


How to create high-performance full-text search of MySQL data using Go language
With the rapid development of the Internet, the amount of data is increasing, and the need for fast data query is becoming more and more urgent. At present, full-text search engine is a relatively common data query method, which can be applied to various fields, such as e-commerce websites, news websites, blogs, etc. This article will introduce how to use Go language to create high-performance MySQL data full-text search.
1. What is full-text search
Full-text search (Full-Text Search) refers to the search technology that finds all text records that match specific keywords from a text. Different from traditional fuzzy search and string matching, the full-text search engine is a query method that analyzes multiple factors such as vocabulary, grammar, and semantics, which can greatly improve the efficiency and accuracy of data query.
2. Go language and MySQL
Go language is an open source programming language developed by Google and officially released in 2009. It has the characteristics of simplicity, efficiency, and concurrency, and is widely used in network programming, cloud computing, microservices and other fields. MySQL is an open source relational database with advantages such as high performance, scalability, and data security. It is one of the most common database management systems in web application development. The deep integration of Go language and MySQL can provide us with powerful data query and processing capabilities.
3. Create a MySQL full-text index
Before using MySQL for full-text search, you first need to create a full-text index in the table to be searched. Assume that the name of our data table is "article", which contains two fields: "title" and "content". We need to create a full-text index for it. The specific steps are as follows:
- Create database connection
db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/database") if err != nil { log.Fatal(err) } defer db.Close()
- Execute the full-text index creation statement
_, err := db.Exec("ALTER TABLE article ADD FULLTEXT(title, content)") if err != nil { log.Fatal(err) }
After execution, you can create a full-text index on the two fields "title" and "content" so that Conduct efficient text searches.
4. Go language to implement MySQL full-text search
After creating the full-text index, we can use Go language to implement MySQL full-text search. The following is the specific implementation code.
- Define query result structure
type Result struct { ID int64 `json:"id"` Title string `json:"title"` Content string `json:"content"` }
We have defined three attributes, representing article ID, title and content respectively.
- Implementing the full-text search function
func Search(keyword string) ([]Result, error) { db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/database") if err != nil { return nil, err } defer db.Close() rows, err := db.Query("SELECT id, title, content, MATCH(title, content) AGAINST(?) AS score FROM article WHERE MATCH(title, content) AGAINST(?)", keyword, keyword) if err != nil { return nil, err } defer rows.Close() var results []Result for rows.Next() { var id int64 var title string var content string var score float64 if err := rows.Scan(&id, &title, &content, &score); err != nil { return nil, err } results = append(results, Result{ID: id, Title: title, Content: content}) } if err := rows.Err(); err != nil { return nil, err } return results, nil }
This function receives a keyword as a parameter, queries the "title" and "content" fields in the "article" table, and Use the MATCH AGAINST statement to calculate the score, and finally parse the query results into the defined Result structure.
5. Optimize query performance
In practical applications, the query characteristics of full-text search are complex query statements and the search for large amounts of data, so it needs to be optimized.
- Use connection pool
When querying multiple times, the creation and destruction of database connections will bring additional overhead. Using a connection pool can reduce this overhead and improve query efficiency. The connection pool size can be set using DB.SetMaxIdleConns and DB.SetMaxOpenConns in the "database/sql" package.
- Build cache
Full-text search queries have high performance requirements, and cache can be used to optimize query speed. When querying, you can first obtain the result from the cache. If it does not exist, perform a database query and cache the query results. In subsequent queries, if there are results in the cache, they will be returned directly to avoid repeated queries.
- Use paging query
When processing large amounts of data, using paging query can reduce the resources required for query and improve query efficiency. You can use the "LIMIT" and "OFFSET" keywords to paginate the query results, and set the number of items displayed on each page and the current page number.
6. Summary
Full-text search is an efficient data query method that can help us quickly search and obtain the data we need. Using the combination of Go language and MySQL, high-performance full-text search function can be achieved. In practical applications, it can also be optimized by combining connection pooling, caching, paging query and other technologies to further improve query efficiency and performance.
The above is the detailed content of How to create high-performance full-text search of MySQL data using Go language. For more information, please follow other related articles on the PHP Chinese website!
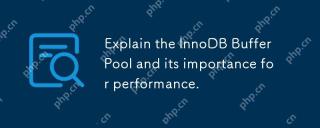
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
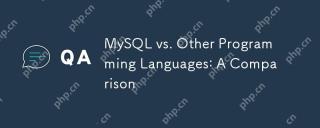
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
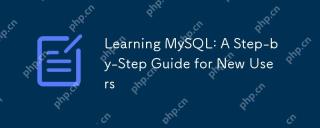
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
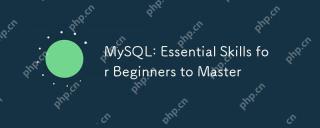
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
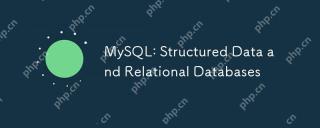
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
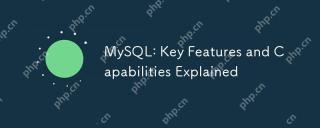
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
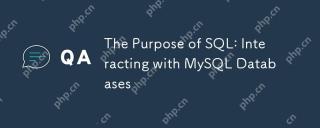
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
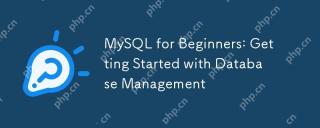
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
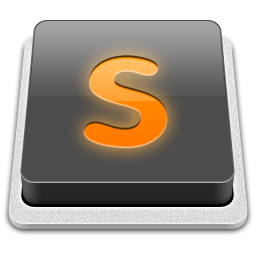
SublimeText3 Mac version
God-level code editing software (SublimeText3)
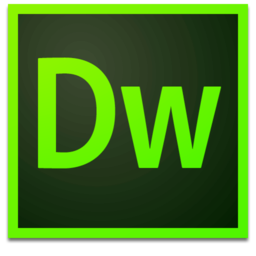
Dreamweaver Mac version
Visual web development tools
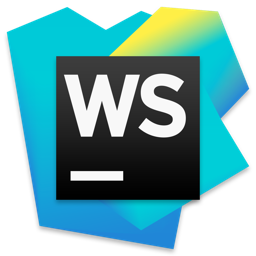
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment