Using Netty5 for TCP communication in Java API development
In Java API development, TCP communication is a very important component, and Netty5 is a high-performance network communication framework based on NIO, which can handle complex network communication tasks very conveniently. This article will introduce how to use Netty5 for TCP communication, including the core components of Netty5, an introduction to common APIs, and practical application cases. At the same time, this article will also introduce how to use Netty5 to improve the performance and reliability of TCP communication.
1. The core components of Netty5
The core components of Netty5 include Channel, EventLoop, Codec, Handler and Bootstrap. Among them, Channel represents an open connection that can read and write data. EventLoop is the thread pool used to handle all events in Netty5. Codec is a set of codecs responsible for converting data from bytecode to objects, and objects to bytecode. Handler is one of the most important components in Netty5, responsible for handling connection status, read and write events, and exception events. Finally, Bootstrap is the main class used in Netty5 to configure, start and manage Netty.
2. Introduction to commonly used APIs
- Create a Server
ServerBootstrap serverBootstrap = new ServerBootstrap(); NioEventLoopGroup bossGroup = new NioEventLoopGroup(); NioEventLoopGroup workGroup = new NioEventLoopGroup(); serverBootstrap.group(bossGroup, workGroup) .channel(NioServerSocketChannel.class) .localAddress(new InetSocketAddress(port)) .childHandler(new ChannelInitializer<SocketChannel>() { @Override public void initChannel(SocketChannel socketChannel) { socketChannel.pipeline(). addLast(new CodecHandler(Encoding.getEncoding()),new TcpServerHandler()); } }); ChannelFuture f = serverBootstrap.bind().sync();
- Create a Client
Bootstrap bootstrap = new Bootstrap(); bootstrap.group(new NioEventLoopGroup()) .channel(NioSocketChannel.class) .remoteAddress(new InetSocketAddress(ip, port)) .handler(new ChannelInitializer<SocketChannel>() { @Override public void initChannel(SocketChannel ch) throws Exception { ch.pipeline().addLast(new CodecHandler(Encoding.getEncoding()),new TcpClientHandler()); } }); ChannelFuture f = bootstrap.connect().sync();
- Create a ChannelInboundHandlerAdapter
public class TcpServerHandler extends ChannelInboundHandlerAdapter { @Override public void channelRead(ChannelHandlerContext ctx, Object msg){ //处理读事件 } @Override public void channelReadComplete(ChannelHandlerContext ctx){ ctx.flush(); } @Override public void exceptionCaught(ChannelHandlerContext ctx,Throwable cause){ //处理异常事件 } }
- Create a ChannelOutboundHandlerAdapter
public class TcpClientHandler extends ChannelOutboundHandlerAdapter { @Override public void write(ChannelHandlerContext ctx, Object msg, ChannelPromise promise) { //处理写事件 } @Override public void exceptionCaught(ChannelHandlerContext ctx,Throwable cause){ //处理异常事件 } }
3. Practical application case
The following is a practical case To introduce how to use Netty5 for TCP communication.
Case description: Suppose there is an online examination system that needs to use TCP protocol to transmit examination answers to the server.
- Server side code:
public class ExamServer { public static void main(String[] args) throws InterruptedException { int port = 8080; if (args.length > 0){ port = Integer.parseInt(args[0]); } ServerBootstrap serverBootstrap = new ServerBootstrap(); NioEventLoopGroup bossGroup = new NioEventLoopGroup(); NioEventLoopGroup workGroup = new NioEventLoopGroup(); serverBootstrap.group(bossGroup, workGroup) .channel(NioServerSocketChannel.class) .localAddress(new InetSocketAddress(port)) .childHandler(new ChannelInitializer<SocketChannel>() { @Override public void initChannel(SocketChannel socketChannel) { socketChannel.pipeline() .addLast(new CodecHandler(Encoding.getEncoding()),new TcpServerHandler()); } }); ChannelFuture f = serverBootstrap.bind().sync(); //等待服务器监听端口关闭 f.channel().closeFuture().sync(); } }
- Client side code:
public class ExamClient { public static void main(String[] args) throws InterruptedException { String host = "localhost"; int port = 8080; Bootstrap bootstrap = new Bootstrap(); bootstrap.group(new NioEventLoopGroup()) .channel(NioSocketChannel.class) .remoteAddress(new InetSocketAddress(host, port)) .handler(new ChannelInitializer<SocketChannel>() { @Override public void initChannel(SocketChannel ch) throws Exception { ch.pipeline() .addLast(new CodecHandler(Encoding.getEncoding()),new TcpClientHandler()); } }); ChannelFuture f = bootstrap.connect().sync(); //一直等到channel关闭 f.channel().closeFuture().sync(); } }
- Test data reading and writing
public class TcpServerHandler extends ChannelInboundHandlerAdapter { @Override public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception { String request = (String)msg; //将请求解析为ExamAnswer对象 JSONObject obj = new JSONObject(request); String answer=obj.getString("answer"); //将答案保存到数据库中 saveAnswer(answer); //将响应返回给客户端 String response = "Success!"; ctx.write(response); ctx.flush(); } private void saveAnswer(String answer) { System.out.println("Save answer......"); // 这里可以自己根据实际需求进行具体操作 } @Override public void channelReadComplete(ChannelHandlerContext ctx){ ctx.flush(); } @Override public void exceptionCaught(ChannelHandlerContext ctx,Throwable cause){ cause.printStackTrace(); ctx.close(); } }
public class TcpClientHandler extends ChannelOutboundHandlerAdapter { @Override public void write(ChannelHandlerContext ctx, Object msg, ChannelPromise promise) throws Exception { //将请求数据转换成ExamAnswer对象 String request = "{ 'answer':'Java'}"; //发送请求数据到服务器 ctx.writeAndFlush(request); } @Override public void exceptionCaught(ChannelHandlerContext ctx,Throwable cause){ cause.printStackTrace(); ctx.close(); } }
4. Performance optimization of Netty5
In addition to Netty5’s powerful functions, its performance is also the biggest difference between it and other network communication frameworks. In practical applications, we usually need to consider how to further improve the performance of Netty5. Here are some commonly used Netty5 performance optimization methods.
- Thread pool optimization
Netty5's EventLoop is a thread pool for event processing, so the size of the thread pool directly affects the performance of Netty5. If the thread pool is too large, it will cause excessive waste of CPU resources, thus affecting performance; conversely, if the thread pool is too small, it may seriously affect concurrent processing efficiency. It is recommended to adjust the thread pool size appropriately according to the application scenario and server hardware configuration.
- Message packet processing
Since TCP communication is stream-oriented, that is to say, a data packet may be divided into multiple small packets for transmission. In order to ensure the integrity and accuracy of the data, we need to subcontract the messages. In Netty5, you can use LengthFieldBasedFrameDecoder for message packet processing.
- Cache Optimization
Netty5 supports custom caching strategies, which can be optimized according to application scenarios and business needs. For example, you can set the appropriate cache size and expiration time based on the size and frequency of cached content to avoid performance degradation caused by excessive cache size or expiration.
Conclusion
This article introduces how to use Netty5 for TCP communication, including the core components of Netty5, introduction to common APIs and practical application cases. At the same time, it also introduces how to use Netty5 to improve the performance and reliability of TCP communication. I hope that readers can better understand Netty5 through studying this article and use it flexibly in actual projects.
The above is the detailed content of Using Netty5 for TCP communication in Java API development. For more information, please follow other related articles on the PHP Chinese website!
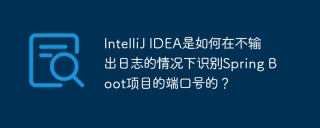
Start Spring using IntelliJIDEAUltimate version...
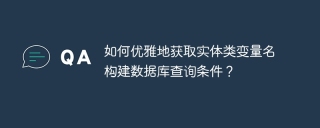
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
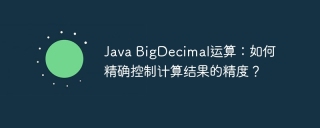
Java...
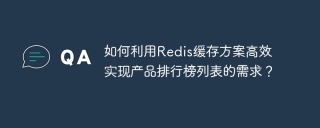
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
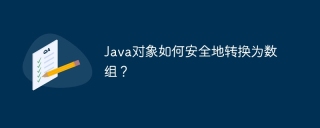
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
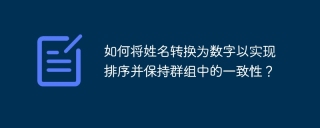
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
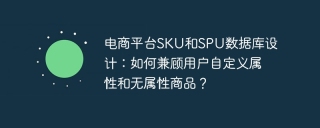
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
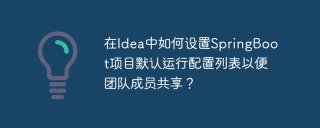
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
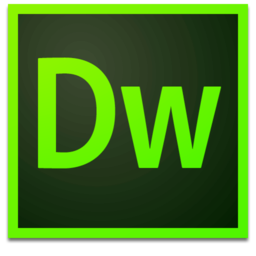
Dreamweaver Mac version
Visual web development tools
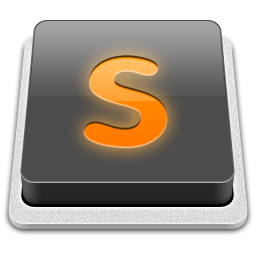
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
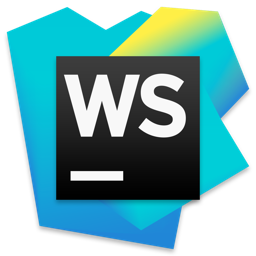
WebStorm Mac version
Useful JavaScript development tools