


Java back-end development: Using Java Network Interface for API network interface management
Java back-end development has become one of the most popular programming languages in the Internet environment. In Java back-end development, the management of API network interfaces is an important link because it is related to data transmission and interaction between multiple systems. This article will introduce the use of Java Network Interface (JNI for short) and manage API network interfaces in Java back-end development.
1. Overview of Java Network Interface
Java Network Interface is a standard API of Java, used to manage network interfaces, including obtaining network interface information and changing the configuration of network interfaces. In layman's terms, JNI is a network manager in Java, which can be used to control and operate network interfaces.
Using JNI in Java can complete many tasks, such as: obtaining the local IP address, MAC address and other network information; obtaining the basic information of the network interface (interface name, address, status, etc.); implementing the network through JNI Operations such as sending and receiving data packets.
2. Use JNI to manage API network interfaces
In Java back-end development, we often need to share data through APIs, and the network interface is the core of implementing the API network interface. Here we will introduce how to manage network interfaces through JNI.
- Get the basic information of the network interface
Get the basic information of the network interface, including interface name, IP address, subnet mask, MAC address and other information. The basis of management. The following is an example of obtaining basic information about a network interface:
import java.net.InetAddress; import java.net.NetworkInterface; import java.net.SocketException; import java.util.Enumeration; public class NetworkInterfaceDemo { public static void main(String[] args) throws SocketException { Enumeration<NetworkInterface> interfaces = NetworkInterface.getNetworkInterfaces(); while (interfaces.hasMoreElements()) { NetworkInterface ni = interfaces.nextElement(); System.out.printf("Name: %s ", ni.getName()); System.out.printf("Display name: %s ", ni.getDisplayName()); Enumeration<InetAddress> inetAddresses = ni.getInetAddresses(); while (inetAddresses.hasMoreElements()) { InetAddress inetAddress = inetAddresses.nextElement(); System.out.printf("InetAddress: %s ", inetAddress); } byte[] hardwareAddress = ni.getHardwareAddress(); if (hardwareAddress != null) { System.out.print("MAC address: "); for (byte b : hardwareAddress) { System.out.printf("%02X ", b); } System.out.println(); } } } }
Running this program will output all the network interfaces of the machine, including their names, display names, IP addresses, MAC addresses and other information.
2. Sending and receiving network data packets through JNI
When implementing the API network interface, it is often necessary to transmit and interact with data through network data packets. JNI provides us with an interface for sending and receiving network data packets. The following is a simple example of implementing UDP protocol to send and receive network data packets:
import java.net.*; public class UDPClientDemo { public static void main(String[] args) throws Exception { DatagramSocket client = new DatagramSocket(); String message = "Hello, UDP!"; byte[] sendBytes = message.getBytes("UTF-8"); DatagramPacket sendPacket = new DatagramPacket(sendBytes, sendBytes.length, InetAddress.getByName("127.0.0.1"), 8888); client.send(sendPacket); byte[] receiveBytes = new byte[1024]; DatagramPacket receivePacket = new DatagramPacket(receiveBytes, receiveBytes.length); client.receive(receivePacket); String receiveMessage = new String(receivePacket.getData(), 0, receivePacket.getLength(), "UTF-8"); System.out.println("Message from server: " + receiveMessage); client.close(); } }
This sample program first creates a DatagramSocket object, which represents a UDP protocol socket. Then the data packet to be sent and the data packet to be received are specified through the DatagramPacket object. Finally, the send() and receive() methods are used to complete the sending and receiving of data packets respectively, realizing the network data packet transmission operation of the UDP protocol.
Conclusion
Java Network Interface is an important network management API in Java. It can help us manage network interfaces, obtain network information, and send and receive network data packets. In Java back-end development, the management of API network interfaces is an essential link, and JNI is the key to achieving this work. By mastering the use of JNI, we can manage network interfaces and implement API network interface operations more efficiently and conveniently.
The above is the detailed content of Java back-end development: Using Java Network Interface for API network interface management. For more information, please follow other related articles on the PHP Chinese website!
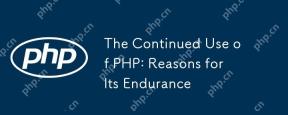
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
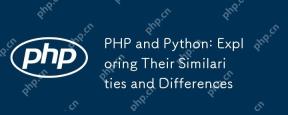
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
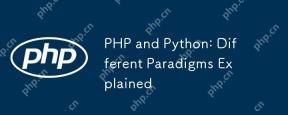
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
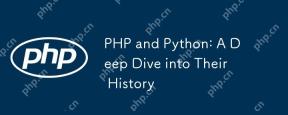
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
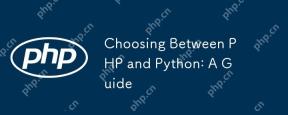
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
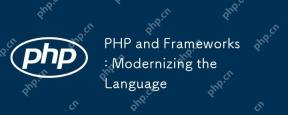
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
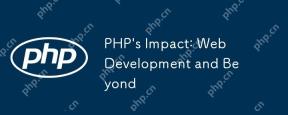
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
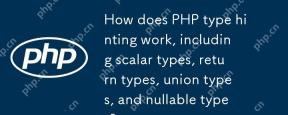
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
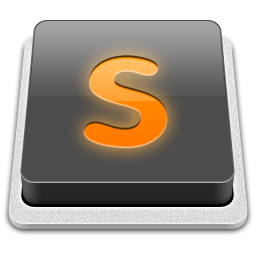
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.