Practical tips for string processing in Java
In Java programming, string processing is a very common operation. String processing can be said to be one of the essential skills for Java programmers. This article will share some practical tips for string processing in Java to help you process strings more efficiently.
- String concatenation
String concatenation is one of the most common operations in Java programming. Java provides multiple ways to implement string splicing:
Method 1: Use the " " symbol to implement string splicing
String str = "Hello, " "world!";
Method 2: Use the concat() method of the String class to implement string splicing
String str1 = "Hello";
String str2 = ", world!";
String result = str1 .concat(str2);
Method 3: Use the append() method of the StringBuilder class to implement string splicing (recommended)
StringBuilder sb = new StringBuilder();
sb.append ("Hello, ");
sb.append("world!");
String result = sb.toString();
- String interception
In Java, you can use the substring() method to implement string interception. This method requires two parameters to be passed in. The first parameter specifies the starting position of the interception, and the second parameter specifies the end position of the interception (excluding the characters at this position).
String str = "Java programming";
String result = str.substring(5, 16);
The above code will intercept "pro" from the string "Java programming" "String.
- String replacement
In Java programming, you can use the replace() method to implement string replacement. This method needs to pass in two parameters, the first parameter is the string to be replaced, and the second parameter is the replaced string.
String str = "Java is a programming language";
String result = str.replace("Java", "Python");
The above code will replace the "Java" is replaced with "Python" and the end result is "Python is a programming language".
- String splitting
In Java, you can use the split() method to achieve string splitting. This method needs to pass in a parameter, which is the separator, and uses the separator to split the string into an array.
String str = "Java,is,a,programming,language";
String[] strArray = str.split(",");
The above code will string " Java,is,a,programming,language" separated by commas, resulting in a string array containing 5 elements.
- Convert string to uppercase/lowercase
In Java, you can use the toUpperCase() method to convert a string to uppercase, and use the toLowerCase() method to convert Strings are converted to lowercase.
String str = "Hello, World!";
String upper = str.toUpperCase(); // Convert to uppercase
String lower = str.toLowerCase(); // Convert to lowercase
- String to remove spaces
In Java, you can use the trim() method to remove leading and trailing spaces from a string.
String str = "Hello, World! ";
String result = str.trim();
The above code removes the spaces before and after the string, and the final result is "Hello, World!" string.
Summary
The above are some practical tips for string processing in Java. I hope they can help you process strings better. In the actual programming process, different methods should be selected to implement string processing according to specific needs. At the same time, it is important to note that strings in Java are immutable, so it is important to note that a new string object is created every time a string is processed. If you need to frequently modify strings, you can use the StringBuilder class, which can modify the original string to avoid creating new string objects multiple times and improve efficiency.
The above is the detailed content of Practical Tips for String Processing in Java. For more information, please follow other related articles on the PHP Chinese website!
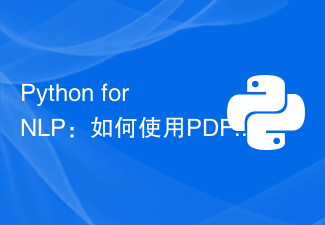
PythonforNLP:如何使用PDFMiner库处理PDF文件中的文本?导语:PDF(PortableDocumentFormat)是一种用于存储文档的格式,通常用于共享和分发电子文档。在自然语言处理(NLP)领域,我们经常需要从PDF文件中提取文本,以进行文本分析和处理。Python提供了许多用于处理PDF文件的库,其中PDFMiner是一个强
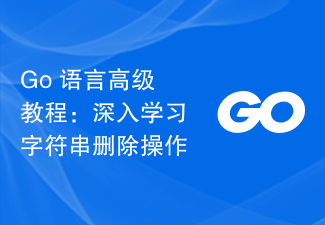
Go语言是一种非常流行的编程语言,其强大的特性使其受到众多开发者的青睐。字符串操作是编程中最常见的操作之一,而在Go语言中,对于字符串的删除操作也是非常常见的。本文将深入探讨Go语言中的字符串删除操作,通过具体的代码示例来帮助你更好地理解和掌握这一知识点。字符串删除操作在Go语言中,我们通常使用strings包来进行字符串操作,包括删除操作
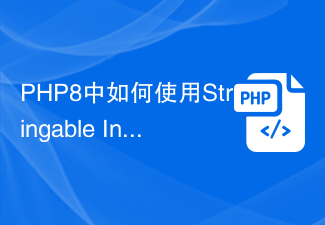
PHP8中如何使用StringableInterface更方便地处理字符串操作?PHP8是PHP语言的最新版本,带来了许多新特性和改进。其中一项令开发者欢欣鼓舞的改进之一就是StringableInterface的加入。StringableInterface是一个用于处理字符串操作的接口,它提供了一种更方便的方式来处理和操作字符串。本文将详细介绍如何使
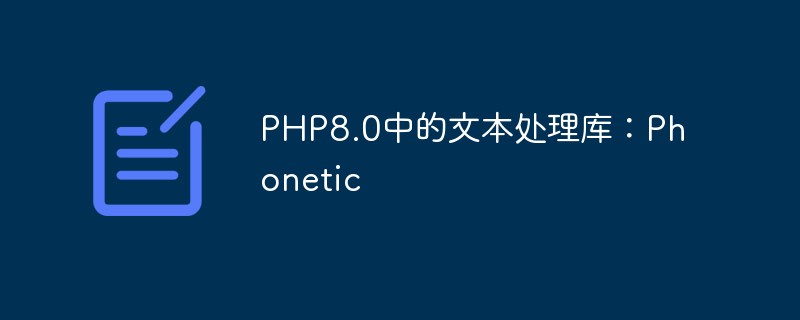
随着PHP8.0的发布,很多人都在关注它的新特性。其中一个备受瞩目的特性是它的文本处理库,Phonetic。这个库提供了一些有用的方法,如音标转换、拼音转换和近似字符串匹配。在本文中,我们将深入探讨这个库的功能和用法。什么是Phonetic?Phonetic是一个用于处理文本的库,它提供了几个方法,使得文本的处理更加方便和准确。该库集成了三个主要的功能:音
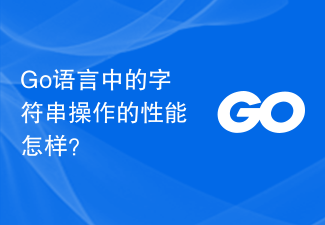
Go语言中的字符串操作的性能怎样?在程序开发中,字符串的处理是不可避免的,尤其在Web开发中,字符串的处理更是经常出现。因此,字符串操作的性能显然是开发者十分关心的问题。那么,在Go语言中,字符串操作的性能如何呢?本文将从以下几个方面来探讨Go语言中字符串操作的性能。基本操作Go语言中的字符串是不可变的,即一旦创建,就无法对其中的字符进行修改
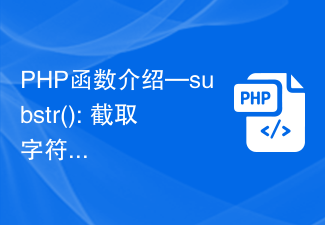
PHP函数介绍—substr():截取字符串的一部分在PHP中,字符串处理是非常常见的操作。而对于一个字符串来说,有时我们需要从中截取出一部分内容进行处理。这时候,PHP提供了一个非常实用的函数——substr()。substr()函数可以截取一个字符串的一部分,具体的使用方式如下:stringsubstr(string$string,int
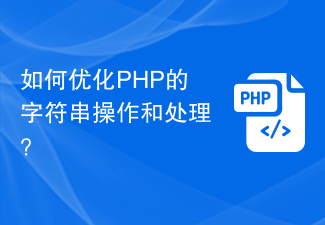
如何优化PHP的字符串操作和处理?在web开发中,字符串操作和处理是非常常见和重要的部分。对于PHP来说,字符串的优化可以提高程序的性能和响应速度。本文将介绍一些优化PHP字符串操作和处理的方法。避免使用不必要的字符串连接操作字符串连接操作(使用"."操作符)会导致性能低下,特别是在循环中。例如,以下代码:$str="";for($
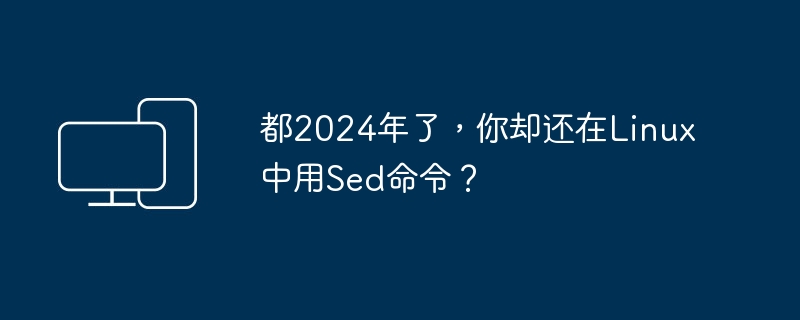
概述在Linux系统中,文本处理是日常任务的关键部分。无论是编辑配置文件、分析日志文件,还是处理数据,文本处理工具都至关重要。尽管sed命令在Linux中被广泛使用,但其语法复杂,学习难度较大。sd命令则是一个简单直观的文本替换工具,旨在提供一个比sed更易用的替代品。本文将详细介绍sd命令的概念、功能和用法。sd命令是一个用于文本处理的命令行工具,提供友好的用户界面和丰富功能,使用户能轻松进行文本操作,如替换、删除、插入行等。其设计目标在于简化文本处理过程,使其更直观易懂。通过sd命令,用户可


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
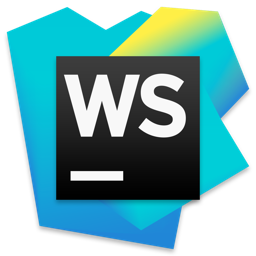
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
