How to use Go language to audit data permissions of MySQL database
With the continuous development of the Internet, the use of databases is becoming more and more widespread. In enterprises, the management of data permissions has also become an issue that cannot be ignored. Therefore, how to review and manage data permissions has become a problem that enterprises must face. This article will introduce how to use Go language to audit data permissions of MySQL database.
1. Introduction to MySQL database permissions
In the MySQL database, user permissions can be divided into four types: Global (global), Database (database), Table (data table) and Column ( column), respectively representing access rights to the entire MySQL server, a certain database, a certain data table and a certain column.
Global permissions are the highest permissions, granting users permission to operate on the entire MySQL server; Database permissions indicate that users can operate on a certain database; Table permissions indicate that users can operate on a certain data table Permission to operate on a certain column; Column permission only allows operations on a certain column.
2. Connection between Go language and MySQL database
Using Go language to operate MySQL database requires the use of third-party libraries provided by Go language, such as go-sql-driver/mysql. The installation method is as follows:
go get -u github.com/go-sql-driver/mysql
Then, you need to use the following code to connect to the MySQL database in Go language:
import( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main(){ db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/mydb") if err != nil { log.Fatal(err.Error()) } defer db.Close() }
Among them, the first parameter "mysql" means using the MySQL database, and the second parameter In "root:password@tcp(127.0.0.1:3306)/mydb", root represents the user name, password represents the password, 127.0.0.1 represents the IP address of the database, 3306 represents the port number of the MySQL database, and mydb represents the name of the database to be connected. . Next, use the defer statement to close the database connection.
3. Go language to implement MySQL database permission audit
- Query user permissions
Use the following SQL statement to query the permissions owned by the user:
SELECT * FROM mysql.user WHERE User = 'username' AND Host = 'host';
Among them, username represents the user name to be queried, and host represents the host address.
The code for querying user permissions in Go language is as follows:
func checkUserPermission(db *sql.DB, username string, host string) bool { query := fmt.Sprintf("SELECT * FROM mysql.user WHERE User = '%s' AND Host = '%s'", username, host) rows, err := db.Query(query) if err != nil { log.Fatal(err.Error) } defer rows.Close() var user string for rows.Next() { err := rows.Scan(&user) if err != nil { log.Fatal(err.Error) } return true } return false }
Among them, db represents the MySQL database to be connected, username represents the user name to be queried, and host represents the host address.
First, use the fmt.Sprintf() method to construct the SQL statement. Then, use the db.Query() method to query the database and use the rows.Close() method to close the result set.
Next, in the loop, use the rows.Scan() method to scan each row of the result set. If the user's record is found, true is returned; otherwise, false is returned.
- Query database permissions
Use the following SQL statement to query the database permissions owned by the user:
SHOW GRANTS FOR 'username'@'host';
Query database permissions in Go language The code is as follows:
func checkDatabasePermission(db *sql.DB, username string, host string, database string) bool { query := fmt.Sprintf("SHOW GRANTS FOR '%s'@'%s'", username, host) rows, err := db.Query(query) if err != nil { log.Fatal(err.Error) } defer rows.Close() for rows.Next() { var grants string err := rows.Scan(&grants) if err != nil { log.Fatal(err.Error) } if strings.Contains(grants, fmt.Sprintf("`%s`.*", database)) { return true } } return false }
Among them, db represents the MySQL database to be connected, username represents the user name to be queried, host represents the host address, and database represents the name of the database to be queried.
First, use the fmt.Sprintf() method to construct the SQL statement. Then, use the db.Query() method to query the database and use the rows.Close() method to close the result set.
Next, in the loop, use the rows.Scan() method to scan each row of the result set. If the found result contains the name of the database to be queried, true is returned; otherwise, false is returned.
- Query data table permissions
Use the following SQL statement to query the data table permissions owned by the user:
SHOW GRANTS FOR 'username'@'host' ON `database`.`table`;
Query data in Go language The code for table permissions is as follows:
func checkTablePermission(db *sql.DB, username string, host string, database string, table string) bool { query := fmt.Sprintf("SHOW GRANTS FOR '%s'@'%s' ON `%s`.`%s`", username, host, database, table) rows, err := db.Query(query) if err != nil { log.Fatal(err.Error) } defer rows.Close() for rows.Next() { var grants string err := rows.Scan(&grants) if err != nil { log.Fatal(err.Error) } if strings.Contains(grants, "ALL PRIVILEGES") || strings.Contains(grants, "SELECT") { return true } } return false }
Among them, db represents the MySQL database to be connected, username represents the user name to be queried, host represents the host address, database represents the name of the database to be queried, and table represents the data to be queried. Table name.
First, use the fmt.Sprintf() method to construct the SQL statement. Then, use the db.Query() method to query the database and use the rows.Close() method to close the result set.
Next, in the loop, use the rows.Scan() method to scan each row of the result set. If the found result contains ALL PRIVILEGES or SELECT, return true; otherwise, return false.
4. Summary
This article introduces how to use Go language to conduct data permission audit of MySQL database. By writing relevant SQL query statements and using the third-party library provided by the Go language to connect to the MySQL database, the review and management of user permissions, database permissions, and data table permissions are realized. Using Go language to review data permissions on MySQL databases is convenient and efficient, and can help enterprises better manage and review database permissions.
The above is the detailed content of How to use Go language to audit data permissions of MySQL database. For more information, please follow other related articles on the PHP Chinese website!
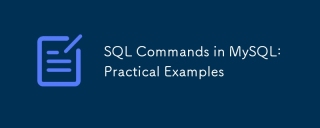
SQL commands in MySQL can be divided into categories such as DDL, DML, DQL, DCL, etc., and are used to create, modify, delete databases and tables, insert, update, delete data, and perform complex query operations. 1. Basic usage includes CREATETABLE creation table, INSERTINTO insert data, and SELECT query data. 2. Advanced usage involves JOIN for table joins, subqueries and GROUPBY for data aggregation. 3. Common errors such as syntax errors, data type mismatch and permission problems can be debugged through syntax checking, data type conversion and permission management. 4. Performance optimization suggestions include using indexes, avoiding full table scanning, optimizing JOIN operations and using transactions to ensure data consistency.
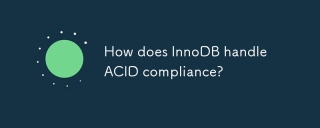
InnoDB achieves atomicity through undolog, consistency and isolation through locking mechanism and MVCC, and persistence through redolog. 1) Atomicity: Use undolog to record the original data to ensure that the transaction can be rolled back. 2) Consistency: Ensure the data consistency through row-level locking and MVCC. 3) Isolation: Supports multiple isolation levels, and REPEATABLEREAD is used by default. 4) Persistence: Use redolog to record modifications to ensure that data is saved for a long time.

MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
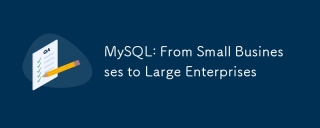
MySQL is suitable for small and large enterprises. 1) Small businesses can use MySQL for basic data management, such as storing customer information. 2) Large enterprises can use MySQL to process massive data and complex business logic to optimize query performance and transaction processing.
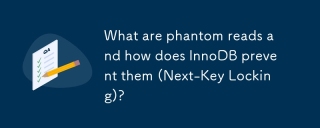
InnoDB effectively prevents phantom reading through Next-KeyLocking mechanism. 1) Next-KeyLocking combines row lock and gap lock to lock records and their gaps to prevent new records from being inserted. 2) In practical applications, by optimizing query and adjusting isolation levels, lock competition can be reduced and concurrency performance can be improved.
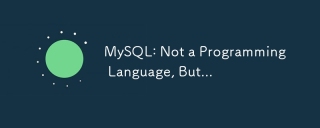
MySQL is not a programming language, but its query language SQL has the characteristics of a programming language: 1. SQL supports conditional judgment, loops and variable operations; 2. Through stored procedures, triggers and functions, users can perform complex logical operations in the database.
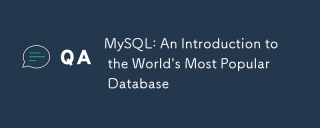
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
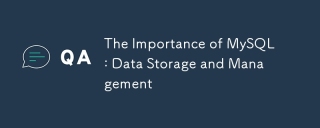
MySQL is an open source relational database management system suitable for data storage, management, query and security. 1. It supports a variety of operating systems and is widely used in Web applications and other fields. 2. Through the client-server architecture and different storage engines, MySQL processes data efficiently. 3. Basic usage includes creating databases and tables, inserting, querying and updating data. 4. Advanced usage involves complex queries and stored procedures. 5. Common errors can be debugged through the EXPLAIN statement. 6. Performance optimization includes the rational use of indexes and optimized query statements.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
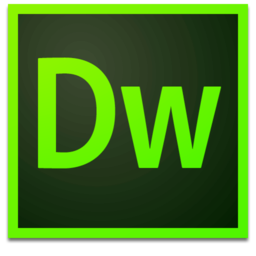
Dreamweaver Mac version
Visual web development tools
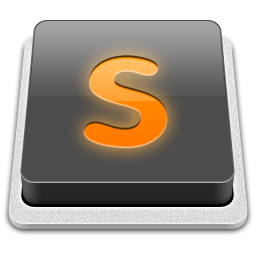
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
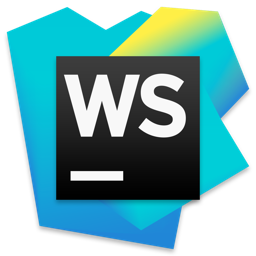
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.