Using RabbitMQ for asynchronous message processing in Java API development
With the rapid development of the Internet, asynchronous message processing plays an important role in distributed systems, which can improve the reliability and concurrency of the system. RabbitMQ is an open source message queue system that can deliver messages quickly and reliably and is widely used in the Internet field. This article will introduce how to use RabbitMQ for asynchronous message processing in Java API development.
1. Introduction to RabbitMQ
RabbitMQ is an open source message queue middleware based on AMQP (Advanced Message Queuing Protocol). It is written in Erlang language and has the characteristics of fast, reliable and scalable. RabbitMQ supports multiple programming languages, including Java, Python, Ruby, etc., allowing developers to use it conveniently.
2. Basic concepts of RabbitMQ
1. Message queue
The message queue is a FIFO (first in, first out) data structure used to store and transmit messages. In RabbitMQ, the message queue is called "Queue".
2. Message
Message is the carrier of information transmission and can contain any type of data. In RabbitMQ, messages are called "Messages".
3. Switch
The switch is the center of message routing, sending messages to the corresponding queue according to rules. In RabbitMQ, the exchange is called "Exchange".
4. Binding
Binding is the operation of connecting queues and switches together, and specific routing rules can be specified. Typically, bindings and queues specify a routing key so that the switch can route messages to the correct queue.
5. Consumer
Consumer is a program that receives and processes messages. It can take messages out of the queue and perform some operations. In RabbitMQ, consumers are called "Consumers".
3. RabbitMQ usage process
Before using RabbitMQ, you need to set up a RabbitMQ server. For installation instructions, please refer to the official documentation. The basic process is as follows:
1. Create a connection factory object and set the RabbitMQ server address and port number.
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
factory.setPort(5672);
2. Create a connection object.
Connection connection = factory.newConnection();
3. Create a channel (Channel) object.
Channel channel = connection.createChannel();
4. Create an Exchange object.
channel.exchangeDeclare("exchange_name", "direct", true);
5. Create a queue (Queue) object.
channel.queueDeclare("queue_name", true, false, false, null);
6. Bind the queue and switch.
channel.queueBind("queue_name", "exchange_name", "routing_key");
7. Create a consumer (Consumer) object and set the consumption callback function.
Consumer consumer = new DefaultConsumer(channel) {
@Override public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException { // 处理消息 }
};
channel.basicConsume("queue_name", true, consumer);
8.Publish message .
channel.basicPublish("exchange_name", "routing_key", null, message.getBytes());
4. Use RabbitMQ for asynchronous message processing
In Java API During development, there are many scenarios for using RabbitMQ for asynchronous message processing. For example, when a user submits a task request to the system, the request can be packaged into a message and submitted to the RabbitMQ queue. The system can then process the task during idle time and send the processing results to another queue. Finally, another part of the program can get the processing results from the queue and return them to the user.
1. Create a connection factory object and set the RabbitMQ server address and port number.
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
factory.setPort(5672);
2. Create a connection object.
Connection connection = factory.newConnection();
3. Create a channel (Channel) object.
Channel channel = connection.createChannel();
4. Create an Exchange object.
channel.exchangeDeclare("exchange_name", "direct", true);
5. Create a request queue (Queue) object.
channel.queueDeclare("request_queue", true, false, false, null);
6. Bind the request queue and switch.
channel.queueBind("request_queue", "exchange_name", "request_routing_key");
7. Create a consumer (Consumer) object and set the consumption callback function.
Consumer consumer = new DefaultConsumer(channel) {
@Override public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException { // 处理请求消息 String response = processMessage(new String(body)); // 将处理结果发送到响应队列 channel.basicPublish("exchange_name", "response_routing_key", null, response.getBytes()); }
};
channel.basicConsume("request_queue", true, consumer);
8. Create a response Queue object.
channel.queueDeclare("response_queue", true, false, false, null);
9. Bind the response queue and switch.
channel.queueBind("response_queue", "exchange_name", "response_routing_key");
10. Send the request message to the request queue.
channel.basicPublish("exchange_name", "request_routing_key", null, requestMessage.getBytes());
11. Wait for the response message.
Consumer responseConsumer = new DefaultConsumer(channel) {
@Override public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException { // 处理响应消息 }
};
channel.basicConsume("response_queue", true, responseConsumer);
5. Summary
This article introduces the basic process of using RabbitMQ for asynchronous message processing in Java API development. RabbitMQ can achieve reliable message delivery and provides an efficient asynchronous message processing method for the system. In actual development, different configurations need to be selected according to different business scenarios to ensure system reliability and performance.
The above is the detailed content of Using RabbitMQ for asynchronous message processing in Java API development. For more information, please follow other related articles on the PHP Chinese website!
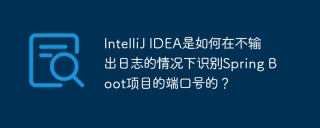
Start Spring using IntelliJIDEAUltimate version...
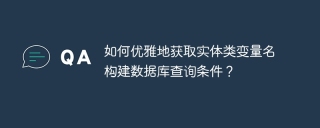
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
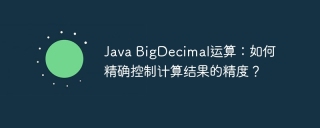
Java...
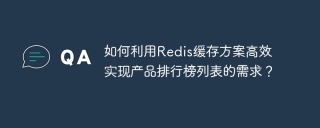
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
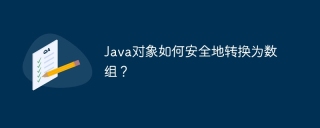
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
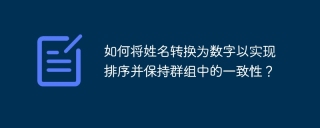
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
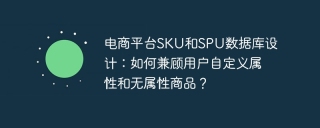
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
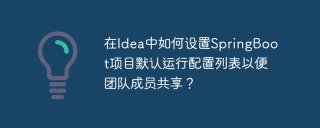
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
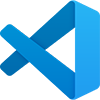
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
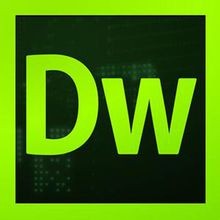
Dreamweaver CS6
Visual web development tools