With the continuous development of Internet business, high concurrency and large amounts of data processing have become challenges that Internet applications must face. In order to deal with these challenges, using queues to handle time-consuming tasks has become a common solution in the Laravel framework. This article will introduce how to use Laravel Horizon and IronMQ to manage queues and their tasks.
1. Laravel Horizon
Laravel Horizon is a queue management tool officially provided by Laravel. It provides an easy-to-use and beautiful web interface that can help us view the queue in real time. task status, manage tasks in the queue, monitor the running status of the queue, etc. In addition, Laravel Horizon also provides powerful features such as statistics, automated retries, time window limits, and exception handling for tasks in the queue.
- Install Laravel Horizon
Use Composer to install Laravel Horizon: Execute the following command:
composer require laravel/horizon
- Publishing of Horizon script
After executing the above command, you also need to publish the Laravel Horizon script to the project by executing the following command:
php artisan horizon:install
- Configuring Laravel Horizon
Laravel Horizon The configuration file is located in config/horizon.php. You can configure Horizon's behavior by changing this file. For example, set the connection and name of the queue in the configuration file:
return [ // 要运行的进程数 'processes' => 10, // 队列连接设置 'environments' => [ 'production' => [ 'supervisor-1' => [ 'connection' => 'redis', 'queue' => ['default'], 'balance' => 'simple', 'processes' => 10, ], 'supervisor-2' => [ 'connection' => 'redis', 'queue' => ['high'], 'balance' => 'simple', 'processes' => 5, ], ], ], ]
- Start Laravel Horizon
Use the following command to start Laravel Horizon:
php artisan horizon
Visit http://localhost/horizon and you will see the web interface of Laravel Horizon.
2. IronMQ
IronMQ is a high-performance cloud message queue service that can receive and transmit large amounts of data through API. Compared with other mainstream message queue services, IronMQ is more flexible and reliable, supports multiple languages and operating systems, and has scalable features.
- Register IronMQ
When registering IronMQ, you need to select the language type and data center location you are using. After registering, you will be able to obtain a project ID and a token, which we will use in the Laravel code to connect to your IronMQ queue.
- Install IronMQ
In the Laravel project, use Composer to install IronMQ:
composer require iron-io/iron_mq
- Configure IronMQ
In the project, we can configure IronMQ by adding the following parameters to the .env file:
IRON_PROJECT_ID=IronMQ_project_id IRON_TOKEN=IronMQ_token IRON_QUEUE_NAME=default
- Push tasks to IronMQ
In the Laravel project , push the task to IronMQ in the following way:
IronMQ::queue('queue_name')->post($payload);
where $payload is the content of the task, and queue_name is the name of the queue.
3. Use Laravel Horizon and IronMQ to manage queues and tasks
The following is the general process of using Laravel Horizon and IronMQ to manage queues and tasks:
- In the Laravel project , configure Horizon and IronMQ and start them.
- In Laravel projects, push tasks to IronMQ. Task data can be saved in a database or otherwise generated.
- Laravel Horizon polls IronMQ and consumes tasks from the queue. You can view the status of the queue in Horizon's web interface, including task completion and task retries.
- During task execution, Laravel Horizon provides many useful means to help us improve code quality. For example, in Horizon's web interface, we can view detailed information such as the number of lines of code and time executed by a task, thereby identifying potential performance bottlenecks and code defects in our tasks.
- After task processing, if necessary, the results can be pushed to other systems or fixed data can be returned to the calling end.
Conclusion
This article introduces how to use IronMQ as a queue service in a Laravel application, and combine it with Laravel Horizon to easily manage queues and tasks. By using Laravel Horizon and IronMQ, we can complete asynchronous tasks more efficiently and more easily detect and solve problems that arise during task execution.
The above is the detailed content of Laravel development: How to manage queues using Laravel Horizon and IronMQ?. For more information, please follow other related articles on the PHP Chinese website!
![VMware Horizon Client无法打开[修复]](https://img.php.cn/upload/article/000/887/227/170835607042441.jpg)
VMwareHorizon客户端可帮助您便捷地访问虚拟桌面。然而,有时虚拟桌面基础设施可能会遇到启动问题。本文将讨论当VMwareHorizon客户端未能成功启动时,您可以采取的解决方法。为什么我的VMwareHorizon客户端无法打开?在配置VDI时,如果未打开VMWareHorizon客户端,可能会出现错误。请确认您的IT管理员提供了正确的URL和凭据。如果一切正常,请按照本指南中提到的解决方案解决问题。修复未打开的VMWareHorizon客户端如果您的Windows计算机上未打开VMW
![VMware Horizon客户端在连接时冻结或停滞[修复]](https://img.php.cn/upload/article/000/887/227/170942987315391.jpg)
在使用VMWareHorizon客户端连接到VDI时,我们可能会遇到应用程序在身份验证过程中冻结或连接阻塞的情况。本文将探讨这个问题,并提供解决这种情况的方法。当VMWareHorizon客户端出现冻结或连接问题时,您可以采取一些措施来解决这一问题。修复VMWareHorizon客户端在连接时冻结或卡住如果VMWareHorizon客户端在Windows11/10上冻结或无法连接,请执行下面提到的解决方案:检查网络连接重新启动Horizon客户端检查Horizon服务器状态清除客户端缓存修复Ho
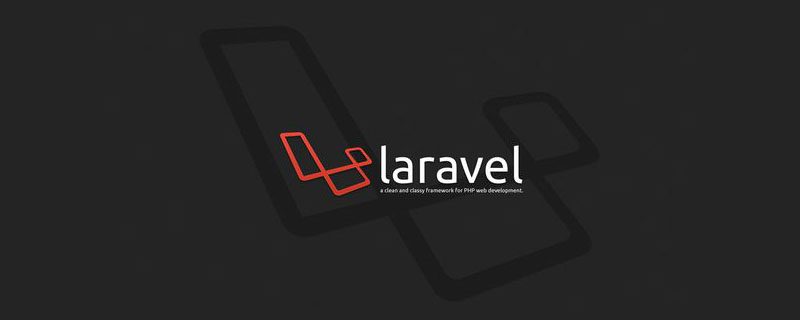
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于单点登录的相关问题,单点登录是指在多个应用系统中,用户只需要登录一次就可以访问所有相互信任的应用系统,下面一起来看一下,希望对大家有帮助。
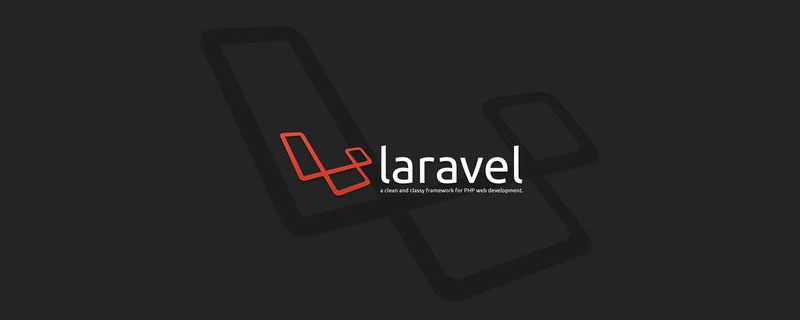
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于Laravel的生命周期相关问题,Laravel 的生命周期从public\index.php开始,从public\index.php结束,希望对大家有帮助。
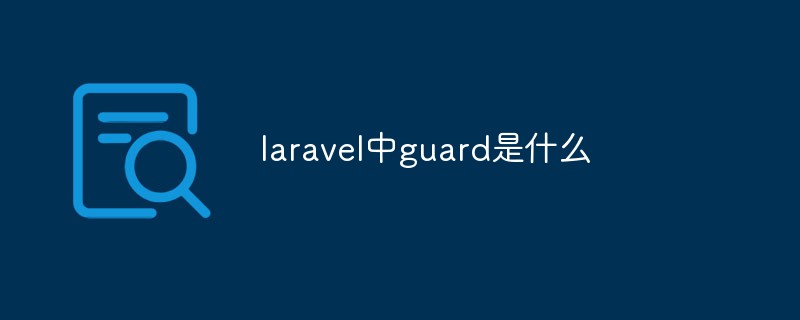
在laravel中,guard是一个用于用户认证的插件;guard的作用就是处理认证判断每一个请求,从数据库中读取数据和用户输入的对比,调用是否登录过或者允许通过的,并且Guard能非常灵活的构建一套自己的认证体系。
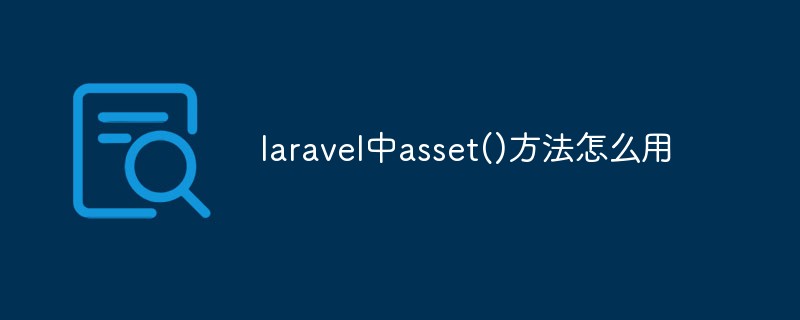
laravel中asset()方法的用法:1、用于引入静态文件,语法为“src="{{asset(‘需要引入的文件路径’)}}"”;2、用于给当前请求的scheme前端资源生成一个url,语法为“$url = asset('前端资源')”。
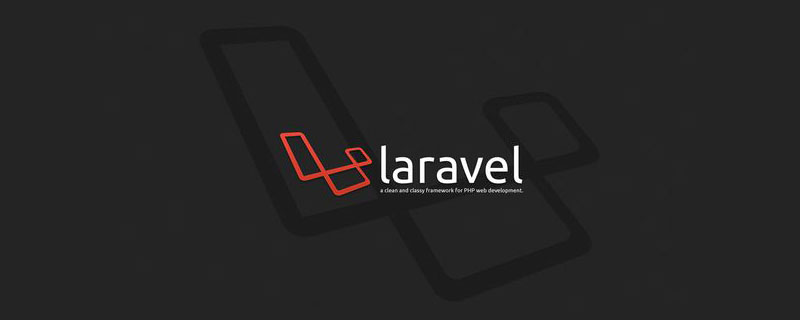
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于使用中间件记录用户请求日志的相关问题,包括了创建中间件、注册中间件、记录用户访问等等内容,下面一起来看一下,希望对大家有帮助。
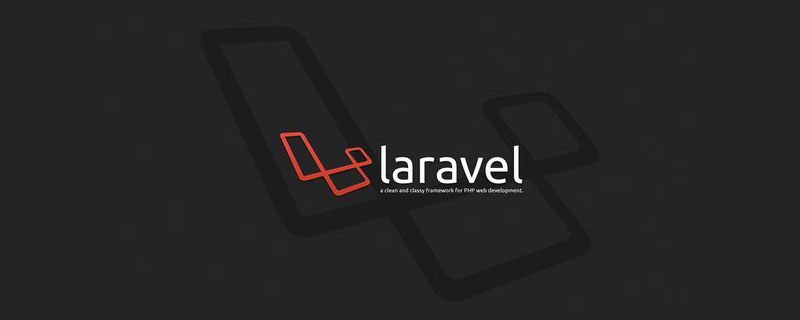
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于中间件的相关问题,包括了什么是中间件、自定义中间件等等,中间件为过滤进入应用的 HTTP 请求提供了一套便利的机制,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
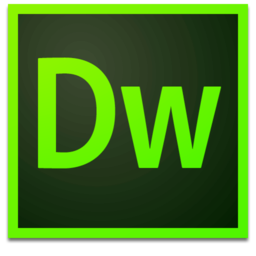
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version
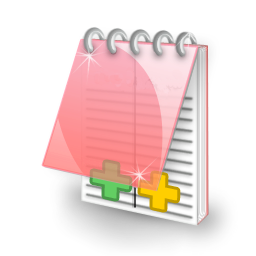
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function