With the popularization of large-scale applications and the rapid development of Internet technology, databases have become an essential tool for data storage, management, and retrieval. MySQL
is an open source and free relational database management system. At the same time, the Go
language is favored by more and more developers due to its high concurrency performance and other advantages. In application scenarios, data roles and data attributes play an important role. In order to better manage data, the attributes of different data roles need to be divided. This article will focus on how to divide data roles and attributes with the MySQL
database in the Go
language.
1. Division of roles
In the database, data roles are usually divided according to business needs and how the data is used. For example, in an e-commerce platform, we can divide data roles into users, products, orders, etc. Different roles have different data attributes. In order to play the role of the MySQL
database, we need to create corresponding tables to store data information for different roles.
1.1 Create a table
First, use MySQL
to create the SQL statement of the user table as follows:
CREATE TABLE user( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(64) NOT NULL, age INT NOT NULL, gender VARCHAR(4) );
Among them, user
table It contains four attributes: id, name, age and gender. The id attribute is the primary key and is automatically added. Similarly, we can also set the properties of other tables according to business needs.
1.2 Data addition, deletion, modification and query
In the Go
language, using MySQL
to add, delete, modify and query data requires the use of SQL Statement, mainly involves the following two methods:
1. CREATE/INSERT/UPDATE/DELETE
##CREATE command is used to create a table. The
INSERT command is used to add data to the table, the
UPDATE command is used to update the table, and the
DELETE command is used to delete some data in the table.
import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { username := "root" password := "password" host := "localhost:3306" database := "db1" db, err := sql.Open("mysql", fmt.Sprintf("%s:%s@tcp(%s)/%s", username, password, host, database)) if err != nil { panic(err) } defer db.Close() // CREATE 命令 _, err = db.Exec("CREATE TABLE user(id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(64) NOT NULL, age INT NOT NULL, gender VARCHAR(4))") if err != nil { panic(err) } // INSERT 命令 _, err = db.Exec("INSERT INTO user(name, age, gender) VALUES (?, ?, ?)", "Tom", 18, "男") if err != nil { panic(err) } // UPDATE 命令 _, err = db.Exec("UPDATE user SET gender = ? WHERE id = ?", "女", 1) if err != nil { panic(err) } // DELETE 命令 _, err = db.Exec("DELETE FROM user WHERE id = ?", 1) if err != nil { panic(err) } }
2. SELECT
SELECT command is used to query the data in the table. You can use
Query or
QueryRow method to execute SQL queries.
import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { username := "root" password := "password" host := "localhost:3306" database := "db1" db, err := sql.Open("mysql", fmt.Sprintf("%s:%s@tcp(%s)/%s", username, password, host, database)) if err != nil { panic(err) } defer db.Close() // 查询 user 表格中所有信息 rows, err := db.Query("SELECT * FROM user") if err != nil { panic(err) } defer rows.Close() for rows.Next() { var id, age int var name, gender string err := rows.Scan(&id, &name, &age, &gender) if err != nil { panic(err) } fmt.Println(id, name, age, gender) } // 根据条件查询 user 表格中数据 row := db.QueryRow("SELECT * FROM user WHERE id = ?", 2) var id, age int var name, gender string err = row.Scan(&id, &name, &age, &gender) if err != nil { if err == sql.ErrNoRows { fmt.Println("没有查询到记录") return } panic(err) } fmt.Println(id, name, age, gender) }Through the above code examples, we can perform common operations such as querying, adding, deleting and updating data. 2. Division of attributesFor data of different roles, the importance of each data attribute is also different. Differences in attribute types and ranges of attribute values also have an impact on how the data is processed. 2.1 Selection of data typeIn
MySQL, the data types mainly include
INT,
VARCHAR,
DATE,
FLOAT,
DOUBLE, etc. Different data types occupy different storage spaces. When selecting attribute types, you need to reduce the storage space as much as possible, otherwise it will have a negative impact on database performance.
INT type for storage, because the age value will not exceed 100, and the
INT type range is -2147483648 to 2147483647, the storage space is smaller and the query is faster.
VARCHAR(64) type, but the password length cannot be too long, otherwise it will increase storage overhead. At the same time, user passwords need to be protected, and generally need to be encrypted using a hash algorithm, such as MD5, SHA-1, SHA-256, etc.
import ( "crypto/md5" "database/sql" "encoding/hex" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { password := "123456" salt := "random_generated_salt" hash := md5.Sum([]byte(password + salt)) passwordHash := hex.EncodeToString(hash[:]) fmt.Println(passwordHash) // 825b5f05eb9584b068ce830d0983e7c9 // 使用哈希算法加密密码,将其值存储到数据库中 username := "root" password := "password" host := "localhost:3306" database := "db1" db, err := sql.Open("mysql", fmt.Sprintf("%s:%s@tcp(%s)/%s", username, password, host, database)) if err != nil { panic(err) } defer db.Close() _, err = db.Exec("INSERT INTO user(name, age, gender, password) VALUES (?, ?, ?, ?)", "Tom", 18, "男", passwordHash) if err != nil { panic(err) } }By using the MD5 encryption algorithm, the length of the password value will become a relatively fixed value, thereby achieving privacy protection. 3. SummaryIn this article, we introduced how to divide data roles and attributes in the
Go language with the
MySQL database. In order to better manage data, we need to divide different data roles according to different business needs, and control the range of data attribute types and values. At the same time, when performing data addition, deletion, modification and query operations, corresponding SQL statements need to be used. Through the above introduction, I believe readers have a deeper understanding of how to divide data roles and attributes.
The above is the detailed content of Go language and MySQL database: How to divide data role attributes?. For more information, please follow other related articles on the PHP Chinese website!
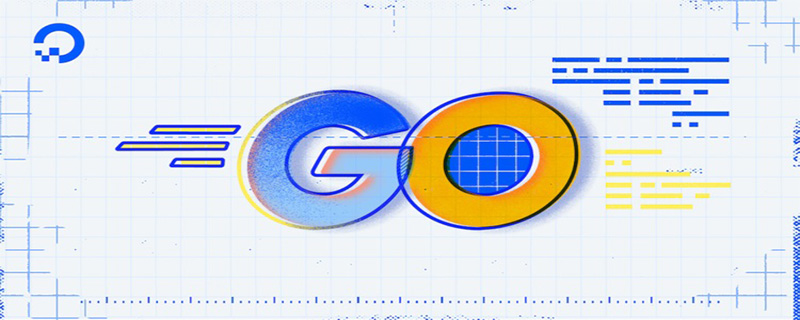
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
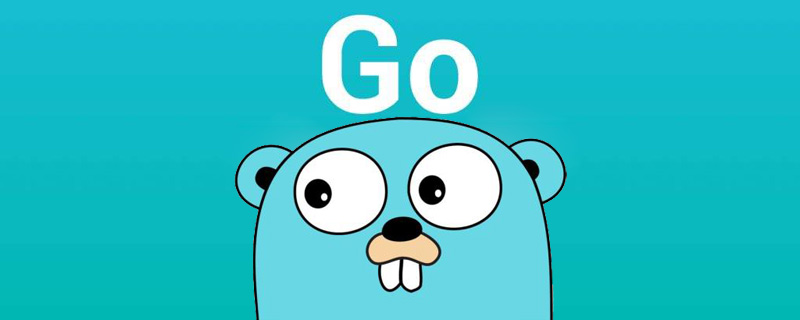
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
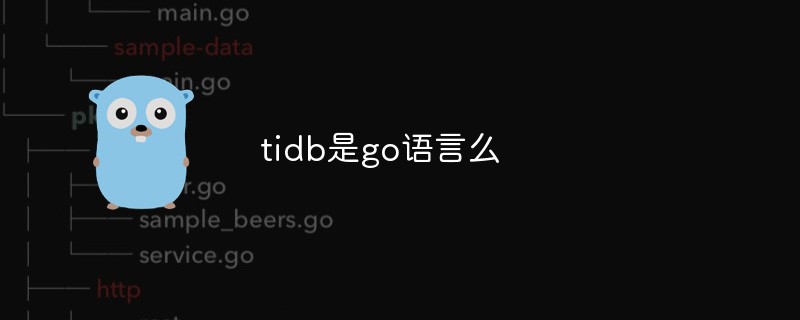
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
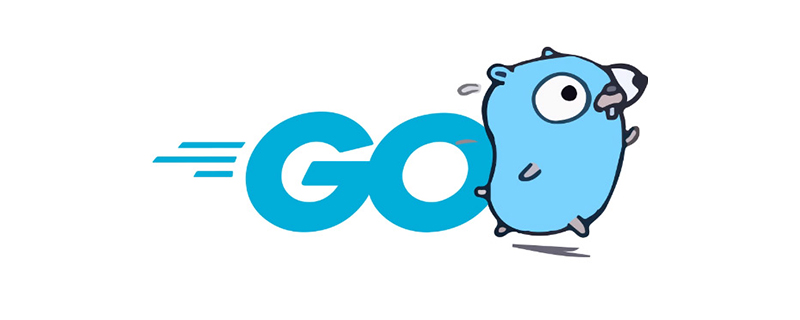
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
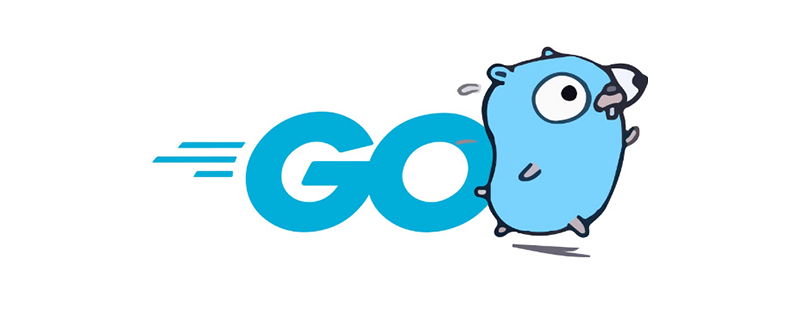
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
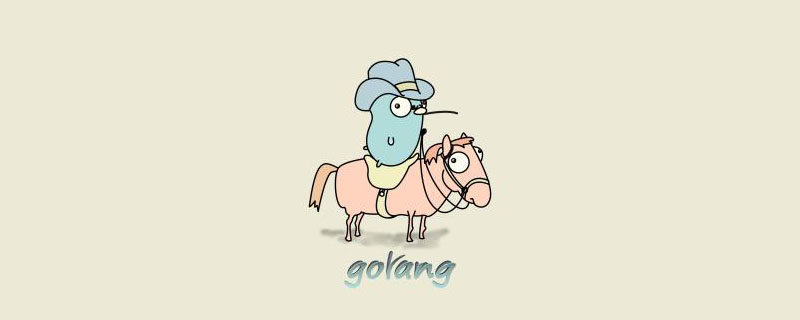
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
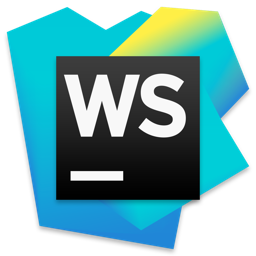
WebStorm Mac version
Useful JavaScript development tools
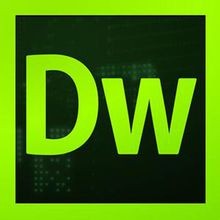
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
