Using JAX-RS for Web service processing in Java API development
Java API development is a widely used programming method, and there are many factors behind its success, one of which is web service processing. Web services processing can use the Java API for RESTful Web Services (JAX-RS), a Java framework for implementing RESTful Web services. In this article, we'll explore the basic concepts, architecture, and usage of JAX-RS.
What is RESTful Web Services
Before we start introducing JAX-RS, we need to understand the basic concepts of RESTful Web services. REST is the abbreviation of Representational State Transfer, which means presentation layer state transfer. RESTful Web service is a service based on HTTP protocol. It uses Uniform Resource Identifier (URI) to represent resources and uses HTTP methods (GET, POST, PUT, DELETE) to operate on resources. A RESTful web service is a lightweight service that can easily interact with other web applications because it uses the HTTP protocol.
JAX-RS Architecture
The JAX-RS framework is implemented based on the Java Servlet API, which allows you to use Java classes and annotations to define RESTful web services. The core of the framework is an HTTP server that receives HTTP requests from clients and converts them into Java objects. In JAX-RS, resources are the core of RESTful web services, and resources are a collection of methods. These methods handle HTTP requests.
The JAX-RS framework consists of two main parts: JAX-RS API and JAX-RS implementation. The JAX-RS API is a Java interface that defines the JAX-RS specification, and a JAX-RS implementation is any framework that implements the JAX-RS specification. There are currently many JAX-RS implementations, including Jersey, CXF, RESTeasy, etc.
JAX-RS Annotations
JAX-RS mainly defines RESTful web services through annotations. Annotations are a technique used to extract metadata out of Java code. The following are the most commonly used annotations for JAX-RS:
- @Path: Specifies the path of the resource. For example, @Path("/books") indicates that the path of the resource is /books.
- @GET, @POST, @PUT, @DELETE: Specify the HTTP method.
- @Produces, @Consumes: Specify the media type of the request and response.
- @QueryParam, @PathParam, @FormParam: Specify query parameters, path parameters and form parameters.
JAX-RS Example
Below we will use Jersey to implement a simple RESTful Web service. This service will handle two requests, one to get all books and the other to get a single book based on its book ID. We will use @Path, @GET, @Produces annotations to implement this service.
First, we need to create a Book class, which has two attributes: id and title. Then, we need to create a BookResource class, which is marked with the @Path("/books") annotation and contains two methods: getAllBooks() and getBookById(). In the getAllBooks() method, we use the @GET and @Produces annotations to specify the HTTP method and the media type of the response. In the getBookById() method, we use the @GET, @Path, and @Produces annotations to specify the HTTP method, request path, and response media type.
public class Book { private int id; private String title; public Book(int id, String title) { this.id = id; this.title = title; } public int getId() { return id; } public String getTitle() { return title; } } @Path("/books") public class BookResource { private static List<Book> bookList = new ArrayList<>(); static { bookList.add(new Book(1, "Java SE 8")); bookList.add(new Book(2, "Java EE 7")); bookList.add(new Book(3, "Spring 5")); } @GET @Produces(MediaType.APPLICATION_JSON) public List<Book> getAllBooks() { return bookList; } @GET @Path("/{id}") @Produces(MediaType.APPLICATION_JSON) public Book getBookById(@PathParam("id") int id) { return bookList.stream().filter(b -> b.getId() == id).findFirst().orElse(null); } }
Finally, we need to create a startup class to run this service on port 8080 of the local host localhost. We use the URI /api to specify the path to this service. For example, a request to get all books is http://localhost:8080/api/books, and a request to get book ID 1 is http://localhost:8080/api/books/1.
public class Application extends ResourceConfig { public Application() { packages("com.example.web"); } public static void main(String[] args) throws Exception { URI baseUri = UriBuilder.fromUri("http://localhost/").port(8080).build(); ResourceConfig config = new Application(); HttpServer server = JdkHttpServerFactory.createHttpServer(baseUri, config, false); Runtime.getRuntime().addShutdownHook(new Thread(server::stop)); server.start(); } }
Summary
JAX-RS provides a convenient way to create RESTful web services and integration with Java applications is very easy. The JAX-RS API provides a set of annotations and classes to define RESTful web services, and the JAX-RS implementation translates these specifications into actual Java code. Using JAX-RS makes it easier to create and deploy RESTful web services, thereby improving application availability and scalability.
The above is the detailed content of Using JAX-RS for Web service processing in Java API development. For more information, please follow other related articles on the PHP Chinese website!
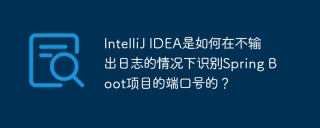
Start Spring using IntelliJIDEAUltimate version...
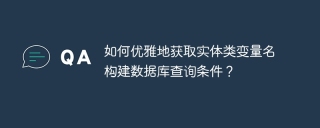
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
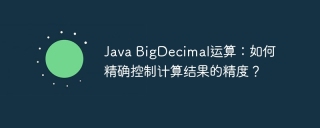
Java...
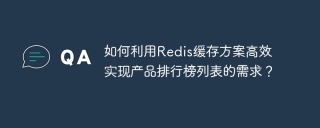
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
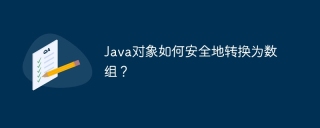
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
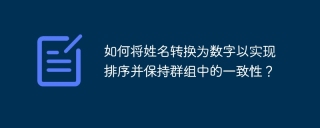
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
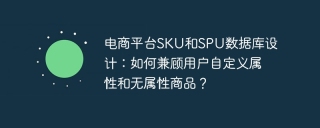
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
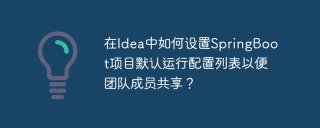
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
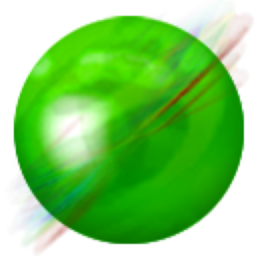
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
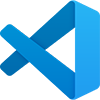
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment