HTTP Routing in Go Language: A Complete Guide
In web development, routing is a crucial part. Routing maps URL requests to specific handler functions, allowing web applications to return different results based on the content of the request. In the Go language, the way to implement HTTP routing is very flexible and customizable. This article will introduce a complete guide to HTTP routing in the Go language, covering all the basics and best practices.
HTTP routing principle
HTTP routing is the process of mapping URL requests to handler functions in a web application. Simply put, routing is a mapping relationship. In the Go language, the http package provides a standard interface for implementing HTTP services and routing. Specifically, a route is a Handler-type function or method that wraps the actual HTTP request handler and configures it in one or more server-side routes.
Server-side routing is a mechanism for assigning HTTP requests to appropriate handlers. In the Go language, requests can be routed using the http package. The http package selects the best matching route based on the requested URL path when routing a request. Once the route is found, the route handler handles the request and generates the appropriate response.
Routing can be implemented in a variety of ways, including regular expressions and function mapping. Routing using regular expressions can match the input URL pattern with a predefined expression and perform the corresponding action. Function map routing can use methods and functions to respond to certain URL requests. These methods and functions are registered as route handlers and group requests by URL path.
HTTP routing implementation method
In Go language, routing can be implemented in two ways:
- Using http.ServeMux
http.ServeMux is the routing implementation provided by the http package. It is an HTTP request multiplexer used to match HTTP requests to their handlers. ServeMux provides a routing table, which stores a set of mapping relationships between patterns and Handlers:
mux := http.NewServeMux() mux.HandleFunc("/", homeHandler) mux.HandleFunc("/users", usersHandler)
ServeMux uses the HandleFunc function to render routes, which takes a handler and a URL string handler as parameters. The items added to the routing table by this method all start with / rather than the full URL path. Once the routing table matches a request to a specific handler, the handler responds to the appropriate request.
- Using third-party routing
In addition to http.ServeMux provided in the standard library, the Go language also has many third-party routing implementations. Some of the most popular third-party routers include:
- Gorilla Mux
- httprouter
- Chi
These packages provide APIs and http.ServeMux is similar, but usually has more powerful features and higher performance, such as support for routing groups, dynamic routing, and HTTP redirection.
How to write routing processing function?
The route processing function is a function of type http.HandlerFunc, and its function signature is the same as http.Request and http.ResponseWriter. This means that when the browser requests a URL that matches a route, Go will call the handler function and pass request-specific information (such as request headers and request body) as request parameters to the function. Typically, a handler interacts with a database, API, or other service and generates response data based on request parameters and returns it to the browser.
The following is an example of a simple route handler function:
func homeHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Welcome to the homepage!") } func usersHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Welcome to the users page!") }
These handler functions use the print function to write the text response into the HTTP response body. In a real application, you can use handlers to query a database, call an API service, return static files, or render other types of pages.
HTTP Routing Best Practices
There are a number of best practices that deserve special attention when writing HTTP routes. Here are some best practices:
- Using the http.Handler interface
http.Handler is an HTTP handler container interface that makes it easier for developers to operate on HTTP routing. It represents an object capable of reading HTTP requests and writing back HTTP responses. Therefore, when writing HTTP routes, it is best to use the http.Handler interface so that you can handle the route like all other HTTP requests. - Use regular expressions to optimize URLs
Using regular expressions can help you better optimize HTTP routing. And regular expressions can make code more concise without breaking security. - Using routing group structure
Routing group is a structure that can improve code readability and maintainability. The routing group structure allows you to organize different URL paths to make it easier to manage and maintain routing tables. - Using middleware
Middleware is a popular HTTP routing optimization technology. Middleware can handle requests and responses before or after the request reaches the route handler to provide better service and better performance to users.
Final summary
HTTP routing is one of the most important components of web applications. Good HTTP routing design can allow us to process HTTP requests faster. In the Go language, there are multiple ways to implement HTTP routing, including http.ServeMux in the standard library and third-party routing packages such as Gorilla Mux and httprouter. Of course, we need to follow best practices for writing HTTP routes in order to write more performant, more maintainable, and more secure code. I hope this article can help you better understand HTTP routing in Go language.
The above is the detailed content of HTTP Routing in Go Language: A Complete Guide. For more information, please follow other related articles on the PHP Chinese website!
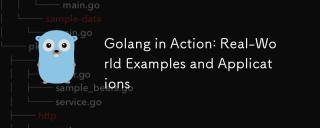
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
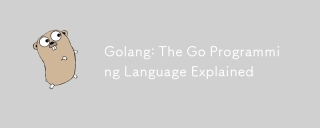
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
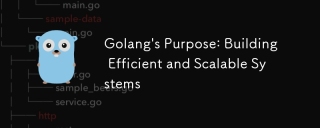
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
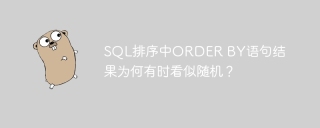
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
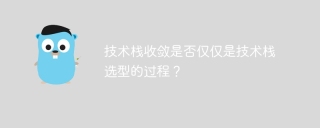
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
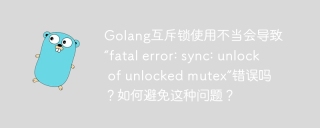
Golang ...
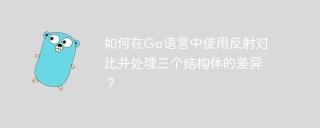
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
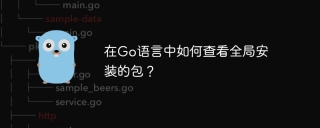
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
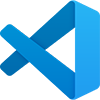
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
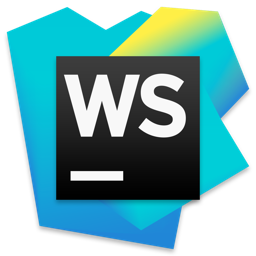
WebStorm Mac version
Useful JavaScript development tools
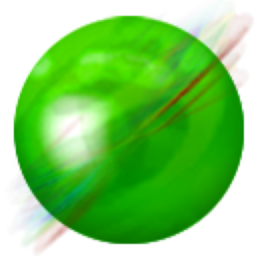
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment