With the continuous development of Internet technology, real-time communication has become an indispensable part of many application systems. In the field of Web applications, WebSocket technology has become one of the main means of real-time communication. On the Java platform, the Java API also provides a set of WebSocket APIs, which can facilitate WebSocket development.
This article will focus on how to use WebSocket API for real-time communication in Java API development. In the process of implementing WebSocket communication, we will explain it in the following aspects:
- Introduction to WebSocket protocol
- Basic use of WebSocket API
- WebSocket API thread model
- Advanced usage scenarios of WebSocket API
1. Introduction to WebSocket protocol
The WebSocket protocol is a new protocol in HTML5, which implements full-duplex communication between the browser and the server. Before the emergence of the WebSocket protocol, the communication between the browser and the server was based on the HTTP protocol. The HTTP protocol was a half-duplex communication, that is, it could only communicate in one way, and the server could not actively send messages to the client. The WebSocket protocol allows true two-way communication between the server and the client.
An important feature of the WebSocket protocol is that it is based on the TCP protocol. When establishing a WebSocket connection, the client and server will first perform a TCP handshake, and then encapsulate the WebSocket protocol data in an HTTP protocol data frame to achieve two-way communication.
2. Basic use of WebSocket API
The WebSocket API in Java API is included in the javax.websocket package, which provides a set of WebSocket standard API interfaces and related class libraries, which can be used Used to implement various operations of WebSocket communication.
The following is the basic usage process of WebSocket API:
- Creating a WebSocket server
You need to define a server endpoint through which a WebSocket connection can be established. and receive and send messages. This endpoint needs to inherit the javax.websocket.Endpoint class and implement key methods such as onOpen, onClose, onMessage, and onError.
- Creating a WebSocket client
You need to specify the address of the WebSocket server and use the WebSocket client object to connect to the server.
- Send a message
WebSocket communication is based on messages. You can use the send method provided by the javax.websocket.Session interface to send messages.
- Receive messages
The logic of message reception needs to be implemented in both the server and the client. You can override the onMessage method to implement the corresponding logic.
The following is an example of using the WebSocket API:
Server-side code:
@ServerEndpoint("/server") public class WebSocketServer { @OnOpen public void onOpen(Session session) { System.out.println("WebSocket opened: " + session.getId()); } @OnClose public void onClose(Session session) { System.out.println("WebSocket closed: " + session.getId()); } @OnMessage public void onMessage(String message, Session session) { System.out.println("WebSocket received message: " + message); try { session.getBasicRemote().sendText("Server received message: " + message); } catch (IOException ex) { ex.printStackTrace(); } } @OnError public void onError(Session session, Throwable throwable) { System.out.println("WebSocket error: " + throwable.getMessage()); } }
Client-side code:
URI uri = new URI("ws://localhost:8080/server"); WebSocketContainer container = ContainerProvider.getWebSocketContainer(); Session session = container.connectToServer(MyClient.class, uri); session.getBasicRemote().sendText("Hello Server!");
Note: In the client, MyClient .class needs to implement the javax.websocket.ClientEndpoint interface and override the onMessage method.
3. WebSocket API thread model
The thread model in WebSocket API is quite special. WebSocket communication is built on Web containers (such as Tomcat, Jetty, etc.), so the thread model in the WebSocket API also depends on the thread model of the Web container. Since WebSocket creates some fixed thread pools in the Web container, special attention needs to be paid to thread safety issues.
WebSocket API provides two annotations: @OnOpen and @OnClose, which can be used to perform related logical operations when establishing and disconnecting WebSocket connections. In these two callback methods, if database operations or other time-consuming operations are required, the connection should be released immediately, otherwise the performance of the web container may be affected.
4. Advanced usage scenarios of WebSocket API
In addition to the above basic usage scenarios, WebSocket API also provides some advanced usage scenarios, such as using annotations to write endpoints and using interceptors to write Endpoints, writing endpoints programmatically, and more.
Due to space limitations, this article cannot introduce these advanced usage scenarios in detail. Readers can study it in combination with official documents.
Summary
This article mainly introduces how to use WebSocket API for real-time communication in Java API development, including an introduction to the WebSocket protocol, the basic use of WebSocket API, and the threading model of WebSocket API and advanced usage scenarios for the WebSocket API. By studying this article, readers can have a preliminary understanding of the programming ideas and implementation methods of WebSocket API, and then better cope with the development needs of real-time communication.
The above is the detailed content of Using WebSocket API for real-time communication in Java API development. For more information, please follow other related articles on the PHP Chinese website!
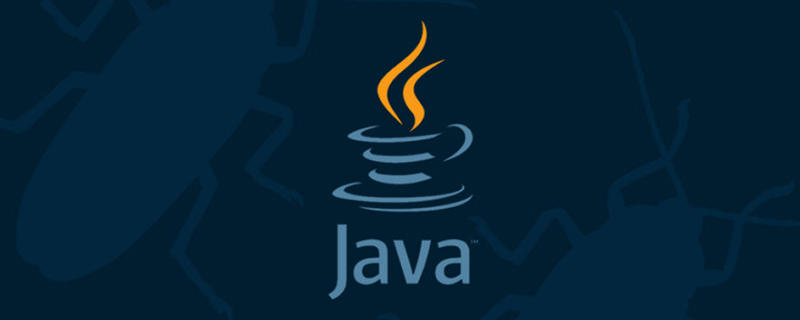
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
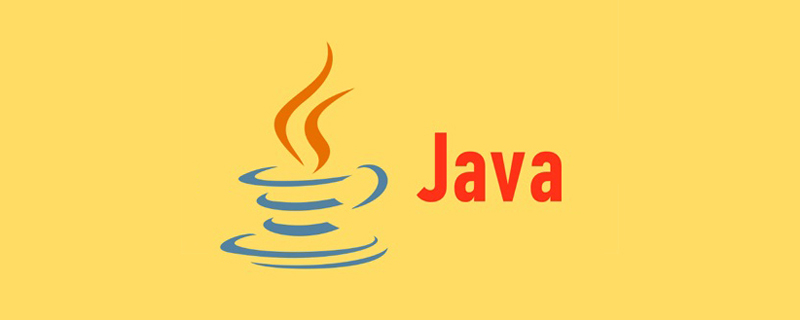
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
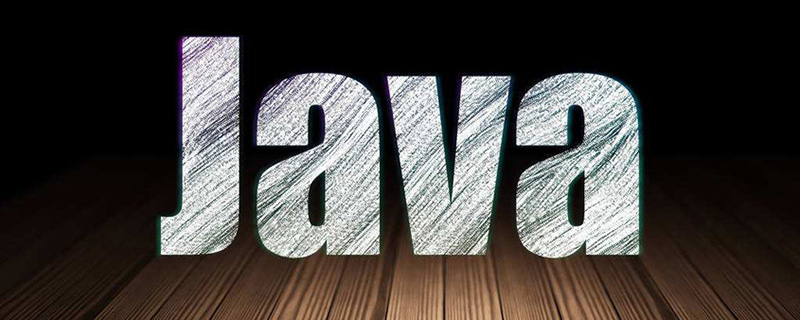
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
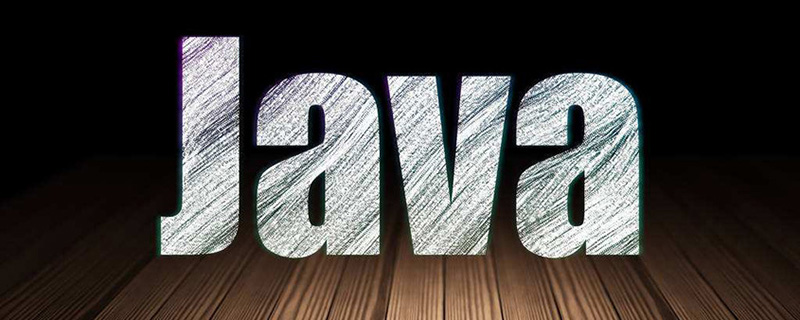
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
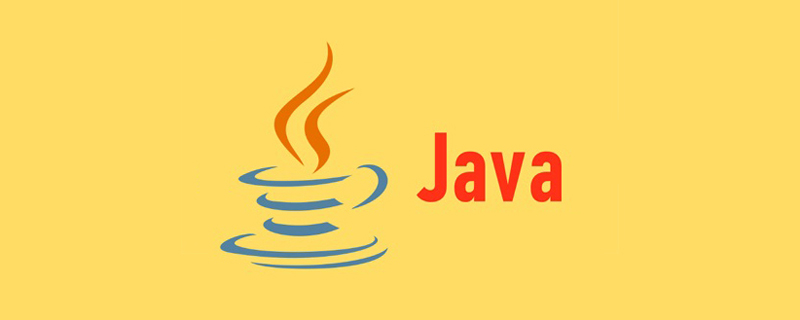
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
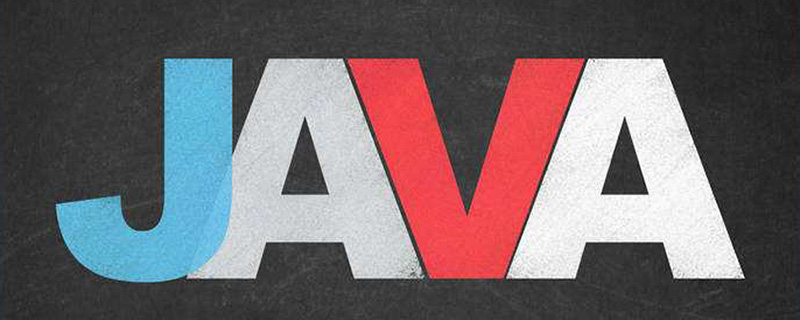
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
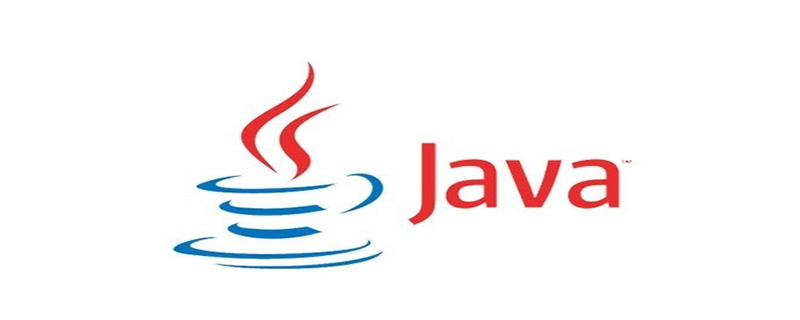
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
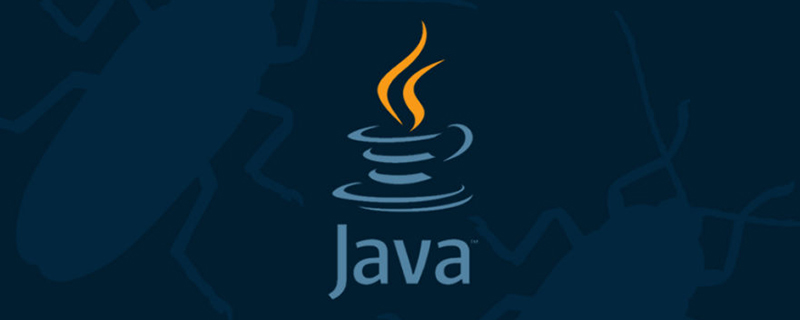
本篇文章给大家带来了关于Java的相关知识,其中主要整理了Stream流的概念和使用的相关问题,包括了Stream流的概念、Stream流的获取、Stream流的常用方法等等内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),