我们介绍了如何使用Flask-Login扩展在Python服务器中实现用户登录功能。使用Flask-Login可以方便地验证和保护Web应用程序中的用户数据。此外,Flask-Login提供了许多有用的功能和方法,如会话管理和用户保护。希望这篇文章能够帮助你更好地了解如何使用Flask-Login编写Python服务器应用程序。
The above is the detailed content of Python server programming: using Flask-Login to implement user login. For more information, please follow other related articles on the PHP Chinese website!
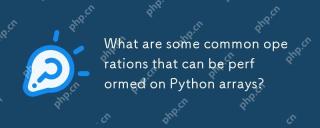
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
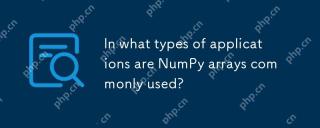
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
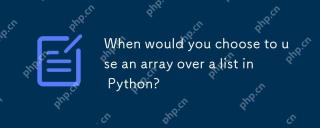
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf
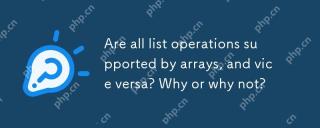
No,notalllistoperationsaresupportedbyarrays,andviceversa.1)Arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,whichimpactsperformance.2)Listsdonotguaranteeconstanttimecomplexityfordirectaccesslikearraysdo.
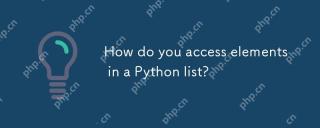
ToaccesselementsinaPythonlist,useindexing,negativeindexing,slicing,oriteration.1)Indexingstartsat0.2)Negativeindexingaccessesfromtheend.3)Slicingextractsportions.4)Iterationusesforloopsorenumerate.AlwayschecklistlengthtoavoidIndexError.
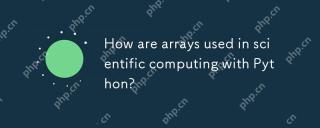
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
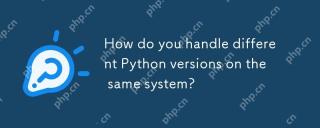
You can manage different Python versions by using pyenv, venv and Anaconda. 1) Use pyenv to manage multiple Python versions: install pyenv, set global and local versions. 2) Use venv to create a virtual environment to isolate project dependencies. 3) Use Anaconda to manage Python versions in your data science project. 4) Keep the system Python for system-level tasks. Through these tools and strategies, you can effectively manage different versions of Python to ensure the smooth running of the project.
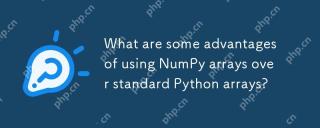
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
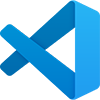
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
