In Java API development, unit testing is a very important part. Unit testing can help us detect and repair unreasonable program design during the code development process, reduce bugs in the code, and improve the quality of the program. In order to better control unit testing, quickly execute and targeted testing, it is also extremely necessary to use unit testing tools.
PowerMock is a powerful unit testing framework that focuses on Java API development – it can simulate some scenarios that we usually cannot simulate, such as static methods, private methods, etc., allowing us to Cover the code execution path more comprehensively and improve code quality.
This article will introduce the basic use of PowerMock and its application in unit testing.
1. Introduction of PowerMock
Before starting to use PowerMock, you need to introduce the following dependencies in the project's pom.xml file:
<dependency> <groupId>org.powermock</groupId> <artifactId>powermock-core</artifactId> <version>2.0.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.powermock</groupId> <artifactId>powermock-module-junit4</artifactId> <version>2.0.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.powermock</groupId> <artifactId>powermock-api-mockito2</artifactId> <version>2.0.2</version> <scope>test</scope> </dependency>
It should be noted here that due to PowerMock The scope of use is only valid in the test environment, so these dependencies need to be declared in the scope of test
.
Next, let’s take a look at the basic usage of PowerMock!
2. Basic usage of PowerMock
- Mock static method
It is very common to call static methods in Java. In unit testing, we It is also necessary to test the static methods in the code. At this time, we will use PowerMock to simulate the execution of the static method, for example:
public class MyClass { public static String staticMethod() { return "staticMethod result"; } }
Test code example of using PowerMock to simulate the static method:
@RunWith(PowerMockRunner.class) @PrepareForTest(MyClass.class) public class MyClassTest { @Test public void testStaticMethod() { PowerMockito.mockStatic(MyClass.class); when(MyClass.staticMethod()).thenReturn("mock result"); String result = MyClass.staticMethod(); Assert.assertEquals(result, "mock result"); } }
In the above code, we simulate the static method through the when
method and return the result we set. Then when calling MyClass.staticMethod()
, the result will be the "mock result" we set, not the actual "staticMethod result".
- Mock Constructor
In some scenarios, we need to simulate the constructor of a certain class. In this case, we can use PowerMockito.whenNew
method to replace its constructor implementation, for example:
public class MyClass { public MyClass() { // ... } } public class MyService { public MyClass createInstance() { return new MyClass(); } }
Use PowerMock to simulate the test code example of the constructor:
@RunWith(PowerMockRunner.class) public class MyServiceTest { @Test public void testCreateInstance() throws Exception { MyClass mockMyClass = PowerMockito.mock(MyClass.class); PowerMockito.whenNew(MyClass.class).withNoArguments().thenReturn(mockMyClass); MyService myService = new MyService(); MyClass instance = myService.createInstance(); Assert.assertEquals(mockMyClass, instance); } }
We replace it through the PowerMockito.whenNew
method The MyClass
constructor is implemented so that it returns the result we simulated. In this way, when MyService.createInstance()
is called, the MyClass
instance obtained is the mock object we set, which facilitates our unit testing.
3. Summary
This article briefly introduces the basic usage of PowerMock and its application in unit testing. Using PowerMock allows us to have more comprehensive control capabilities in unit testing, improving Code quality and reduced repetitive manual testing. Although PowerMock brings more testing ideas and optimization space, you must also be careful not to overuse it while avoiding leaking simulations. You should follow the principle of simplicity to facilitate subsequent code maintenance and reconstruction.
The above is the detailed content of Using PowerMock for unit testing in Java API development. For more information, please follow other related articles on the PHP Chinese website!
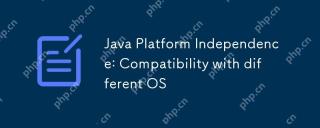
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
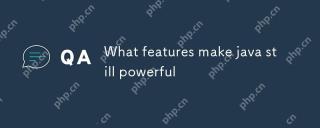
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
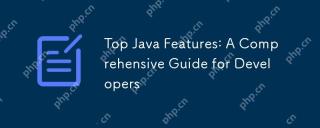
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
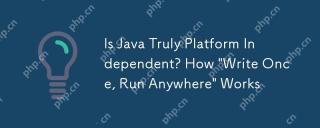
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
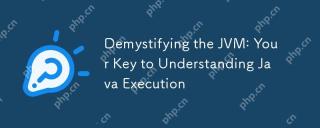
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
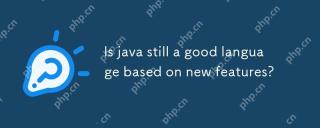
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
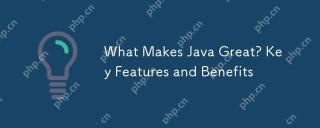
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
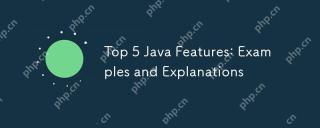
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
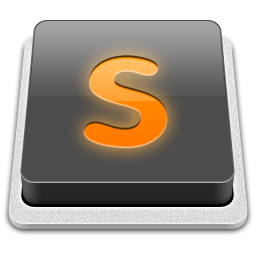
SublimeText3 Mac version
God-level code editing software (SublimeText3)
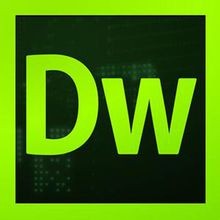
Dreamweaver CS6
Visual web development tools
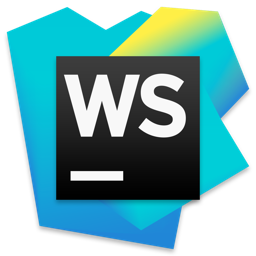
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
