Java’s own training strategy and incremental learning technology
In recent years, machine learning and artificial intelligence technology have continued to develop, and more and more application scenarios have emerged, such as natural language processing and image recognition. , intelligent recommendation, etc., there are also more and more engineers engaged in work in related fields. However, in practical applications, we often encounter some problems, such as the small amount of original data, the continuous accumulation of new data, and the insufficient stability of the training model. This article will introduce an own training strategy and incremental learning technology implemented in Java to solve the above problems and improve model stability and accuracy.
1. Self-training strategy
The self-training strategy refers to dividing the original data set into several mutually exclusive subsets, and then using the cross-validation method to use each subset as a test set, and the remaining The subset is used as a training set to train and test the model. Finally, the results of each training and test are combined to obtain the final model. The advantage of this is to make full use of the original data and improve the accuracy and stability of the model through continuous training and testing. In addition, after each training and testing, we can also adjust the model parameters based on the results to further improve model performance.
The specific implementation method is as follows:
- Randomly divide the original data set into k mutually exclusive subsets.
- Using the cross-validation method, each subset is verified separately, and the remaining subsets are used to train the model.
- After each training and test, the model parameters are adjusted based on the results to further improve the accuracy and stability of the model.
The code is implemented as follows:
public class SelfTraining { private int k; private List<List<Data>> subsets; private Model model; public void train(List<Data> data, Model model, int k) { this.k = k; this.subsets = splitData(data, k); this.model = model; double bestAccuracy = 0; Model bestModel = null; for (int i = 0; i < k; i++) { List<Data> trainData = new ArrayList<>(); List<Data> testData = subsets.get(i); for (int j = 0; j < k; j++) { if (j != i) { trainData.addAll(subsets.get(j)); } } model.train(trainData); double accuracy = model.test(testData); if (accuracy > bestAccuracy) { bestAccuracy = accuracy; bestModel = model.clone(); } } this.model = bestModel; } private List<List<Data>> splitData(List<Data> data, int k) { List<List<Data>> subsets = new ArrayList<>(); int subsetSize = data.size() / k; for (int i = 0; i < k; i++) { List<Data> subset = new ArrayList<>(); for (int j = 0; j < subsetSize; j++) { int index = i * subsetSize + j; subset.add(data.get(index)); } subsets.add(subset); } return subsets; } }
2. Incremental learning technology
Incremental learning technology refers to the continuous introduction of existing models on the basis of New data is trained and updated to achieve a dynamic learning and optimization process. Compared with retraining the entire model, incremental learning technology can significantly improve model training efficiency and accuracy. In addition, in the face of increasing data volume or changing features, incremental learning technology can better adapt to scene changes.
The specific implementation method is as follows:
- Load the existing model and import the original training data.
- When new data arrives, add the new data to the original training data to ensure that the features and labels of the original data and the new data are consistent.
- Train on new data and update model parameters based on the results.
- Save and back up the updated model for subsequent use.
The code is implemented as follows:
public class IncrementalLearning { private Model model; public void train(List<Data> newData) { List<Data> allData = loadOldData(); allData.addAll(newData); model.train(allData); saveModel(model); } private List<Data> loadOldData() { // load old training data from disk or database return Collections.emptyList(); } private void saveModel(Model model) { // save model to disk or database } private Model loadModel() { // load model from disk or database return new Model(); } public void update() { List<Data> newData = loadNewData(); this.model = loadModel(); train(newData); backupModel(this.model); } private List<Data> loadNewData() { // load new data from disk or network return Collections.emptyList(); } private void backupModel(Model model) { // backup model to disk or database } }
3. Conclusion
Self-training strategy and incremental learning technology are two commonly used machine learning optimization technologies. In many are of great significance in practical applications. This article introduces the basic concepts, implementation steps and Java code implementation of the two technologies. Readers can choose suitable technologies and implementation methods according to their actual situations, and continuously improve and optimize them in specific practice.
The above is the detailed content of Own training strategy and incremental learning technology implemented in Java. For more information, please follow other related articles on the PHP Chinese website!
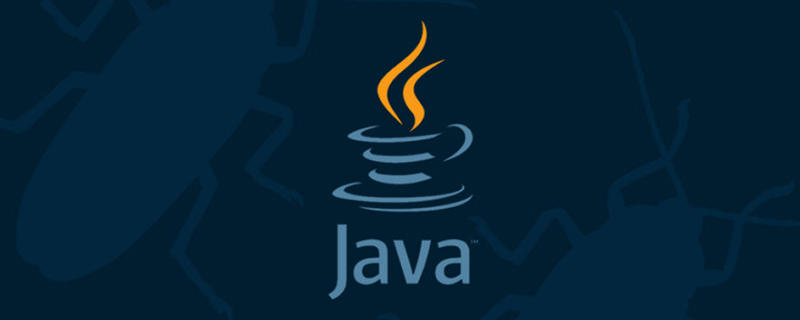
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
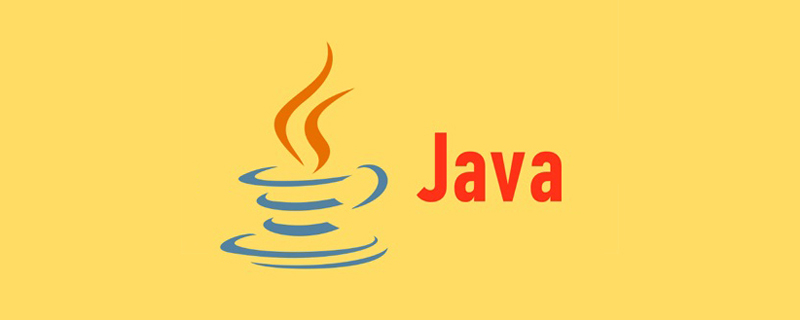
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
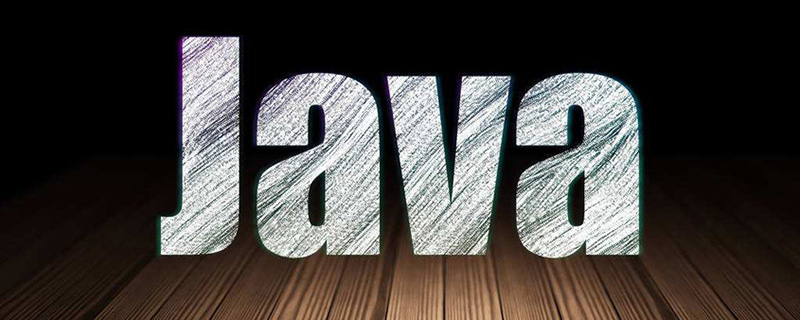
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
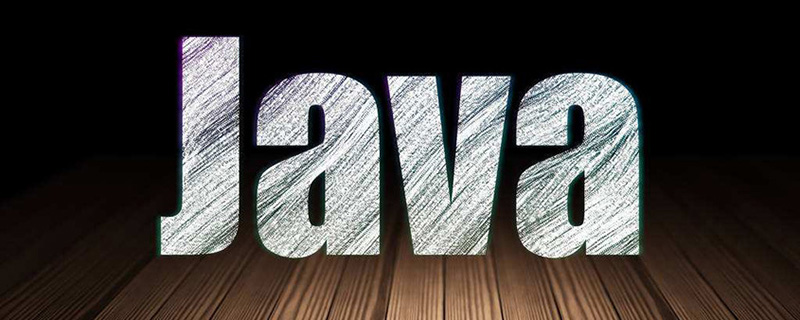
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
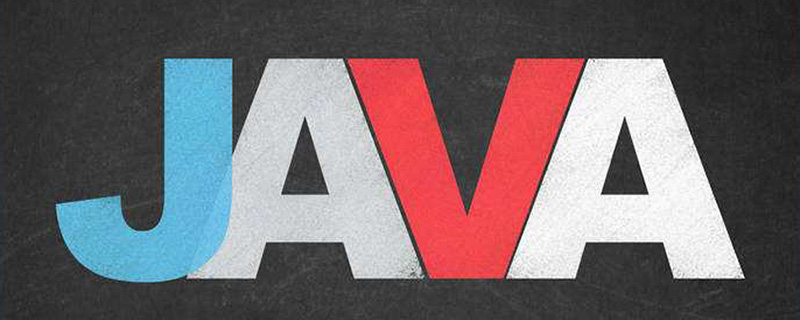
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
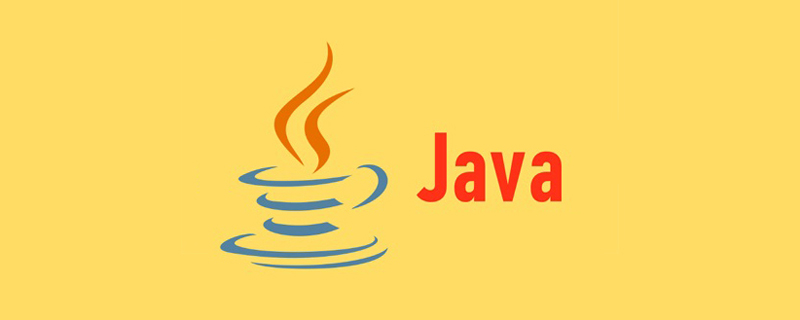
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
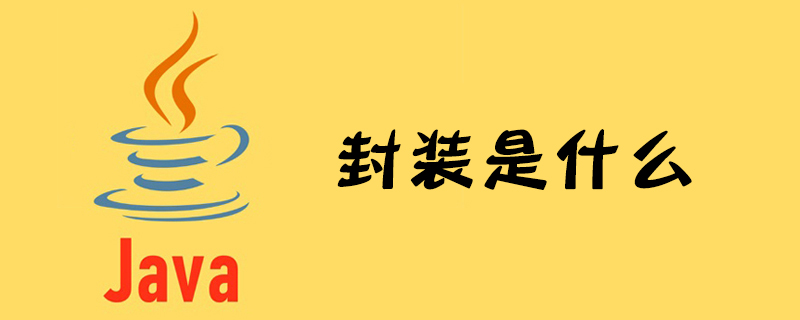
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
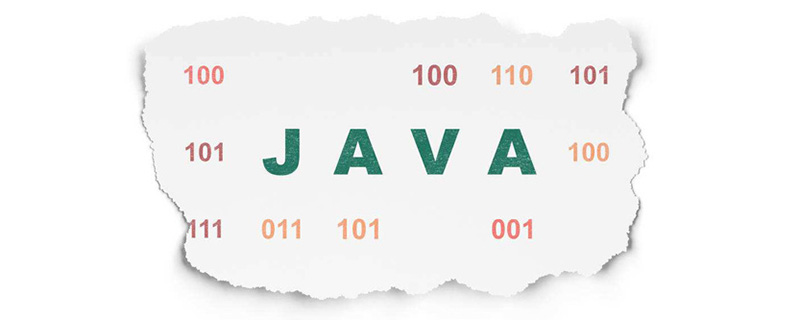
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
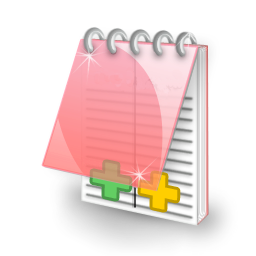
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
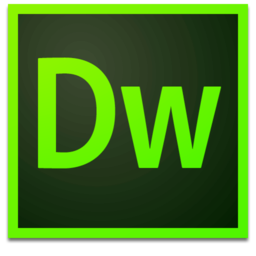
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
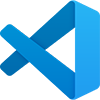
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
