Detailed explanation of caching mechanism in Django framework
In web applications, caching is usually an important means to optimize performance. As a well-known web framework, Django naturally provides a complete caching mechanism to help developers further improve application performance.
This article will provide a detailed explanation of the caching mechanism in the Django framework, including cache usage scenarios, recommended caching strategies, cache implementation and usage, etc. I hope it will be helpful to Django developers or readers who are interested in the caching mechanism.
1. Cache usage scenarios
Cache usage scenarios vary depending on the application. Among them, the most common ones are data with high reading frequency, slow data change or unchanged data. This type of data is usually static resources, configuration information, data dictionaries, etc. In large-scale web applications, due to the large amount of data access, if each request requires querying the database or performing additional calculations, the access speed will inevitably decrease. Caching can store this data in memory or other fast storage media to quickly respond to requests.
In addition, caching can also be used to handle large-traffic concurrent requests. For some computationally intensive operations, if the number of concurrent requests is too large, thread blocking or process crash may occur. At this time, by using cache, the results can be cached to avoid repeated calculations and waste of resources.
2. Recommended caching strategy
When designing an application caching strategy, developers need to make trade-offs based on the actual situation of the application. Listed below are some recommended caching strategies during development:
- Cache time
The cache time should be determined based on the frequency of cached data changes and the cache usage scenario. For some scenarios where the data will not change, you can use a longer cache time, such as one day or a week, to reduce the cost of cache updates. For some data that needs to be updated in real time, a relatively short cache time can be used, such as a few seconds or minutes.
- Cache scope
The cache scope is generally divided into global cache and local cache. For global cache, relatively stable data can be cached in memory or other storage media to reduce the overhead of repeated database queries. For some data that is frequently operated but relatively stable, you can use local caching and store it in the cache to reduce query overhead and improve response speed.
- Cache Cleaning
Cache cleaning is the key to ensuring the validity of cached data. Because the data stored in the cache may change at any time, if it is not cleaned up in time, the cache data may be inconsistent or invalid. Generally speaking, you can choose to clean the cache when the data changes, or when the cache time expires.
3. How to implement cache
The Django framework provides a variety of cache backends, including memory cache, file cache, database cache, etc. Developers can choose the appropriate cache backend according to the actual situation and implement cache. The following is a brief introduction to several commonly used cache implementation methods.
- Memory cache
Memory cache is Django’s default caching backend and the most widely used backend. It uses memory to store cached data, has a very fast response speed and is suitable for storing some temporary data. At the same time, it also supports functions such as cache time and cache key version number, which facilitates developers to perform data cleaning and data version management.
- File caching
File caching uses the file system to store cache data. Compared with the memory cache, it can store a larger amount of data, and the data can be persisted to disk. However, the response speed is relatively low, disk IO operations are required, and system resources are consumed.
- Database cache
Database cache uses a database to store cached data. Compared with file caching, it can achieve higher data persistence and flexibility. The disadvantage is that the response speed is relatively low, database IO operations are required, and it may also cause excessive database pressure.
4. How to use cache
In the Django framework, the use of cache is very simple. You only need to perform the following operations:
- Install django-cacheops library
In order to use caching more conveniently, we can use the django-cacheops library, which extends Django's caching mechanism and provides more caching functions. You can add the following dependencies in the project's requirements.txt:
django-cacheops==6.0.2
Then execute the command to install the dependencies:
pip install -r requirements. txt
- Configure the cache backend
Configure the cache backend in Django’s settings.py file, as follows:
CACHES = {
'default': { 'BACKEND': 'django.core.cache.backends.memcached.MemcachedCache', 'LOCATION': '127.0.0.1:11211', }
}
In the above configuration, the Memcached cache backend is used, the cache server address is 127.0.0.1, and the port number is 11211. The specific cache configuration will be modified according to the actual situation.
- Using caching
During the development process, we can use Django’s own cache module to perform caching operations. For example, to use cache in a view function, you can do the following:
from django.shortcuts import render
from django.core.cache import cache
def my_view(request):
value = cache.get('my_key') if value is None: value = expensive_calculation() cache.set('my_key', value, timeout=3600) return render(request, 'my_template.html', {'value': value})
在上述代码中,我们首先使用cache.get()方法从缓存中获取数据。如果数据不存在,我们就进行耗时计算并将其存储到缓存中,然后再返回结果。其中,timeout参数指定了缓存时间,单位是秒。
可以看到,使用Django缓存,可以大大简化程序的编写和优化过程。
总结
本文简要介绍了Django框架中的缓存机制,包括缓存的使用场景、建议的缓存策略、缓存的实现方式和使用方法等方面。希望能够对Django开发者或对缓存机制感兴趣的读者有所帮助。最后提醒开发者在使用缓存时要谨慎,根据实际情况进行合理的缓存策略设计,避免不必要的性能问题和数据不一致的情况发生。
The above is the detailed content of Detailed explanation of caching mechanism in Django framework. For more information, please follow other related articles on the PHP Chinese website!
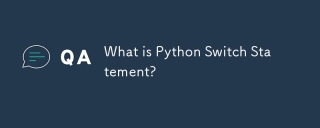
The article discusses Python's new "match" statement introduced in version 3.10, which serves as an equivalent to switch statements in other languages. It enhances code readability and offers performance benefits over traditional if-elif-el
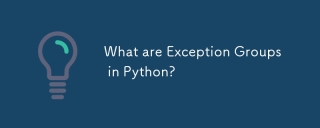
Exception Groups in Python 3.11 allow handling multiple exceptions simultaneously, improving error management in concurrent scenarios and complex operations.
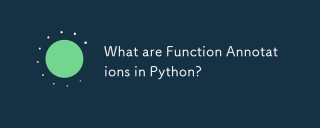
Function annotations in Python add metadata to functions for type checking, documentation, and IDE support. They enhance code readability, maintenance, and are crucial in API development, data science, and library creation.
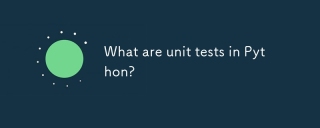
The article discusses unit tests in Python, their benefits, and how to write them effectively. It highlights tools like unittest and pytest for testing.
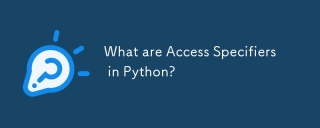
Article discusses access specifiers in Python, which use naming conventions to indicate visibility of class members, rather than strict enforcement.
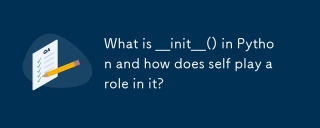
Article discusses Python's \_\_init\_\_() method and self's role in initializing object attributes. Other class methods and inheritance's impact on \_\_init\_\_() are also covered.
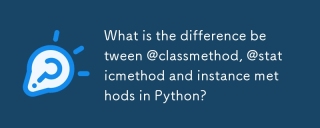
The article discusses the differences between @classmethod, @staticmethod, and instance methods in Python, detailing their properties, use cases, and benefits. It explains how to choose the right method type based on the required functionality and da
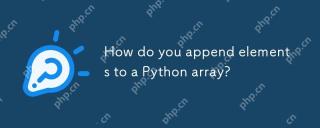
InPython,youappendelementstoalistusingtheappend()method.1)Useappend()forsingleelements:my_list.append(4).2)Useextend()or =formultipleelements:my_list.extend(another_list)ormy_list =[4,5,6].3)Useinsert()forspecificpositions:my_list.insert(1,5).Beaware


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
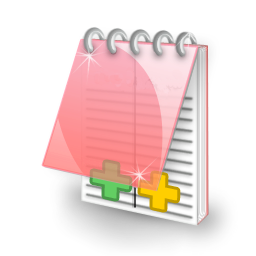
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
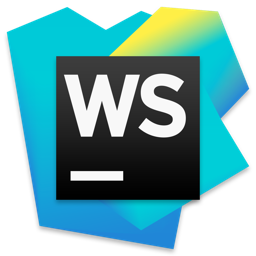
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
