How to deal with GraphQL API in PHP API development
With the continuous development of web applications, the use of API (Application Programming Interface) is becoming more and more common. API, as the core of web applications, allows different applications to communicate with each other and share data. In PHP development, GraphQL API has become an increasingly popular development method, which can be more flexible and efficient than traditional RESTful API.
GraphQL is an API query language developed by Facebook, which allows the client to obtain the precise data required from the server. Compared with traditional RESTful APIs, GraphQL is more flexible and efficient, and can greatly reduce the communication volume between the application and the server. In this article, we will explore how to develop and process GraphQL APIs in PHP.
The first step is to understand how the GraphQL API works. The core of the GraphQL API is a Schema (schema), which defines queryable and changeable data types and operations. The client can use the GraphQL query language to submit a request to the server to query the required data. The server interprets the operation according to GraphQL Schema and returns the data required by the client. graphql-php is a PHP library that can help us develop GraphQL API more conveniently.
The first step in developing a GraphQL API is to create a Schema. We need to define Schema, which contains queryable and changeable data types and operations. Schema usually contains query objects, change objects, input objects and scalar types.
Among them, the query object represents the data that the client can query; the change object represents the data that the client can modify; the input object represents the data that the client can submit to the server; scalar types include strings, Boolean values, Basic data types such as integers and floating point numbers.
In the process of creating Schema, we need to specify the type and parameters of each object and field. For example, the following is a simple query object:
type Query { hello: String! }
This query object indicates that a string type hello field can be queried. The exclamation point indicates that this field is required and cannot return null.
Next, we need to define a Resolver (parser), which is responsible for processing data acquisition and changes for each object or field. Resolver is a PHP callback function that needs to receive the request and context information, and then return the query results. For example, for the hello field defined above, we can create a Resolver:
$resolvers = [ 'Query' => [ 'hello' => function () { return 'Hello, World!'; }, ], ];
This Resolver directly returns the string "Hello, World!". We need to bind this Resolver to Schema so that query requests can be processed.
Next, we can use the GraphQL class in the graphql-php library to write an HTTP request processor to handle the client's query request. The HTTP request handler receives the request, parses the query and changes in the request into the GraphQL query language, and passes it to the Schema.
<?php require_once __DIR__ . '/vendor/autoload.php'; use GraphQLGraphQL; use GraphQLTypeSchema; use function GraphQLTypealidate; $schema = new Schema([ 'query' => $queryType, 'mutation' => $mutationType, ]); try { $rawInput = file_get_contents('php://input'); $input = json_decode($rawInput, true); $query = isset($input['query']) ? $input['query'] : null; $variableValues = isset($input['variables']) ? $input['variables'] : null; $operationName = isset($input['operationName']) ? $input['operationName'] : null; $result = GraphQL::executeQuery($schema, $query, null, null, $variableValues, $operationName); $output = $result->toArray(); } catch (Exception $e) { $output = [ 'error' => [ 'message' => $e->getMessage() ] ]; } header('Content-Type: application/json; charset=UTF-8'); echo json_encode($output);
Finally, we also need to consider how to protect the GraphQL API. The GraphQL API allows clients to query and modify data flexibly, so some measures are needed to prevent abuse. We can ensure the security and legality of the API through authentication and authorization.
In summary, developing GraphQL API requires us to define Schema, write Resolver, and create HTTP request processor. GraphQL API has the advantages of high flexibility and high efficiency. Therefore, if you need to build a flexible and efficient API in PHP development, then GraphQL API will undoubtedly be a good choice.
The above is the detailed content of How to deal with GraphQL API in PHP API development. For more information, please follow other related articles on the PHP Chinese website!
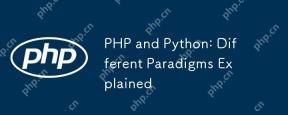
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
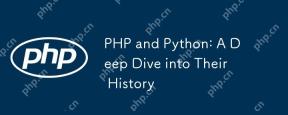
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
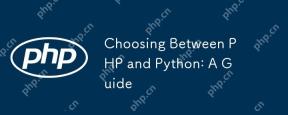
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
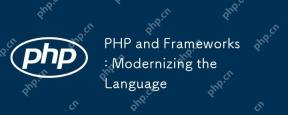
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
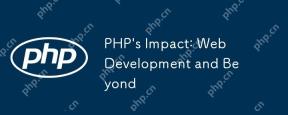
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
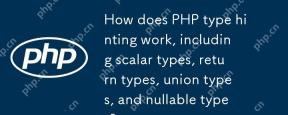
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
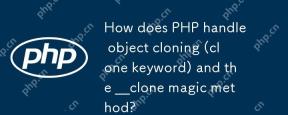
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
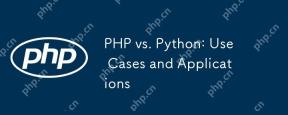
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
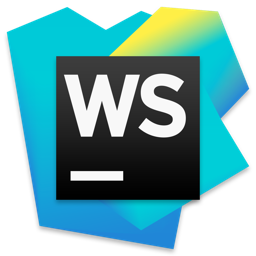
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor