Vue.js is a very popular JavaScript framework that can help us build high-performance, maintainable web applications. In Vue.js, filters are a very useful tool for working with data, especially when working with tabular data. This article will introduce how to use Vue.js filters to process tabular data.
1. Overview of Vue.js filters
Filters are a way of data processing that allow us to convert input data into the required output format. In Vue.js, filters are global functions or Vue instance functions that can be used in templates when data needs to be transformed.
There are two types of Vue.js filters: global filters and local filters. Global filters can be used globally, local filters can only be used within a Vue instance.
2. Use Vue.js filter to process table data
Suppose we have a table containing user data, which includes user name, email address and registration date. We want to format the registration date and sort the table by registration date.
First, define a global filter dateFilter in the Vue instance:
Vue.filter('dateFilter', function(value) { if (!value) return '' return moment(value).format('YYYY/MM/DD') })
In the above code, we use the moment.js library to convert the date string into a date object and use format () method formats it into 'YYYY/MM/DD' format.
Next, use the filter in the template of the table:
<table> <thead> <tr> <th>用户名</th> <th>邮箱</th> <th @click="sortTable('registerDate')">注册日期</th> </tr> </thead> <tbody> <tr v-for="user in sortedUsers"> <td>{{ user.name }}</td> <td>{{ user.email }}</td> <td>{{ user.registerDate | dateFilter }}</td> </tr> </tbody> </table>
In the above code, we use the pipe character '|' to pass the user's registerDate field to the dateFilter filter. Vue will automatically call the dateFilter function, passing registerDate as a parameter.
Note that when using filters in templates, the processing order of filters may affect the results. In the above code, we first sort the table by registration date (sortedUsers) and then format the date.
Next, we need to implement the sortTable() method. This method sorts the table based on the clicked header title.
sortTable(column) { if (this.sortColumn === column) { this.sortOrder = this.sortOrder * -1 } else { this.sortColumn = column this.sortOrder = 1 } this.users.sort((a, b) => { let x = a[column] let y = b[column] if (column === 'registerDate') { x = moment(x) y = moment(y) } if (x < y) { return -1 * this.sortOrder } else if (x > y) { return 1 * this.sortOrder } else { return 0 } }) }
In the above code, we use the sort() method to sort the table. If the column name is 'registerDate', we convert the date string to a date object and sort by the date object.
Finally, we need to define users data in the Vue instance and load user data in the created() function.
data: { users: [], sortColumn: '', sortOrder: 1 }, created() { axios.get('/api/users').then(response => { this.users = response.data }) }
In the above code, we are using Axios to load user data from the backend server.
3. Summary
Vue.js filter is a very useful tool that can help us process various types of data. In this article, we covered how to use Vue.js filters to process tabular data. We define a global filter that converts the date string into a specified format and use this filter to convert the user's registration date data. In addition, we also introduced how to implement the sorting function of the table. Through studying this article, I believe you have understood the basic usage of Vue.js filters, and I hope it will be helpful to your future development work.
The above is the detailed content of VUE3 basic tutorial: Using Vue.js filters to process table data. For more information, please follow other related articles on the PHP Chinese website!
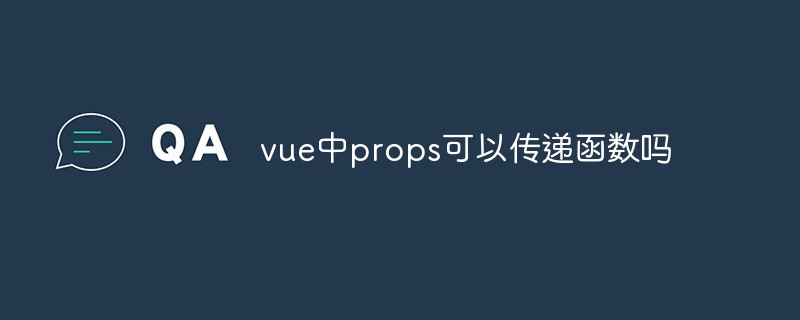
vue中props可以传递函数;vue中可以将字符串、数组、数字和对象作为props传递,props主要用于组件的传值,目的为了接收外面传过来的数据,语法为“export default {methods: {myFunction() {// ...}}};”。
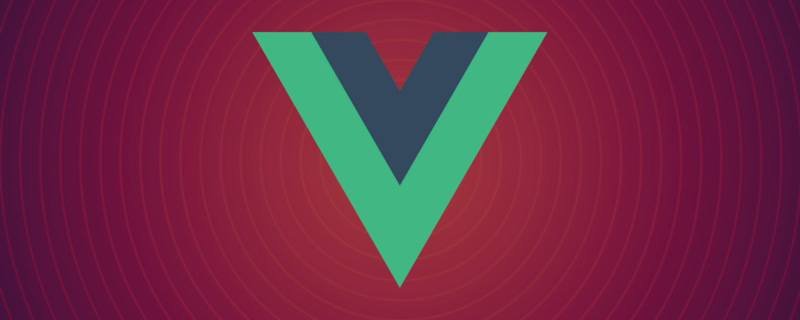
本篇文章带大家聊聊vue指令中的修饰符,对比一下vue中的指令修饰符和dom事件中的event对象,介绍一下常用的事件修饰符,希望对大家有所帮助!
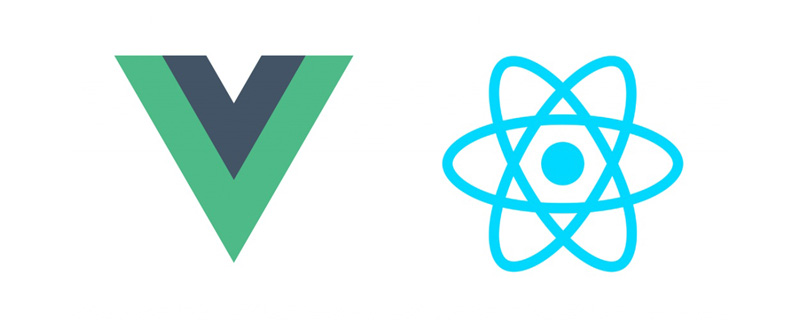
如何覆盖组件库样式?下面本篇文章给大家介绍一下React和Vue项目中优雅地覆盖组件库样式的方法,希望对大家有所帮助!
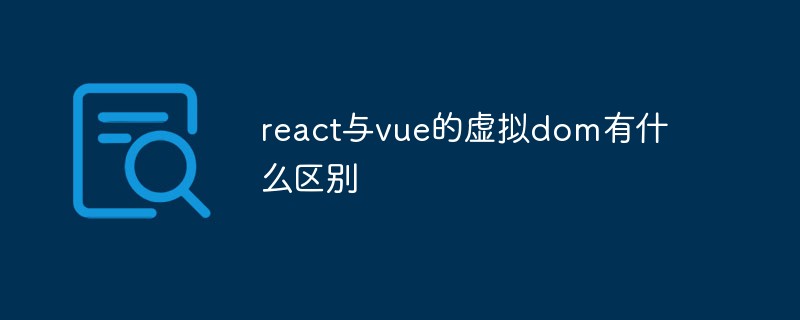
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
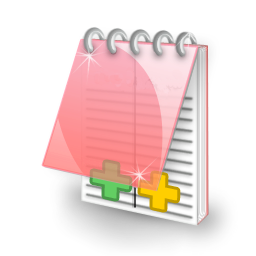
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
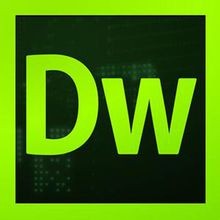
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
