Using javaparser for code analysis in Java API development
In the Java development process, it is often necessary to analyze and process the code. In this process, you can use javaparser, a popular open source library for Java code parsing. This article will introduce the basics of Java code parsing using javaparser, and how to use it for code analysis and processing.
What is javaparser?
Javaparser is an open source Java code parser. It provides a convenient way to read and manipulate the AST (Abstract Syntax Tree) of Java code. Using AST, different aspects of Java code such as syntax, structure, data types, etc. can be analyzed and processed.
Javaparser provides an easy-to-use API to quickly analyze and process Java code in a few lines of code. It parses Java code into an AST, which developers can then use an API to traverse, modify, and reuse.
Steps to use javaparser for code analysis
Using javaparser for code analysis requires the following steps:
1. Include the javaparser library
First, you need to add javaparser The library is included in the development project. The jar files can be downloaded locally and added to the classpath of the development project. If you use build tools such as Maven or Gradle, you can also specify the version information of the libraries you need to use in the project configuration.
2. Create AST
Use the following code to create an AST:
FileInputStream in = new FileInputStream("filename.java"); CompilationUnit cu = JavaParser.parse(in);
Here, you need to pass the Java source file name to the FileInputStream constructor. The file is then parsed using the parse method of the JavaParser class. It returns a CompilationUnit object representing the AST of the Java source file.
3. Traverse the AST
With the CompilationUnit object, you can traverse the AST and analyze and process it. The following is sample code for iterating over a CompilationUnit object:
public static void visitNode(Node node) { if(node == null) return; System.out.println(node.getClass().getSimpleName()); node.getChildrenNodes().forEach(child -> visitNode(child)); } visitNode(cu);
Here, the visitNode method recursively traverses the AST and prints the class name of each node. When traversing the AST, you can also use other API methods to obtain the properties and status of nodes for more advanced analysis and processing.
4. Modify the AST
The AST can be modified using the following method:
MethodDeclaration method = new MethodDeclaration(); method.setName("newMethod"); method.setPublic(true); cu.addClassOrInterface(newClass);
Here, a new MethodDeclaration object is created and set. Then, add new methods to the CompilationUnit object.
5. Save the modified file
Finally, save the modified Java file to disk:
FileOutputStream out = new FileOutputStream("filename.java"); out.write(cu.toString().getBytes()); out.close();
Here, use the FileOutputStream class to create an output stream to Save the modified code. Converts a CompilationUnit object to a string and writes it to the output stream.
Summary
Javaparser is a convenient Java code parser that can be used to analyze and process Java code. Use it to easily create an AST, traverse and modify it, and save the modified file to disk. The library provides an easy-to-use API and is also a popular open source library, so more features and support are available through community support.
The above is the detailed content of Using javaparser for code analysis in Java API development. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
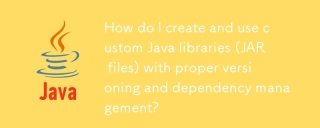
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
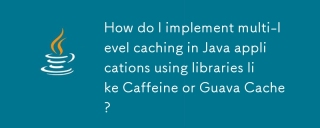
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
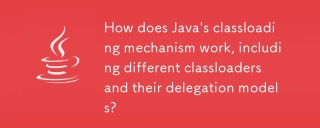
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
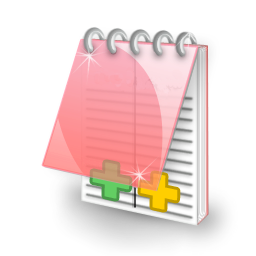
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
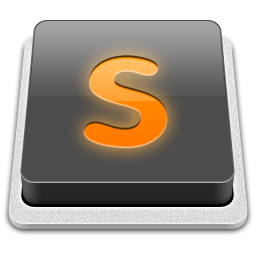
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.