Unit Testing and Integration Testing in Go: Best Practices
In software development, testing is an extremely important link. Testing not only helps developers find errors in the code, but also improves the quality and maintainability of the code. In Go language, testing is done using Go Test tool. Go Test supports two testing methods: unit testing and integration testing. In this article, we will introduce the best practices for unit testing and integration testing in Go language.
- Unit testing
Unit testing refers to testing the smallest testable unit in the program. In Go language, a function or method is a minimum testable unit. The following are some best practices when writing unit tests:
1.1 Use a test framework
The test framework can help us write test code more conveniently and judge the output of the program through various assertion functions Is it consistent with expectations? Commonly used testing frameworks include testing and Testify. Testing is the testing framework that comes with the Go language, while Testify is a third-party framework that provides more assertion functions and tool functions to make the test code more concise and readable.
1.2 Use table-driven testing
Table-driven testing refers to storing test data and expected results in a table or array, and using a loop to traverse the table or array for testing. This approach can reduce code duplication and redundancy, making it easier to maintain and modify. The following is an example of using table-driven testing:
func TestAdd(t *testing.T) { tests := []struct { a, b, expected int }{ {1, 2, 3}, {0, 0, 0}, {-1, 1, 0}, {1, -1, 0}, } for _, tt := range tests { actual := Add(tt.a, tt.b) if actual != tt.expected { t.Errorf("Add(%d, %d): expected %d, actual %d", tt.a, tt.b, tt.expected, actual) } } }
1.3 Use test coverage to detect code coverage
Test coverage can help us evaluate the quality of the test code. It detects how much program code is covered by the test code and displays the results as a percentage. When using Go Test, just add the -cover parameter to generate a coverage report:
go test -cover ./...
- Integration testing
Integration testing refers to testing multiple modules. to ensure normal interaction between them. In the Go language, you can use the httptest package to simulate HTTP requests and responses for integration testing. Here are some best practices when writing integration tests:
2.1 Use a real database
In integration tests, you should use a real database for testing. This can more realistically simulate the actual situation in the production environment and avoid artifacts during testing. Of course, during the testing process, you should ensure that the database used is independent and will not affect the data in the production environment.
2.2 Use the test helper function
In order to avoid duplication of code and improve the maintainability of the code, you can write a test helper function to handle some repetitive work in the test code. For example, you can write a function that initializes the database so that it is cleared and some basic data is inserted when the test starts.
2.3 Writing end-to-end tests
End-to-end testing refers to testing the entire application to ensure that its various components (such as user interface, database, and network connections) work together properly . This kind of testing can help us find problems hidden between various components and ensure that the application can run normally in the production environment.
Summary
In the Go language, unit testing and integration testing are important means to ensure code quality and maintainability. When writing test code, you should follow best practices and use techniques such as testing frameworks, table-driven testing, and test coverage to test your code more efficiently and reliably. When using integration testing, you should use a real database, write test helper functions, and write end-to-end tests to maintain the correctness and stability of the code.
The above is the detailed content of Unit Testing and Integration Testing in Go: Best Practices. For more information, please follow other related articles on the PHP Chinese website!
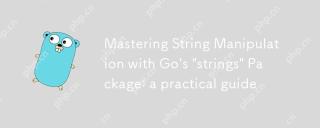
ThestringspackageinGoiscrucialforefficientstringmanipulationduetoitsoptimizedfunctionsandUnicodesupport.1)ItsimplifiesoperationswithfunctionslikeContains,Join,Split,andReplaceAll.2)IthandlesUTF-8encoding,ensuringcorrectmanipulationofUnicodecharacters
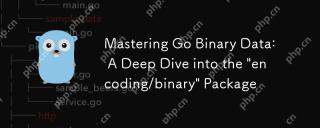
The"encoding/binary"packageinGoiscrucialforefficientbinarydatamanipulation,offeringperformancebenefitsinnetworkprogramming,fileI/O,andsystemoperations.Itsupportsendiannessflexibility,handlesvariousdatatypes,andisessentialforcustomprotocolsa
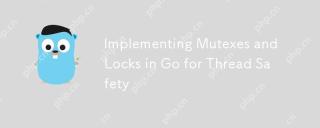
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
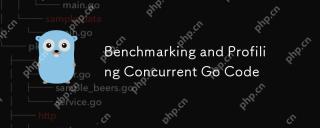
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
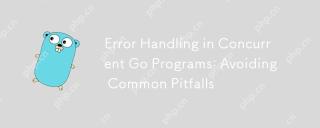
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
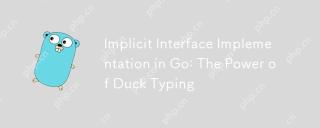
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
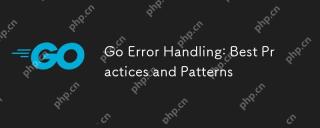
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
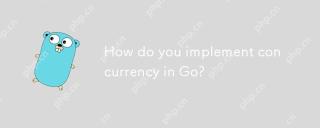
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
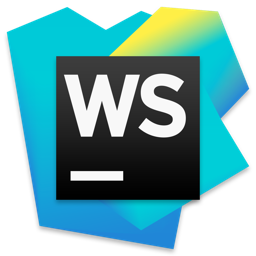
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
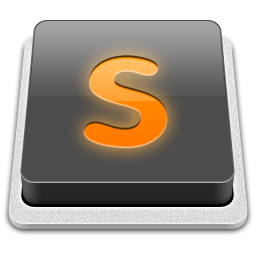
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
