The Go language is increasingly becoming a popular programming language, just like the database MySQL. This article will introduce five common mistakes when using MySQL in Go language so that developers can avoid these mistakes and improve the quality and stability of the code.
Error 1: Not closing the database connection
When using MySQL, it is best to manually close the database connection after use. If the connection is not closed promptly, system resources will be wasted and database performance may decrease. To avoid this happening, developers need to make sure the connection is closed when writing code. Here is a sample code snippet that demonstrates how to properly close a database connection:
// 创建数据库连接 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") // 检查错误 if err != nil { log.Fatal(err) } // 明确关闭数据库连接 defer db.Close()
Note the defer statement in the code. This statement will be executed when the function returns, ensuring that the database connection is closed after use.
Error 2: Unhandled error
When using MySQL, errors may occur at any time, including: database connection failure, query statement error, incorrect parameters, etc. Developers need to ensure that these errors are handled accurately to prevent the program from terminating abnormally. Here is a sample code snippet that demonstrates how to check for errors and handle them:
// 执行SQL查询 rows, err := db.Query("SELECT name FROM users WHERE id = ?", 1) // 检查错误 if err != nil { log.Fatal(err) } // 关闭 rows 对象,注意 defer defer rows.Close() // 遍历结果 for rows.Next() { var name string err := rows.Scan(&name) if err != nil { log.Fatal(err) } fmt.Println(name) }
Note the if statement and log.Fatal() in the code. This statement will interrupt program execution when an error occurs and output an error message.
Error 3: Unable to handle NULL values correctly
In MySQL, NULL is a special value that represents a missing or unknown value. When using MySQL, developers need to determine whether a table field is NULL and handle NULL values correctly. Here is a sample code snippet that demonstrates how to properly handle NULL values:
// 执行SQL查询 rows, err := db.Query("SELECT name, age FROM users WHERE id = ?", 1) // 检查错误 if err != nil { log.Fatal(err) } // 关闭 rows 对象,注意 defer defer rows.Close() // 遍历结果 for rows.Next() { var name string var age sql.NullInt64 err := rows.Scan(&name, &age) if err != nil { log.Fatal(err) } // 处理 NULL 值 if age.Valid { fmt.Println(name, age.Int64) } else { fmt.Println(name, "Age is NULL") } }
Note the sql.NullInt64 type and the if statement in the code. This code will determine whether the age field is NULL when processing NULL values. If so, print "Age is NULL", otherwise print the age value.
Error 4: Using SQL statements to splice strings
Using strings to splice SQL statements is a common mistake. This approach is vulnerable to SQL injection attacks and is not conducive to code maintenance. To avoid this happening, developers can use SQL placeholders and parameters. Here is a sample code snippet that demonstrates how to use placeholders and parameters:
// 定义 SQL 查询和占位符 query := "SELECT name FROM users WHERE age > ? AND gender = ?" // 准备参数 args := []interface{}{18, "M"} // 执行 SQL 查询 rows, err := db.Query(query, args...) if err != nil { log.Fatal(err) } // 关闭 rows 对象,注意 defer defer rows.Close() // 遍历结果 for rows.Next() { var name string err := rows.Scan(&name) if err != nil { log.Fatal(err) } fmt.Println(name) }
Note the placeholders and parameter args in the code. This code uses placeholders when querying, replacing possible SQL injection attacks with parameters.
Error 5: Not using connection pool technology
Connection pool technology is a commonly used database optimization technology, which can reduce the load pressure on the database server and improve system performance. When using MySQL, developers need to use connection pooling technology to manage database connections. The following is a sample code snippet that demonstrates how to use connection pooling technology:
// 创建连接池 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") if err != nil { log.Fatal(err) } db.SetMaxIdleConns(10) db.SetMaxOpenConns(100) // 执行 SQL 查询 rows, err := db.Query("SELECT name FROM users WHERE age > ?", 18) if err != nil { log.Fatal(err) } // 关闭 rows 对象,注意 defer defer rows.Close() // 遍历结果 for rows.Next() { var name string err := rows.Scan(&name) if err != nil { log.Fatal(err) } fmt.Println(name) }
Note SetMaxIdleConns() and SetMaxOpenConns() in the code. This code will use connection pooling technology to manage database connections and limit the maximum number of connections.
Summary
When using MySQL in the Go language, developers need to avoid some common mistakes. These errors include not closing the database connection, not handling errors, failing to handle NULL values correctly, using SQL statements to concatenate strings, and not using connection pooling technology. By avoiding these errors, developers can improve the quality and stability of their code while improving system performance.
The above is the detailed content of Using MySQL in Go: avoid five common mistakes. For more information, please follow other related articles on the PHP Chinese website!
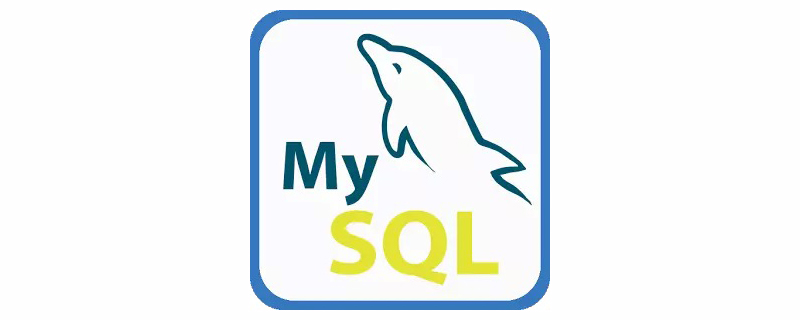
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
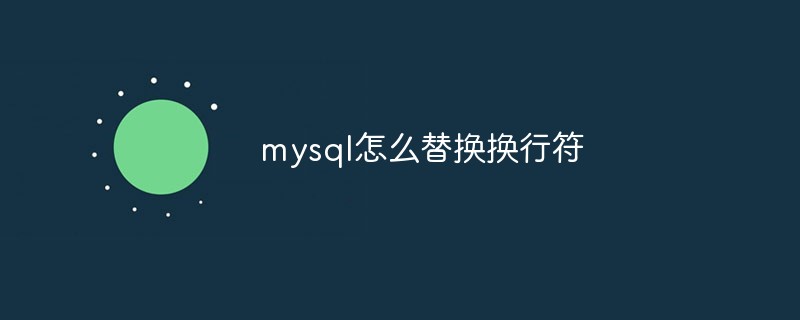
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
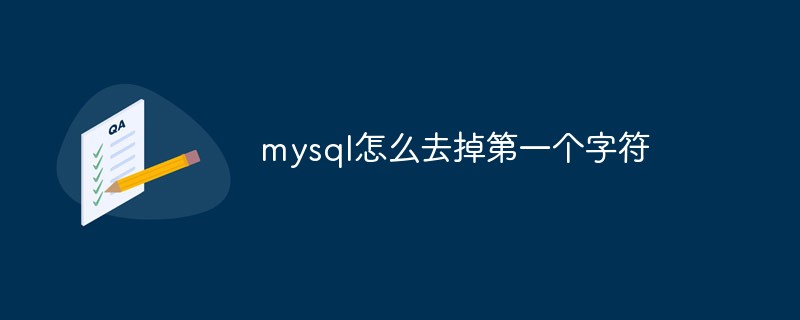
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
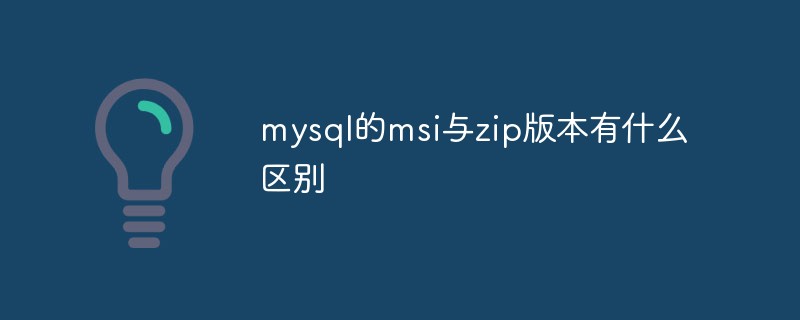
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
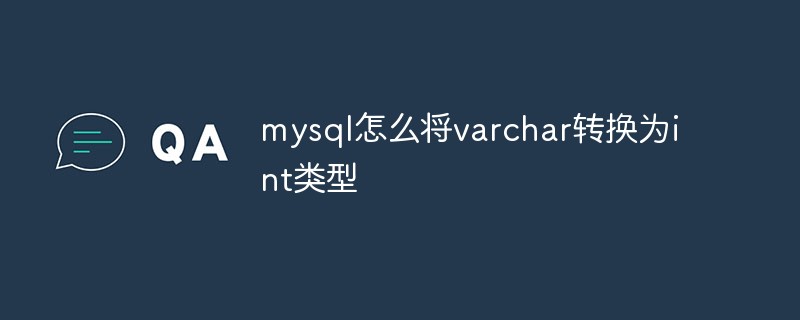
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
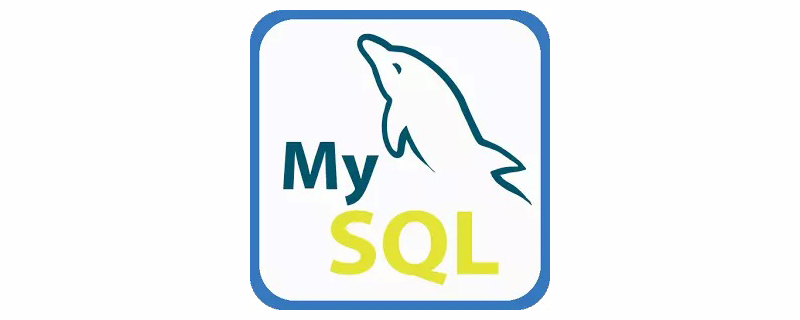
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
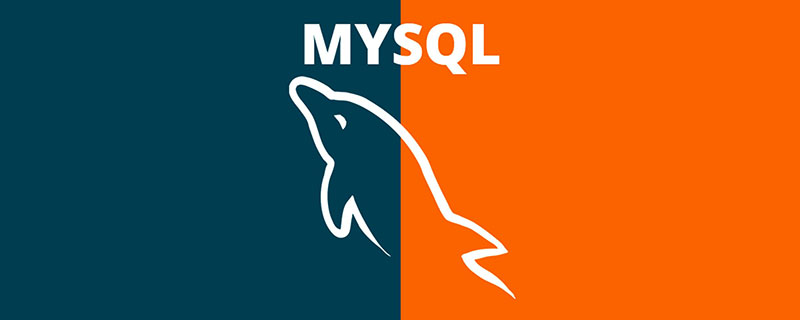
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了mysql高级篇的一些问题,包括了索引是什么、索引底层实现等等问题,下面一起来看一下,希望对大家有帮助。
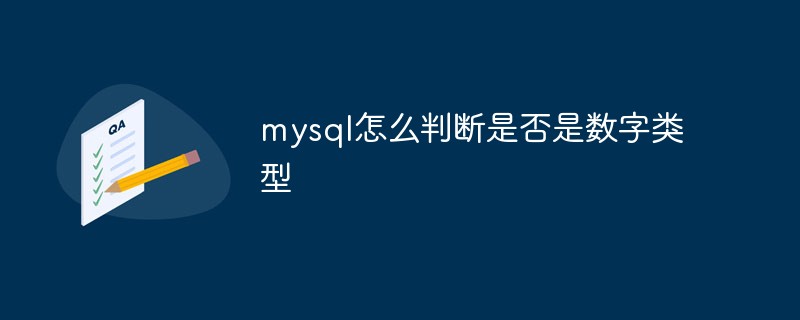
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
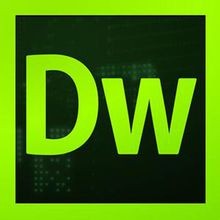
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
