How to perform ACID operations in MySQL database using Go language
With the rapid development of the Internet and digitization, the formation and processing of various big data have become one of the most important things at the moment. In data processing, relational databases play an important role. As one of the most popular relational databases currently, MySQL is often used in big data processing. ACID (Atomicity, Consistency, Isolation, Durability) is the most basic transaction processing feature in relational databases, and it is also the principle that must be followed when performing any operation on data. This article will introduce how to use Go language to perform ACID operations in MySQL database.
1. Install required packages
Before starting to use Go language for MySQL database operations, we need to install some necessary packages. The most common is the mysql driver, which can be installed using the following command
go get github.com/go-sql-driver/mysql
If you are using the Gorm framework for MySQL development, you need to install the following packages
go get -u github.com/jinzhu/gorm go get -u github.com/go-sql-driver/mysql
2. Connect to the database
Before using Go language to perform MySQL database operations, we need to connect to the MySQL database. The following is a sample code to connect to the MySQL database.
import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main(){ db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/database_name") if err != nil { panic(err.Error()) } }
This is the most basic example connection code. Among them, mysql is the name of the driver; root:password is the user name and password; 127.0.0.1:3306 is the server address and port number; database_name is the name of the database to be connected. When using the connection process, you also need to use some other parameters, such as connection timeout, etc. Specific parameters can be changed by consulting the relevant documentation.
3. Transaction operation
Transaction operation refers to a series of operations in the database, which will be applied to the database only after they are all successfully executed. In Go, you can use the Tx of the sql package to perform transaction operations. The following is an example of a basic transaction operation.
import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main(){ db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/database_name") if err != nil { panic(err.Error()) } tx, err := db.Begin() if err != nil { fmt.Println(err) } _, err = tx.Exec("INSERT INTO table_name (column1, column2) VALUES (?, ?)", value1, value2) if err != nil { fmt.Println(err) tx.Rollback() } else { _, err = tx.Exec("UPDATE table_name SET column1 = ? WHERE column2 = ?", value1, value2) if err != nil { fmt.Println(err) tx.Rollback() } else { tx.Commit() } } }
This is a basic transaction operation example. Two operations are performed in this program: the first is to insert data, and the second is to update data. If both operations execute successfully, the transaction is committed. If one of the operations fails, the transaction will be rolled back and all changes will be undone.
4. Isolation level
When performing transaction operations, we need to consider the isolation level. MySQL divides the isolation level into four levels: uncommitted read (read uncommitted), committed read (read committed), repeatable read (repeatable read) and serializable (serializable). The isolation level used by default is Repeatable Read. When we use transactions, we can use the DB.Transaction() method to specify the isolation level. The following is an example:
func main() { db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/database_name") if err != nil { panic(err.Error()) } txOptions := &sql.TxOptions{ Isolation: sql.LevelSerializable, } tx, err := db.BeginTx(context.Background(), txOptions) if err != nil { panic(err.Error()) } }
In this example, we selected the Serializable level isolation level. If you need to modify the isolation level, just change LevelSerializable.
5. Summary
In this article, we introduced how to use Go language to perform ACID operations in the MySQL database, including connecting to the database, transaction operations, and isolation levels. In order to ensure the correctness of the code, we must follow the ACID guidelines to ensure that the data is saved correctly when abnormal conditions occur. At the same time, we must also learn how to use isolation levels to determine the consistency and reliability of the database.
The above is the detailed content of How to perform ACID operations in MySQL database using Go language. For more information, please follow other related articles on the PHP Chinese website!
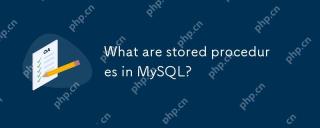
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
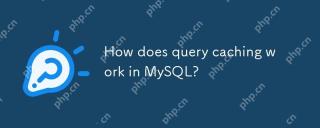
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
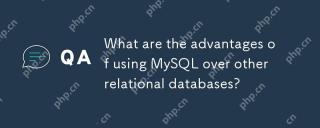
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
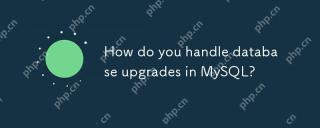
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.
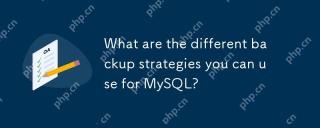
MySQL backup policies include logical backup, physical backup, incremental backup, replication-based backup, and cloud backup. 1. Logical backup uses mysqldump to export database structure and data, which is suitable for small databases and version migrations. 2. Physical backups are fast and comprehensive by copying data files, but require database consistency. 3. Incremental backup uses binary logging to record changes, which is suitable for large databases. 4. Replication-based backup reduces the impact on the production system by backing up from the server. 5. Cloud backups such as AmazonRDS provide automation solutions, but costs and control need to be considered. When selecting a policy, database size, downtime tolerance, recovery time, and recovery point goals should be considered.
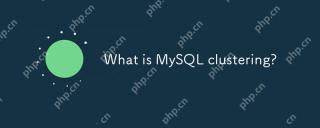
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
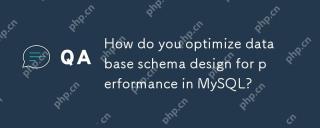
Optimizing database schema design in MySQL can improve performance through the following steps: 1. Index optimization: Create indexes on common query columns, balancing the overhead of query and inserting updates. 2. Table structure optimization: Reduce data redundancy through normalization or anti-normalization and improve access efficiency. 3. Data type selection: Use appropriate data types, such as INT instead of VARCHAR, to reduce storage space. 4. Partitioning and sub-table: For large data volumes, use partitioning and sub-table to disperse data to improve query and maintenance efficiency.
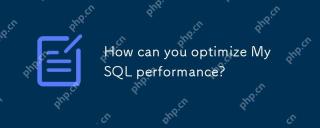
TooptimizeMySQLperformance,followthesesteps:1)Implementproperindexingtospeedupqueries,2)UseEXPLAINtoanalyzeandoptimizequeryperformance,3)Adjustserverconfigurationsettingslikeinnodb_buffer_pool_sizeandmax_connections,4)Usepartitioningforlargetablestoi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
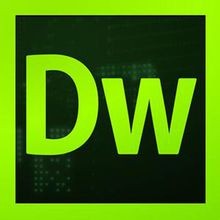
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
